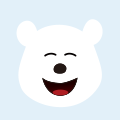
回复
效果是实现的获取Mysql所有的数据库,然后单击数据库名称以获取数据库中的所有表。
它主要通过DefaultComboBoxModel实现。 思路是删除先前的模型,然后将数据重新添加到该模型,从而实现二级联动。
给出参考代码:
package com.xmlDemo.frame;
import java.awt.FlowLayout;
import java.awt.Image;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import javax.swing.ComboBoxModel;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import com.xmlDemo.service.DBService;
import com.xmlDemo.service.DBToXmlService;
import com.xmlDemo.util.DBConnectionUtil;
//主要是用DefaultComboBoxModel来实现
public class ExportFrame extends JFrame implements ItemListener{
/**
*
*/
private static final long serialVersionUID = 1L;
String dbName = "xml";
private List<String> list2;
private String[] arrs2 = {};
private DefaultComboBoxModel model;
private JComboBox comboBox1;
private JComboBox comboBox2;
private final static String BASEURL="../xmlDemo/images/";
private String tableName = "users";
public ExportFrame(){
try {
export();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void export() throws Exception{
Image image=Toolkit.getDefaultToolkit().getImage(BASEURL+"logo.png");
setIconImage(image);
setTitle("导出信息");
setLayout(new FlowLayout(FlowLayout.LEFT));
JPanel panel1 = new JPanel();
JPanel panel2 = new JPanel();
JLabel label1 = new JLabel("数据库:");
List<String> list1 = new DBService().getAllDatabases();
String arrs1[] = new String[list1.size()];
for(int i = 0; i < list1.size(); i++){
arrs1[i] = list1.get(i);
}
comboBox1 = new JComboBox(arrs1);
comboBox1.setSelectedItem(dbName);
comboBox1.addItemListener(this);
panel1.add(label1);
panel1.add(comboBox1);
list2 = new DBService().getAllTables(dbName);
arrs2 = new String[list2.size()];
for(int i = 0; i < list2.size(); i++){
arrs2[i] = list2.get(i);
}
JLabel label2 = new JLabel("数据表:");
//创建一个模型
model = new DefaultComboBoxModel();
try {
list2 = new DBService().getAllTables(dbName);
} catch (Exception e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
arrs2 = new String[list2.size()];
for(int i = 0; i < list2.size(); i++){
arrs2[i] = list2.get(i);
model.addElement(arrs2[i]);
}
comboBox2 = new JComboBox();
comboBox2.setSelectedItem(tableName);
comboBox2.setModel(model);
comboBox2.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
// TODO Auto-generated method stub
if(e.getStateChange()==ItemEvent.SELECTED){
tableName = "" + e.getItem();
}
}
});
panel2.add(label2);
panel2.add(comboBox2);
JButton btn = new JButton("导出");
btn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
try {
if(dbName.equals("xml")){
new DBToXmlService().exportDataToXMlFile(dbName, tableName);
}else{
JOptionPane.showConfirmDialog(null, "当前版本只支持特定数据库","温馨提示",JOptionPane.YES_NO_OPTION);
}
} catch (Exception e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
add(panel1);
add(panel2);
add(btn);
setVisible(true);
setSize(400,400);
setLocation(300,300);
}
@Override
public void itemStateChanged(ItemEvent e) {
// TODO Auto-generated method stub
if(e.getStateChange()==ItemEvent.SELECTED){//选中JComboBox组件
dbName = ""+e.getItem();//获取字符串
try {
list2 = new DBService().getAllTables(dbName);
} catch (Exception e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
//@SuppressWarnings("unchecked")
model = (DefaultComboBoxModel)comboBox2.getModel();
//移除之前的ComboBox模型
while(model.getSize()>0){
model.removeElementAt(model.getSize()-1);
}
arrs2 = new String[list2.size()];
//重新添加模型
for(int i = 0;i<list2.size();i++){
arrs2[i] = list2.get(i);
model.addElement(arrs2[i]);
}
}
}
}
数据库操作的类:
package com.xmlDemo.service;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.xmlDemo.util.DBConnectionUtil;
public class DBService {
//获取某个数据库的所有数据表
public List<String> getAllTables(String databaseName) throws Exception{
List<String> list = new ArrayList<String>();
int i = 0;
String url = "jdbc:mysql://localhost:3306/"+databaseName;
Connection connection = new DBConnectionUtil().getConnection(url);
try {
ResultSet rs=connection.getMetaData().getTables("","","",null);
while (rs.next()) {
list.add(rs.getString("TABLE_NAME"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return list;
}
public List<String> getAllDatabases() throws Exception{
List<String> list = new ArrayList<String>();
int i = 0;
String sql = "show databases";
String url="jdbc:mysql://localhost:3306?useUnicode=true&characterEncoding=UTF-8";
Connection connection = new DBConnectionUtil().getConnection(url);
try {
PreparedStatement prepare = connection.prepareStatement(sql);
ResultSet rs=prepare.executeQuery();
while (rs.next()) {
list.add(rs.getString(1));
}
} catch (SQLException e) {
e.printStackTrace();
}finally{
new DBConnectionUtil().close();
}
return list;
}
}