1.网络请求结果如下:
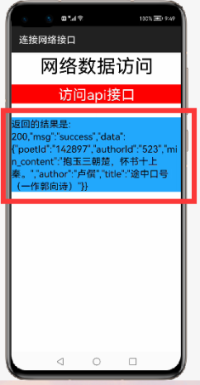

2.新建项目,在ability_main.xml布局如下:
3.在配置文件config.json中添加访问网络的请求:
4.在这里封装一下网络请求的工具类,方便工程调用和复用,工具类封装如下:
public class HttpRequestUtil {
/**
* 有前三个参数即可访问成功,设置更多参数以便更通用
* @param context 上下文
* @param urlString 请求的接口路径
* @param requestMethod 请求方式:GET POST DELETE PUT
* @param token 访问受限资源携带token,非受限资源传递null即可
* @param data 当请求方式为PUT/POST时,通过请求体传递数据(JSON格式的字符串)
* @return 返回请求结果(字符串类型)
*/
private static String sendRequest(Context context,String urlString,String requestMethod,String token,String data){
String result = null;
//创建链接
NetManager netManager = NetManager.getInstance(context);
if (!netManager.hasDefaultNet()) {
return null;
}
NetHandle netHandle = netManager.getDefaultNet();
// 可以获取网络状态的变化
NetStatusCallback callback = new NetStatusCallback() {
// 重写需要获取的网络状态变化的override函数
};
netManager.addDefaultNetStatusCallback(callback);
// 通过openConnection来获取URLConnection
HttpURLConnection connection = null;
try {
//发送链接
URL url = new URL(urlString);
connection = (HttpURLConnection) netHandle.openConnection(url, Proxy.NO_PROXY);
//设置请求方式
connection.setRequestMethod(requestMethod);
if (data != null){
//允许通过此网络连接向服务器端写数据
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type","application/json;charset=utf-8");
}
//如果参数token!=null,则需要将token设置到请求头
if (token != null){
connection.setRequestProperty("token",token);
}
//发送请求建立连接
connection.connect();
//向服务器传递data中的数据
if (data != null){
byte[] bytes = data.getBytes("UTF-8");
OutputStream os = connection.getOutputStream();
os.write(bytes);
os.flush();
}
//获取链接结果
//从连接中获取输入流,读取api接口返回的数据
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK){
InputStream is = connection.getInputStream();
StringBuilder builder = new StringBuilder();
byte[] bs = new byte[1024];
int len = -1;
while ((len = is.read(bs)) != -1){
builder.append(new String(bs,8,len));
}
result = builder.toString();
}
} catch(IOException e) {
e.printStackTrace();
} finally {
if (connection != null){
connection.disconnect();
}
}
return result;
}
//get请求
public static String sendGetRequest(Context context,String urlString){
return sendRequest(context,urlString,"GET",null,null);
}
public static String sendGetRequestWithToken(Context context,String urlString,String token){
return sendRequest(context,urlString,"GET",token,null);
}
//post请求
public static String sendPostRequest(Context context,String urlString){
return sendRequest(context,urlString,"POST",null,null);
}
public static String sendPostRequestWithToken(Context context,String urlString,String token){
return sendRequest(context,urlString,"POST",token,null);
}
public static String sendPostRequestWithData(Context context,String urlString,String data){
return sendRequest(context,urlString,"POST",null,data);
}
public static String sendPostRequestWithTakenWithData(Context context,String urlString,String token,String data){
return sendRequest(context,urlString,"POST", token,data);
}
}
- 1.
- 2.
- 3.
- 4.
- 5.
- 6.
- 7.
- 8.
- 9.
- 10.
- 11.
- 12.
- 13.
- 14.
- 15.
- 16.
- 17.
- 18.
- 19.
- 20.
- 21.
- 22.
- 23.
- 24.
- 25.
- 26.
- 27.
- 28.
- 29.
- 30.
- 31.
- 32.
- 33.
- 34.
- 35.
- 36.
- 37.
- 38.
- 39.
- 40.
- 41.
- 42.
- 43.
- 44.
- 45.
- 46.
- 47.
- 48.
- 49.
- 50.
- 51.
- 52.
- 53.
- 54.
- 55.
- 56.
- 57.
- 58.
- 59.
- 60.
- 61.
- 62.
- 63.
- 64.
- 65.
- 66.
- 67.
- 68.
- 69.
- 70.
- 71.
- 72.
- 73.
- 74.
- 75.
- 76.
- 77.
- 78.
- 79.
- 80.
- 81.
- 82.
- 83.
- 84.
- 85.
- 86.
- 87.
- 88.
- 89.
- 90.
- 91.
- 92.
- 93.
- 94.
- 95.
- 96.
- 97.
- 98.
- 99.
- 100.
- 101.
- 102.
- 103.
- 104.
- 105.
- 106.
- 107.
- 108.
- 109.
- 110.
- 111.
- 112.
- 113.
- 114.
5.在MainAbilitySlice.java文件中的onstart方法中(注意:访问网络要在子线程中完成):
6.接下来介绍一下如何引用第三方依赖(Gradle):
仓库的官方网址:仓库
6.1在搜索框搜索Gson,然后点击谷歌旗下的
6.2选择合适的版本,然后点击gradle(因为鸿蒙app开发使用的是gradle),复制如下的代码:
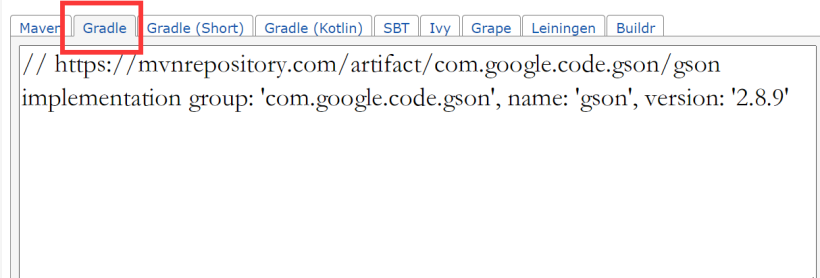
6.3然后在entry模块下的build.gradle文件下添加:
6.4此时便可以创建Gson对象了:
6.5主要调用这两个方法: