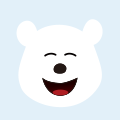
OpenHarmony三方组件:mp4parser
简介
一个读取、写入操作音视频文件编辑的工具。
编译运行
1、通过IDE工具下载依赖SDK,Tools->SDK Manager->Openharmony SDK 把native选项勾上下载,API版本>=9
2、开发板选择RK3568,ROM下载地址. 选择开发板类型是rk3568,请使用最新的版本
3、项目中需要用到资源文件,例如mp4、mp3文件,自行下载素材添加到
entry\src\main\resources\base\media下面
4、项目中需要引用libohffmpeg.so,FFmpeg需要自行编译,要放到library模块 libs\armeabi-v7a和libs\arm64-v8a下面,参考FFmpeg源码链接. 另外FFmpeg的头文件需要拷贝到目录:mp4parser\src\main\cpp\include。 关于FFmpeg编译,请参考:Openharmony编译构建指导. 编译脚本参考详见目录:doc/
下载安装
npm install @ohos/mp4parser --save
OpenHarmony npm环境配置等更多内容,请参考 如何安装OpenHarmony npm包 。
使用说明
视频合成
import {MP4Parser} from "@ohos/mp4parser";
import {ICallBack} from "@ohos/mp4parser";
/**
* 视频合成
*/
private videoMerge() {
let getLocalDirPath = getContext(this).cacheDir+"/";
let that = this;
let filePathOne = getLocalDirPath + "qqq.mp4";
let filePathTwo = getLocalDirPath + "www.mp4";
let outMP4 = getLocalDirPath + "mergeout.mp4";
var callBack: ICallBack = {
callBackResult(code: number) {
that.btnText = "视频合成点击执行"
that.imageWidth = 0
that.imageHeight = 0
if (code == 0) {
AlertDialog.show({ message: '执行成功' })
}
else {
AlertDialog.show({ message: '执行失败' })
}
}
}
MP4Parser.videoMerge(filePathOne, filePathTwo, outMP4, callBack);
}
视频裁剪
import {MP4Parser} from "@ohos/mp4parser";
import {ICallBack} from "@ohos/mp4parser";
/**
* 视频裁剪
*/
private videoClip() {
let getLocalDirPath = getContext(this).cacheDir+"/";
let that=this;
let sTime = "00:00:10";
let eTime = "00:00:20";
let sourceMP4 = getLocalDirPath+"qqq.mp4";
let outMP4 = getLocalDirPath+"clipout.mp4";
var callBack: ICallBack = {
callBackResult(code: number) {
that.btnText="视频裁剪点击执行"
that.imageWidth=0
that.imageHeight=0
if (code == 0) {
AlertDialog.show({ message: '执行成功' })
}
else {
AlertDialog.show({ message: '执行失败' })
}
}
}
MP4Parser.videoClip(sTime, eTime, sourceMP4, outMP4, callBack);
}
音频合成
import {MP4Parser} from "@ohos/mp4parser";
import {ICallBack} from "@ohos/mp4parser";
/**
* 音频合成
*/
private audioMerge() {
let getLocalDirPath = getContext(this).cacheDir+"/";
let that = this;
let filePathOne = getLocalDirPath + "a.mp3";
let filePathTwo = getLocalDirPath + "b.mp3";
let outPath = getLocalDirPath + "mergeout.mp3";
var callBack: ICallBack = {
callBackResult(code: number) {
console.log("mp4parser-->audioMerge--->end");
that.btnText = "音频合成点击执行"
that.imageWidth = 0
that.imageHeight = 0
if (code == 0) {
AlertDialog.show({ message: '执行成功' })
}
else {
AlertDialog.show({ message: '执行失败' })
}
}
}
MP4Parser.audioMerge(filePathOne, filePathTwo, outPath, callBack);
}
音频裁剪
import {MP4Parser} from "@ohos/mp4parser";
import {ICallBack} from "@ohos/mp4parser";
/**
* 音频裁剪
*/
private audioClip() {
let getLocalDirPath = getContext(this).cacheDir+"/";
let that = this;
let sTime = "00:00:00";
let eTime = "00:00:10";
let sourcePath = getLocalDirPath + "a.mp3";
let outPath = getLocalDirPath + "clipout.mp3";
var callBack: ICallBack = {
callBackResult(code: number) {
that.btnText = "音频裁剪点击执行"
that.imageWidth = 0
that.imageHeight = 0
if (code == 0) {
AlertDialog.show({ message: '执行成功' })
}
else {
AlertDialog.show({ message: '执行失败' })
}
}
}
MP4Parser.audioClip(sTime, eTime, sourcePath, outPath, callBack);
}
视频批量合成
import {MP4Parser} from "@ohos/mp4parser";
import {ICallBack} from "@ohos/mp4parser";
/**
* 视频批量合成
*/
private videoMultMerge() {
let that = this;
let getLocalDirPath = getContext(this).cacheDir+"/";
let filePath = getLocalDirPath + "mergeList.txt";
let outMP4 = getLocalDirPath + "mergeout3.mp4";
var callBack: ICallBack = {
callBackResult(code: number) {
that.btnText2 = "视频合成点击执行"
that.imageWidth = 0
that.imageHeight = 0
if (code == 0) {
AlertDialog.show({ message: '执行成功' })
}
else {
AlertDialog.show({ message: '执行失败' })
}
}
}
MP4Parser.videoMultMerge(filePath, outMP4, callBack);
}
音频批量合成
import {MP4Parser} from "@ohos/mp4parser";
import {ICallBack} from "@ohos/mp4parser";
/**
* 音频批量合成
*/
private audioMultMerge() {
let getLocalDirPath = getContext(this).cacheDir+"/";
let that = this;
let filePath = getLocalDirPath + "mergemp3List.txt";
let outPath = getLocalDirPath + "mergeout3.mp3";
var callBack: ICallBack = {
callBackResult(code: number) {
that.btnText2 = "音频合成点击执行"
that.imageWidth = 0
that.imageHeight = 0
if (code == 0) {
AlertDialog.show({ message: '执行成功' })
}
else {
AlertDialog.show({ message: '执行失败' })
}
}
}
MP4Parser.audioMultMerge(filePath, outPath, callBack);
}
接口说明
import {MP4Parser} from "@ohos/mp4parser";
- 视频合成
MP4Parser.videoMerge()
- 视频裁剪
MP4Parser.videoClip()
- 批量视频合成
MP4Parser.videoMultMerge()
- 音频合成
MP4Parser.audioMerge()
- 音频裁剪
MP4Parser.audioClip()
- 音频批量合成
MP4Parser.audioMultMerge()
兼容性
- DevEco Studio 版本:DevEco Studio 3.1 Beta1及以上版本。
- OpenHarmony SDK版本:API version 9 及以上版本。
目录结构
|---- mp4parser
| |---- entry # 示例代码文件夹
| |---- mp4parser # mp4parser库文件夹
| |---- MP4Parser.ets # 对外接口
| |---- README.MD # 安装使用方法
贡献代码
使用过程中发现任何问题都可以提 Issue 给我们,当然,我们也非常欢迎你给我们发 PR 。
开源协议
本项目基于 Apache License 2.0 ,请自由地享受和参与开源。
文章转载自:https://gitee.com/openharmony-tpc/mp4parser
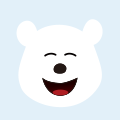