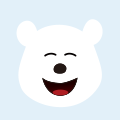
梅科尔工作室OpenHarmony设备开发培训笔记-第二章学习笔记
任务管理:
实例讲解(代码版):
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include "ohos_init.h"
#include "cmsis_os2.h"
osThreadId_t thread1ID;//定义任务ID
osThreadId_t thread2ID;//定义任务ID
/*****任务一*****/
void thread1(void)
{
printf("enter thread1\r\n");
osDelay(1);//导致thread1阻塞,thread2运行
printf("thread1 delay done\r\n");//thread1阻塞超时,thread1开始运行,thread2就绪
osThreadSuspend(thread1ID);//挂起thread1
printf("thread1 osThreadResume success\r\n");//在thread2里恢复thread1导致thread1运行,thread2就绪
osThreadTerminate(thread1ID);//删除thread1
printf("Hello\r\n");//删除thread1导致这行命令不能执行
// int sum = 0;
// while (1)
// {
// printf("This is BearPi Harmony Thread1----%d\r\n", sum++);
// usleep(1000000);
// }
}
/*****任务二*****/
void thread2(void)
{
for(int i = 0; i < 20; i++){
printf("enter thread2 frequency is %d\r\n", i);
}
printf("thread1 osThreadSuspend success\r\n");//在thread1里挂起thread1导致thread1阻塞,thread2运行
osThreadResume(thread1ID);//恢复thread1
osThreadTerminate(thread2ID);//删除thread2
printf("World\r\n");//删除thread2导致这行命令不能执行
// int sum = 0;
// while (1)
// {
// printf("This is BearPi Harmony Thread2----%d\r\n", sum++);
// usleep(500000);
// }
}
/*****任务创建*****/
static void Thread_example(void)
{
osThreadAttr_t attr;
attr.name = "thread1";
attr.attr_bits = 0U;
attr.cb_mem = NULL;
attr.cb_size = 0U;
attr.stack_mem = NULL;
attr.stack_size = 1024 * 4;
attr.priority = 25;
thread1ID = osThreadNew((osThreadFunc_t)thread1, NULL, &attr);//获取任务ID
thread2ID = osThreadNew((osThreadFunc_t)thread2, NULL, &attr);//获取任务ID
if (thread1ID == NULL)
{
printf("Falied to create thread1!\n");
}
attr.name = "thread2";
if (thread2ID == NULL)
{
printf("Falied to create thread2!\n");
}
}
APP_FEATURE_INIT(Thread_example);
------------------------------------------------------------------------
软件定时器:
实例讲解(代码版):
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include "ohos_init.h"
#include "cmsis_os2.h"
uint32_t exec1, exec2;
/***** 定时器1 回调函数 *****/
void Timer1_Callback(void *arg)
{
(void)arg;
printf("This is BearPi Harmony Timer1_Callback!\r\n");
}
/***** 定时器2 回调函数 *****/
void Timer2_Callback(void *arg)
{
(void)arg;
printf("This is BearPi Harmony Timer2_Callback!\r\n");
}
/***** 定时器创建 *****/
static void Timer_example(void)
{
osTimerId_t id1, id2;
uint32_t timerDelay;
osStatus_t status;
exec1 = 1U;
id1 = osTimerNew(Timer1_Callback, osTimerOnce, &exec1, NULL);//单次定时器
if (id1 != NULL)
{
// Hi3861 1U=10ms,100U=1S
timerDelay = 100U;
status = osTimerStart(id1, timerDelay);
if (status != osOK)
{
// Timer could not be started
}
}
osDelay(50U);//必须小于100U,因为停止定时器函数只能在定时器启动时才能正常工作,而Timer1_Callback单次定时器在100U后自动删除
status = osTimerStop(id1);//停止定时器
if(status != osOK)
{
printf("stop Timer1 falled\r\n");
}
else{
printf("stop Timer1 success\r\n");
}
status = osTimerStart(id1, timerDelay);//重启定时器
if (status != osOK)
{
printf("start Timer1_Callback failed\r\n");
}
osDelay(200U);//删除定时器函数无论定时器是否在启动中都可以正常工作
status = osTimerDelete(id1);//删除定时器
if (status != osOK)
{
printf("Delete Timer1_Callback failed\r\n");
}
exec2 = 1U;
id2 = osTimerNew(Timer2_Callback, osTimerPeriodic, &exec2, NULL);//循环定时器
if (id2 != NULL)
{
// Hi3861 1U=10ms,300U=3S
timerDelay = 300U;
status = osTimerStart(id2, timerDelay);
if (status != osOK)
{
// Timer could not be started
}
}
}
APP_FEATURE_INIT(Timer_example);
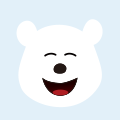