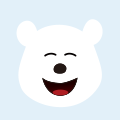
如何写精华回答,获更多曝光?
发布
点赞和取消点赞不刷新,
HealthCircleInfo
是一个多层嵌套模型。用Text模拟点赞,UI不刷新
import { PullToRefresh } from '@ohos/pulltorefresh'
import { LazyDataSource } from '../utils/LazyDataSource';
import { MessageItemList, StringData } from 'authorizedcontrol/src/main/ets/model/HealthCircleModel';
import { AppUtil, DateUtil, DeviceUtil, ObjectUtil, PreferencesUtil } from '@pura/harmony-utils';
import { apiHealthCircleData } from '../axios/ApiParam';
import Constants from 'authorizedcontrol/src/main/ets/utils/Constants';
import { AxiosError, AxiosResponse } from '@ohos/axios';
import { HealthCircleInfo } from 'authorizedcontrol/src/main/ets/model/HealthCircleModel';
@Entry
@Component
struct Index {
private scroller: Scroller = new Scroller();
private getResourceString(res: Resource) {
return getContext().resourceManager.getStringSync(res.id)
}
// 用状态变量来驱动UI刷新,而不是通过Lazyforeach的api来驱动UI刷新
@State data: LazyDataSource<HealthCircleInfo> = new LazyDataSource();
@State mCircleList: Array<HealthCircleInfo> = []
async apiHealthCircleData() {
let login_token = await PreferencesUtil.getString("login_token");
let user_id = await PreferencesUtil.getString("user_id");
apiHealthCircleData({
token: login_token,
os_type: Constants.os_type,
appver: AppUtil.getVersionName(),
deviceid: DeviceUtil.getDeviceId(false, ""),
os_ver: DeviceUtil.getBuildVersion() + '',
tsktime: DateUtil.getTodayTime() + '',
tab_id: '1',
msg_id: '0',
})
.then((res: AxiosResponse) => {
if (res.data.code == 0) {
if (!ObjectUtil.isEmpty(res.data.obj.messages)) {
this.mCircleList = []
this.mCircleList = res.data.obj.messages
for (let index = 0; index < this.mCircleList.length; index++) {
this.data.pushData(this.mCircleList[index]);
}
}
}
}).catch((err: AxiosError) => {
console.log('error', JSON.stringify(err.message))
})
}
aboutToAppear() {
this.apiHealthCircleData()
}
build() {
Column() {
PullToRefresh({
// 必传项,列表组件所绑定的数据
data: $data,
// 必传项,需绑定传入主体布局内的列表或宫格组件
scroller: this.scroller,
// 必传项,自定义主体布局,内部有列表或宫格组件
customList: () => {
// 一个用@Builder修饰过的UI方法
this.getListView();
},
// 可选项,下拉刷新回调
onRefresh: () => {
return new Promise<string>((resolve, reject) => {
// 模拟网络请求操作,请求网络2秒后得到数据,通知组件,变更列表数据
setTimeout(() => {
resolve('陈瑶');
// this.data = [...this.dataNumbers];
}, 2000);
});
},
// 可选项,上拉加载更多回调
onLoadMore: () => {
return new Promise<string>((resolve, reject) => {
// 模拟网络请求操作,请求网络2秒后得到数据,通知组件,变更列表数据
setTimeout(() => {
resolve('');
// this.data.push(`${'陈瑶真美'} ${this.data.length}`);
}, 2000);
});
},
customLoad: null,
customRefresh: null,
})
}
}
@Builder
private getListView() {
List({ space: 20, scroller: this.scroller }) {
LazyForEach(this.data, (item: HealthCircleInfo, index: number) => {
ListItem() {
ChildComponent({ data: item })
}
}, (item: HealthCircleInfo, index: number) => index.toString())
}
.backgroundColor('#eeeeee')
.divider({ strokeWidth: 1, color: 0x222222 })
.edgeEffect(EdgeEffect.None) // 必须设置列表为滑动到边缘无效果
}
}
@Component
struct ChildComponent {
@ObjectLink data: HealthCircleInfo
build() {
Column() {
Text(this.data.realname).fontSize(50)
.onAppear(() => {
// console.info("appear:" + this.data.message)
}).onClick(() => {
this.data.realname += '陈瑶';
})
Image($r('app.media.ai_chenyao'))
.width(500)
.height(200)
}.margin({ left: 10, right: 10 })
}
}
下面是我请求接口之后的model,多层嵌套
import { image } from "@kit.ImageKit";
import { url } from "@kit.ArkTS";
// @Observed类装饰器 和 @ObjectLink 用于在涉及嵌套对象或数组的场景中进行双向数据同步
@Observed
export class StringData {
realname: string;
img: Array<MessageItemList> = []
constructor(realname: string) {
this.realname = realname
}
//
// constructor(realname: string, imgList: Array<MessageItemList>) {
// this.realname = realname
// this.img = imgList
// }
}
// @Observed类装饰器 和 @ObjectLink 用于在涉及嵌套对象或数组的场景中进行双向数据同步
@Observed
export class HealthCircleInfo {
// 首页列表图片
photo: string = '';
realname: string = '';
userid: string = '';
id: string = '';
msg_id: string = '';
create_time: string = '';
update_time?: string;
status?: string;
topic_name: string = '';
open_type1_cnt: string = ''; //评论数
open_type2_cnt: string = ''; //点赞数
content: MessageInfo = new MessageInfo();
comment: Array<CommentInfo> = []
isEmptyComment: boolean = true
praiseAllName?: string //点赞名字集合
isPraiseOrNo: boolean = false //是否点赞过
}
export class MessageInfo {
type?: string
message: MessageItemInfo = new MessageItemInfo();
}
export class CommentInfo {
id: string = ''
msg_id: string = ''
comment_userid: string = ''
reply_userid?: string
type?: string //1 评论 2点赞
comment_user_realname: string = ''
reply_user_realname?: string
content?: string //评论内容
}
export class MessageItemInfo {
msg?: string;
img: Array<MessageItemList> = []
}
export class MessageItemList {
url: string = '';
thumb?: PixelMap;
sz?: string
}
export class NewsItemList {
icon: PixelMap;
title: string;
url: string;
read_total: string;
constructor(icon: PixelMap, title: string, url: string, read_total: string) {
this.icon = icon;
this.title = title
this.url = url;
this.read_total = read_total
}
}
export class ServiceCenterInfo {
title: string;
area_address: string;
phone: string;
img: string;
constructor(title: string, area_address: string, phone: string, img: string,) {
this.title = title
this.area_address = area_address;
this.phone = phone
this.img = img;
}
}
export class HomeToolsListItem {
scene_type: string
scene_info: Scene_Info;
constructor(scene_type: string, scene_info: Scene_Info) {
this.scene_type = scene_type;
this.scene_info = scene_info
}
}
export class Scene_Info {
url: string;
title: string;
icon: string;
show_num: string;
constructor(url: string, title: string, icon: string, show_num: string,) {
this.url = url;
this.title = title;
this.icon = icon;
this.show_num = show_num;
}
}
export class DiseaseData {
value: number;
time: string;
desc: number;
constructor(value: number, time: string, desc: number) {
this.value = value;
this.time = time;
this.desc = desc
}
}
export class DiseaseDataTitle extends DiseaseData {
title: string;
constructor(value: number, time: string, title: string, desc: number) {
super(value, time, desc);
this.title = title;
}
}
结果,
this.data.realname += '陈瑶';
这行代码没有效果,UI不刷新