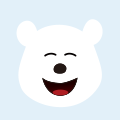
Java常用核心类(详细讲解) 原创
春节不停更,此文正在参加「星光计划-春节更帖活动」
Java常用核心类有:
Object:终极父类
Math类
基本类型包装类
日期/时间API
本期内容详细讲解了每个类的定义以及具体的使用
超详细!!!
Object: 终极父类
Java语言中有一个名为java.lang.Object的特殊类,所有的类都是直接或间接地继承该类而得到的。
定义类时,若没有用extends指明继承哪个类,编译器会自动加上extends Object。
Object 类中定义的方法:
public String toString( ) //返回对象的字符串表示
public boolean equals( Object obj) //比较对象是否与obj相等
public class<?> getClass() //返回对象所属的类所对应的Class对象
public int hashCode() //返回对象的哈希码值
protected Object clone() //创建并返回对象的一个副本
protected void finalize() //当对该对象没有引用时由垃圾回收器调用
toString( )方法
- 调用对象的toString()方法可以返回对象的字符串表示。
- 如果在Employee类中没有覆盖toString()方法,执行以下代码:
- 可能产生类似下面的输出:
com.demo.Employee@1db9742 //类完全限定名+@+16进制数据
- 在Employee类中覆盖 toString()方法
- System.out.println(emp.toString());
- System.out.println(emp); //自动调用toString()方法
输出结果都为:
员工信息:李浪 19 5000.0
equals( )方法
- equals()方法主要用来比较两个对象是否相等,使用格式为:
obj1.equals(obj2) - equals()方法在Object类中的定义:
public boolean equals(Object obj){
return (this == obj); //比较引用是否相等
} - 相当于两个对象使用“==”号进行比较。
getClass( )方法
- 返回运行时的对象所属的类所对应的Class对象。
- 每当一个类被加载时,JVM就会自动为其生成一个Class对象。由于Class类没有构造方法,需要通过Object类的getClass()方法取得对象对应的Class对象。
- getClass().getName()是用来返回Class对象所代表的具体对象的名称。
hashCode( )方法
- hashCode()方法返回对象的哈希码(hash code)值,即对象在内存中的十进制地址。
- 在覆盖Object类的hashCode()方法时,要保证相同对象的哈希码必须相同。
- 覆盖时常调用java.util.Objects类的hash()方法
可以使用不同算法生成对象的哈希码,例如,String类使用下面算法生成它的哈希码:
clone( )方法
- 使用Object类的clone()方法可以克隆一个对象,即创建一个对象的副本。
- 要使类的对象能够克隆,类必须实现Cloneable接口。
- clone()方法声明抛出CloneNotSupportedException异常。
- clone()方法的返回类型为Object
finalize( )方法
- 在对象被销毁之前,垃圾回收器允许对象调用finalize( )方法进行清理工作,称为对象终结。
- finalize( )方法的定义格式为:
protected void finalize( ) throws Throwable - 每个对象的finalize( )方法仅被调用一次。
Object类中toString()、equals(Object obj)、hashCode()、getClass()、clone()方法使用
Math类
- java.lang.Math类中定义了一些方法实现数学上的基本函数功能:
指数函数
对数函数
平方根函数
三角函数
两个常量PI和E
- Math类中定义的所有的方法和两个常量都是static的,仅能通过类名访问。
方法声明 | 功能描述 |
---|---|
public static double abs (double a) | 返回a的绝对值 |
public static double max (double a,double b) | 返回a、b的较大值 |
public static double min (double a,double b) | 返回a、b的较小值 |
public static double pow (double a,double b) | 返回a的b次幂 |
public static double sqrt (double a) | 返回a的平方根 |
public static double log (double a) | 返回a的对数在 |
public static double sin (double a) | 返回a的正弦值 |
public static double asin (double a) | 返回a的反正弦值 |
public static double random() | 产生一个[0.0,1.0)之间的随机数 |
public static double ceil (double a) | 向上取整 |
public static double floor(double a) | 向下取整 |
Math类的使用
- Math类中的random()方法用来生成大于等于0.0小于1.0的double型随机数
(0.0<=Math.random()<1.0)
(int)(Math.random() * 10) // [0,9]
50 + (int)(Math.random() * 51) // [50, 100]
a + (int)(Math.random() * (b+1)) // [a, a +b]
random()方法的使用
问题描述
- 编写一个方法,随机返回一个小写字母。用该方法随机生成100个小写字母输出,每行20个。
public static char getLetter() - 思路:小写字母的ASCII码值在97(‘a’)到122(‘z’)之间,因此只需随机产生97到122之间的整数,然后把它们转换成字符即可。
基本类型包装类
- Java提供8个基本数据类型包装类(wrapper class),通过这些类,可以将基本类型的数据包装成对象。
注:以上包装类在java.lang包中
每种包装类型都覆盖了toString( )方法和equals( )方法。
- toString( )方法将包装类型的对象中的值以字符串形式返回。
Boolean b = new Boolean(false);
System.out.println(b); - equal( )方法比较包装类型的对象时是比较所包装的值
Character类
Character类对象封装了单个字符值。
- 构造方法:
public Character(char value)。
- 常用方法:
public char charValue() : 返回Character对象所包含的char值。
public int compareTo(Character anotherCharacter):比较两个字符对象,相等返回0,小于参数返回负值,大于参数返回正值。
public static boolean isDigit(char ch): 返回参数字符是否是数字。
public static boolean isLetter (char ch): 返回参数字符是否是字母。
public static char toLowerCase(char ch): 将参数字符转换为小写字母返回。
public static char toUpperCase(char ch): 将参数字符转换为大写字母返回
Boolean类
Boolean类封装了一个布尔值(true或false)。
- 构造方法:
public Boolean(boolean value) :用一个boolean型值创建Boolean对象。
public Boolean(String s) :用一个字符串创建对象。若s不为null且其值为”true”(不区分大小写)则封装的布尔值为true,否则封装的值为false。
- 常用方法:
public boolean booleanValue():返回该对象封装的boolean值。
public static boolean parseBoolean(String s):将s解析为一个boolean值,s不为null且等于”true”(不区分大小写)时解析为true。
public static Boolean valueOf(boolean b):将b的值转化为Boolean对象。
public static Boolean valueOf(String s):将s的值转化为Boolean对象。
数值型包装类
- 6种数值型的包装类:
Byte/Short/Integer/Long/Float/Double
- 都有两个构造方法:
以该类型的基本数据类型作为参数;
以一个字符串作为参数。
Integer类
Integer类封装了一个int型数据。
- 构造方法:
public Integer(int value) :使用int类型的值创建Integer对象。
public Integer(String s) :使用字符串构造Integer对象,若字符串不能转换成相应的数值,则抛出NumberFormatException。
- 常用方法:
public static String toBinaryString(int i): 返回i用字符串表示的2进制序列。
public static String toHexString(int i) : 返回i用字符串表示的16进制序列。
public static String toOctalString(int i): 返回i用字符串表示的8进制序列。
public static int signum(int i): 返回i的符号,若i>0返回1,若i=0返回0,若i<0返回-1。
public static int parseInt(String s) :将s转化为int类型值。
数值型包装类的常量
- 每个数值包装类都定义了以下常量:
SIZE:对应基本类型数据所占比特数
BYTES:对应基本类型数据所占字节数
MAX_VALUE:对应基本类型数据的最大值
MIN_VALUE:对应基本类型数据的最小值(对于浮点型是最小正值)
- Float和Double还增加了以下常量:
POSITIVE_INFINITY :正无穷大
NEGATIVE_INFINITY :负无穷大
NaN :非数值
自动装箱与自动拆箱
Java 5版本提供了自动装箱与自动拆箱功能。
- 自动装箱(autoboxing)是指基本类型的数据可以自动转换为包装类的实例。
- 自动拆箱(unboxing)是指包装类的实例自动转换为基本类型的数据。
例子:
Interger value = 308; //自动装箱
int x = value; //自动拆箱
BigInteger和BigDecimal类
- 表示非常大的整数或非常高精度的浮点数。
在java.math包中定义。 - 扩展了Number类并实现了Comparable接口,它们的实例都是不可变的。
- 创建实例,可以使用
new BigInteger(String) //大整数对象
new BigDecimal(String) //高精度浮点数对象
BigInteger类的应用
问题描述
- 编写一个方法,求任意整数的阶乘。编程求50的阶乘。
public static BigInteger factor( long n) - 思路:可通过循环计算1 * 2 * …* n,结果用BigInteger类型,乘法用multiply方法。
BigDecimal类的应用
BigDecimal类的divide( )方法
日期/时间API
- Java 8开始提供了一个新的日期-时间API,它们定义在java.time包中。
- 常用的类包括LocalDate、LocalTime、LocalDateTime、YearMonth、MonthDay、Year、Instant、Duration及Period等类。
本地日期类LocalDate
- LocalDate对象用来表示带年月日的本地日期,它不带时区和时间信息。
- 使用下列方法创建LocalDate对象。
static LocalDate now()
static LocalDate of(int year, int month, int dayOfMonth) - LocalDate类的常用方法:
plusDays, plusWeeks, plusMonths, plusYears
minusDays, minusWeeks, minusMonths, minusYears
getDayOfWeek, getDayOfMonth, getDayOfYear
getMonthValue
getYear
lengthOfMonth, lengthOfYear
isLeapYear
本地时间类LocalTime
- LocalTime对象表示本地时间,包含时、分和秒,它是不可变对象。时间对象中不包含日期和时区
- 使用下列方法创建LocalTime对象。
static LocalTime now()
static LocalTime of(int hour, int minute, int second)
LocalDate和LocalTime应用
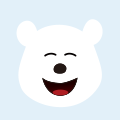