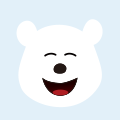
OpenHarmony三方组件:MaterialFloatingActionButton
Material FAB replicates the floating action buttons(FAB) using material design 3 specifications in openHarmony.
Dependencies
For using materialfab in your app, add the below dependency in entry/package.json
"dependencies": {
"@ohos/materialfab": "file:../materialfab"
}
Usage Instructions
Import all components at once
import {FAB_extended, FAB_regular, FabType, FabExtendedModel, FabRegularModel} from '@ohos/materialfab'
Screenshots
Class CommonProperties
Methods
Name | Return Type | Description |
| | sets icon for the fab. |
| | return fab icon. |
| | sets custom icon size. |
| | returns icon size. |
| | sets padding to the icon. |
| | return icon padding. |
| | sets custom ripple model. |
| | return current ripple model. |
| | sets custom fab size. |
| | return current fab size. |
| | sets fab background color. |
| | return fab background color. |
| | sets custom border for the fab. |
| | return current border specs. |
| | sets fab position. |
| | return current position of the fab. |
| | sets custom ripple color. |
| | return current ripple color. |
Class FabRegularModel.Model
Implementation of Regular FAB buttons with size options as SMALL, MEDIUM and LARGE.
Methods
Name | Return Type | Description |
| | sets fab type. Defaults to FabType.MEDIUM |
| | return current fab type. |
FabType enum
Name | Description |
| Small fab as per material design 3 specifications. |
| Medium fab as per material design 3 specifications. |
| Large fab as per material design 3 specifications. |
Usage/Examples
SMALL FAB
import {FAB_regular, FabRegularModel, FabType} from '@ohos/materialfab'
@Entry
@Component
struct SMALL_fab_demo {
model : FabRegularModel.Model = new FabRegularModel.Model().setFabType(FabType.SMALL)
aboutToAppear(){
this.model.setPosition({x : 100, y : 300})
}
build() {
Column() {
FAB_regular({
model : this.model,
onClick : (event) => {
AlertDialog.show({ message: 'You clicked SMALL fab' })
}
})
}.height('100%')
}
}
MEDIUM FAB
import {FAB_regular, FabRegularModel, FabType} from '@ohos/materialfab'
@Entry
@Component
struct MEDIUM_fab_demo {
model : FabRegularModel.Model = new FabRegularModel.Model().setFabType(FabType.MEDIUM)
aboutToAppear(){
this.model.setPosition({x : 100, y : 300})
this.model.setBackgroundColor(Color.Green)
}
build() {
Column() {
FAB_regular({
model : this.model,
onClick : (event) => {
AlertDialog.show({ message: 'You clicked MEDIUM fab' })
}
})
}.height('100%')
}
}
LARGE FAB
import {FAB_regular, FabRegularModel, FabType} from '@ohos/materialfab'
@Entry
@Component
struct LARGE_fab_demo {
model : FabRegularModel.Model = new FabRegularModel.Model().setFabType(FabType.LARGE)
aboutToAppear(){
this.model.setPosition({x : 100, y : 300})
this.model.setBackgroundColor(Color.Red)
}
build() {
Column() {
FAB_regular({
model : this.model,
onClick : (event) => {
AlertDialog.show({ message: 'You clicked LARGE fab' })
}
})
}.height('100%')
}
}
Class FabExtendedModel.Model
Implementation of Extended FAB buttons with an options for addition of leading icon.
Methods
Name | Return Type | Description |
| | sets label text. |
| | return label text. |
| | sets text color. |
| | return text color. |
| | sets font style. |
| | returns font style. |
| | sets text size. |
| | returns current text size. |
| | sets font weight. |
| | returns font weight. |
| | sets text padding. |
| | return current text padding. |
| | sets maximum allowed width for fab. |
| | return maximum allowed width for the fab. |
Usage/Examples
With Leading Icon
import {FAB_extended, FabExtendedModel} from '@ohos/materialfab'
@Entry
@Component
struct extended_fab_demo {
model : FabExtendedModel.Model = new FabExtendedModel.Model()
aboutToAppear(){
this.model.setPosition({x : 100, y : 300})
this.model.setFabIcon($r('app.media.add_icon'))
}
build() {
Column() {
FAB_extended({
model : this.model,
onClick : (event) => {
AlertDialog.show({ message: 'You clicked extended fab' })
}
})
}.height('100%')
}
}
Without Leading Icon
import {FAB_extended, FabExtendedModel} from '@ohos/materialfab'
@Entry
@Component
struct extended_fab_demo {
model : FabExtendedModel.Model = new FabExtendedModel.Model()
aboutToAppear(){
this.model.setPosition({x : 100, y : 300})
this.model.setBackgroundColor(Color.Gray)
}
build() {
Column() {
FAB_extended({
model : this.model,
onClick : (event) => {
AlertDialog.show({ message: 'You clicked extended fab' })
}
})
}.height('100%')
}
}
Compatibility
Supports OpenHarmony API version 9
Code Contribution
If you find any problems during usage, you can submit an Issue to us. Of course, we also welcome you to send us PR.
Open source License
This project is based on Apache License 2.0, please enjoy and participate in open source freely.
文章转载自:https://gitee.com/openharmony-tpc/MaterialFloatingActionButton
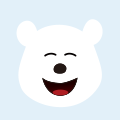