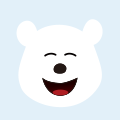
扔掉okhttp、httpClient,来试试这款轻量级 HTTP 客户端框架,吹爆!
大家好,我是不才陈某~
在SpringBoot项目直接使用okhttp、httpClient或者RestTemplate发起HTTP请求,既繁琐又不方便统一管理。
因此,在这里推荐一个适用于SpringBoot项目的轻量级HTTP客户端框架retrofit-spring-boot-starter
,使用非常简单方便,同时又提供诸多功能增强。
适用于retrofit的spring-boot-starter,支持快速集成和功能增强。
- Spring Boot 3.x 项目,请使用retrofit-spring-boot-starter 3.x。
- Spring Boot 1.x/2.x 项目,请使用retrofit-spring-boot-starter 2.x。
github项目地址:
https://github.com/LianjiaTech/retrofit-spring-boot-starter
gitee项目地址:
https://gitee.com/lianjiatech/retrofit-spring-boot-starter
功能特性
- 自定义OkHttpClient
- 注解式拦截器
- 日志打印
- 请求重试
- 熔断降级
- 错误解码器
- 微服务之间的HTTP调用
- 全局拦截器
- 调用适配器
- 数据转换器
- 元注解
- 其他功能示例
快速开始
引入依赖
<dependency>
<groupId>com.github.lianjiatech</groupId>
<artifactId>retrofit-spring-boot-starter</artifactId>
<version>3.0.2</version>
</dependency>
如果启动失败,大概率是依赖冲突,烦请引入或者排除相关依赖。
定义HTTP接口
接口必须使用@RetrofitClient
注解标记!
@RetrofitClient(baseUrl = "${test.baseUrl}")
public interface HttpApi {
@GET("person")
Result<Person> getPerson(@Query("id") Long id);
}
注意:方法请求路径慎用
/
开头。对于Retrofit
而言,如果baseUrl=http://localhost:8080/api/test/
,方法请求路径如果是person
,则该方法完整的请求路径是:http://localhost:8080/api/test/person
。而方法请求路径如果是/person
,则该方法完整的请求路径是:http://localhost:8080/person
。
注入使用
将接口注入到其它Service中即可使用!
@Service
public class TestService {
@Autowired
private HttpApi httpApi;
public void test() {
// 使用`httpApi`发起HTTP请求
}
}
默认情况下,自动使用SpringBoot
扫描路径进行RetrofitClient
注册。你也可以在配置类加上@RetrofitScan
手工指定扫描路径。关注工众号:码猿技术专栏,回复关键词:1111 获取阿里内部Java性能调优手册!
HTTP请求相关注解
HTTP
请求相关注解,全部使用了Retrofit
原生注解,以下是一个简单说明:
注解分类 | 支持的注解 |
请求方式 | |
请求头 | |
Query参数 | |
path参数 | |
form-encoded参数 | |
请求体 | |
文件上传 | |
url参数 | |
配置属性
组件支持了多个可配置的属性,用来应对不同的业务场景,具体可支持的配置属性及默认值如下:
注意:应用只需要配置要更改的配置项!
retrofit:
# 全局转换器工厂
global-converter-factories:
- com.github.lianjiatech.retrofit.spring.boot.core.BasicTypeConverterFactory
- retrofit2.converter.jackson.JacksonConverterFactory
# 全局调用适配器工厂(组件扩展的调用适配器工厂已经内置,这里请勿重复配置)
global-call-adapter-factories:
# 全局日志打印配置
global-log:
# 启用日志打印
enable: true
# 全局日志打印级别
log-level: info
# 全局日志打印策略
log-strategy: basic
# 全局重试配置
global-retry:
# 是否启用全局重试
enable: false
# 全局重试间隔时间
interval-ms: 100
# 全局最大重试次数
max-retries: 2
# 全局重试规则
retry-rules:
- response_status_not_2xx
- occur_io_exception
# 全局超时时间配置
global-timeout:
# 全局读取超时时间
read-timeout-ms: 10000
# 全局写入超时时间
write-timeout-ms: 10000
# 全局连接超时时间
connect-timeout-ms: 10000
# 全局完整调用超时时间
call-timeout-ms: 0
# 熔断降级配置
degrade:
# 熔断降级类型。默认none,表示不启用熔断降级
degrade-type: none
# 全局sentinel降级配置
global-sentinel-degrade:
# 是否开启
enable: false
# 各降级策略对应的阈值。平均响应时间(ms),异常比例(0-1),异常数量(1-N)
count: 1000
# 熔断时长,单位为 s
time-window: 5
# 降级策略(0:平均响应时间;1:异常比例;2:异常数量)
grade: 0
# 全局resilience4j降级配置
global-resilience4j-degrade:
# 是否开启
enable: false
# 根据该名称从#{@link CircuitBreakerConfigRegistry}获取CircuitBreakerConfig,作为全局熔断配置
circuit-breaker-config-name: defaultCircuitBreakerConfig
# 自动设置PathMathInterceptor的scope为prototype
auto-set-prototype-scope-for-path-math-interceptor: true
高级功能
超时时间配置
如果仅仅需要修改OkHttpClient
的超时时间,可以通过@RetrofitClient
相关字段修改,或者全局超时配置修改。
自定义OkHttpClient
如果需要修改OkHttpClient
其它配置,可以通过自定义OkHttpClient
来实现,步骤如下:
1.实现SourceOkHttpClientRegistrar
接口,调用SourceOkHttpClientRegistry#register()
方法注册OkHttpClient
。
@Slf4j
@Component
public class CustomSourceOkHttpClientRegistrar implements SourceOkHttpClientRegistrar {
@Override
public void register(SourceOkHttpClientRegistry registry) {
// 添加testSourceOkHttpClient
registry.register("testSourceOkHttpClient", new OkHttpClient.Builder()
.connectTimeout(Duration.ofSeconds(3))
.writeTimeout(Duration.ofSeconds(3))
.readTimeout(Duration.ofSeconds(3))
.addInterceptor(chain -> {
log.info("============use testSourceOkHttpClient=============");
return chain.proceed(chain.request());
})
.build());
}
}
2.通过@RetrofitClient.sourceOkHttpClient
指定当前接口要使用的OkHttpClient
。
@RetrofitClient(baseUrl = "${test.baseUrl}", sourceOkHttpClient = "testSourceOkHttpClient")
public interface CustomOkHttpTestApi {
@GET("person")
Result<Person> getPerson(@Query("id") Long id);
}
注意:组件不会直接使用指定的
OkHttpClient
,而是基于该OkHttpClient
创建一个新的。
注解式拦截器
组件提供了注解式拦截器,支持基于url路径匹配拦截,使用的步骤如下:
- 继承
BasePathMatchInterceptor
- 使用
@Intercept
注解指定要使用的拦截器
如果需要使用多个拦截器,在接口上标注多个
@Intercept
注解即可。
下面以"给指定请求的url后面拼接timestamp时间戳"为例,介绍下如何使用注解式拦截器。
继承BasePathMatchInterceptor
编写拦截处理器
@Component
public class TimeStampInterceptor extends BasePathMatchInterceptor {
@Override
public Response doIntercept(Chain chain) throws IOException {
Request request = chain.request();
HttpUrl url = request.url();
long timestamp = System.currentTimeMillis();
HttpUrl newUrl = url.newBuilder()
.addQueryParameter("timestamp", String.valueOf(timestamp))
.build();
Request newRequest = request.newBuilder()
.url(newUrl)
.build();
return chain.proceed(newRequest);
}
}
默认情况下,组件会自动将BasePathMatchInterceptor
的scope
设置为prototype
。
可通过retrofit.auto-set-prototype-scope-for-path-math-interceptor=false
关闭该功能。关闭之后,需要手动将scope
设置为prototype
。
@Component
@Scope("prototype")
public class TimeStampInterceptor extends BasePathMatchInterceptor {
@Override
public Response doIntercept(Chain chain) throws IOException {
// ...
}
}
接口上使用@Intercept
进行标注
@RetrofitClient(baseUrl = "${test.baseUrl}")
@Intercept(handler = TimeStampInterceptor.class, include = {"/api/**"}, exclude = "/api/test/savePerson")
@Intercept(handler = TimeStamp2Interceptor.class) // 需要多个,直接添加即可
public interface HttpApi {
@GET("person")
Result<Person> getPerson(@Query("id") Long id);
@POST("savePerson")
Result<Person> savePerson(@Body Person person);
}
上面的@Intercept
配置表示:拦截HttpApi
接口下/api/**
路径下(排除/api/test/savePerson
)的请求,拦截处理器使用TimeStampInterceptor
。
自定义拦截注解
有的时候,我们需要在"拦截注解"动态传入一些参数,然后在拦截的时候使用这些参数。这时候,我们可以使用"自定义拦截注解",步骤如下:
- 自定义注解。必须使用
@InterceptMark
标记,并且注解中必须包括include、exclude、handler
字段。 - 继承
BasePathMatchInterceptor
编写拦截处理器 - 接口上使用自定义注解
例如,我们需要"在请求头里面动态加入accessKeyId
、accessKeySecret
签名信息才能再发起HTTP请求",这时候可以自定义@Sign
注解来实现。
自定义@Sign
注解
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@Documented
@InterceptMark
public @interface Sign {
String accessKeyId();
String accessKeySecret();
String[] include() default {"/**"};
String[] exclude() default {};
Class<? extends BasePathMatchInterceptor> handler() default SignInterceptor.class;
}
在@Sign
注解中指定了使用的拦截器是SignInterceptor
。
实现SignInterceptor
@Component
public class SignInterceptor extends BasePathMatchInterceptor {
private String accessKeyId;
private String accessKeySecret;
public void setAccessKeyId(String accessKeyId) {
this.accessKeyId = accessKeyId;
}
public void setAccessKeySecret(String accessKeySecret) {
this.accessKeySecret = accessKeySecret;
}
@Override
public Response doIntercept(Chain chain) throws IOException {
Request request = chain.request();
Request newReq = request.newBuilder()
.addHeader("accessKeyId", accessKeyId)
.addHeader("accessKeySecret", accessKeySecret)
.build();
return chain.proceed(newReq);
}
}
注意:
accessKeyId
和accessKeySecret
字段必须提供setter
方法。
拦截器的accessKeyId
和accessKeySecret
字段值会依据@Sign
注解的accessKeyId()
和accessKeySecret()
值自动注入,如果@Sign
指定的是占位符形式的字符串,则会取配置属性值进行注入。
接口上使用@Sign
@RetrofitClient(baseUrl = "${test.baseUrl}")
@Sign(accessKeyId = "${test.accessKeyId}", accessKeySecret = "${test.accessKeySecret}", exclude = {"/api/test/person"})
public interface HttpApi {
@GET("person")
Result<Person> getPerson(@Query("id") Long id);
@POST("savePerson")
Result<Person> savePerson(@Body Person person);
}
日志打印
组件支持支持全局日志打印和声明式日志打印。
全局日志打印
默认情况下,全局日志打印是开启的,默认配置如下:
retrofit:
# 全局日志打印配置
global-log:
# 启用日志打印
enable: true
# 全局日志打印级别
log-level: info
# 全局日志打印策略
log-strategy: basic
四种日志打印策略含义如下:
-
NONE
:No logs. -
BASIC
:Logs request and response lines. -
HEADERS
:Logs request and response lines and their respective headers. -
BODY
:Logs request and response lines and their respective headers and bodies (if present).
声明式日志打印
如果只需要部分请求才打印日志,可以在相关接口或者方法上使用@Logging
注解。
日志打印自定义扩展
如果需要修改日志打印行为,可以继承LoggingInterceptor
,并将其配置成Spring bean
。
聚合日志打印
如果需要将同一个请求的日志聚合在一起打印,可配置AggregateLoggingInterceptor
。
@Bean
public LoggingInterceptor loggingInterceptor(RetrofitProperties retrofitProperties){
return new AggregateLoggingInterceptor(retrofitProperties.getGlobalLog());
}
请求重试
组件支持支持全局重试和声明式重试。
全局重试
全局重试默认关闭,默认配置项如下:
retrofit:
# 全局重试配置
global-retry:
# 是否启用全局重试
enable: false
# 全局重试间隔时间
interval-ms: 100
# 全局最大重试次数
max-retries: 2
# 全局重试规则
retry-rules:
- response_status_not_2xx
- occur_io_exception
重试规则支持三种配置:
-
RESPONSE_STATUS_NOT_2XX
:响应状态码不是2xx
时执行重试 -
OCCUR_IO_EXCEPTION
:发生IO异常时执行重试 -
OCCUR_EXCEPTION
:发生任意异常时执行重试
声明式重试
如果只有一部分请求需要重试,可以在相应的接口或者方法上使用@Retry
注解。
请求重试自定义扩展
如果需要修改请求重试行为,可以继承RetryInterceptor
,并将其配置成Spring bean
。
熔断降级
熔断降级默认关闭,当前支持sentinel
和resilience4j
两种实现。
retrofit:
# 熔断降级配置
degrade:
# 熔断降级类型。默认none,表示不启用熔断降级
degrade-type: sentinel
Sentinel
配置degrade-type=sentinel
开启,然后在相关接口或者方法上声明@SentinelDegrade
注解即可。
记得手动引入Sentinel
依赖:
<dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>sentinel-core</artifactId>
<version>1.6.3</version>
</dependency>
此外,还支持全局Sentinel
熔断降级:
retrofit:
# 熔断降级配置
degrade:
# 熔断降级类型。默认none,表示不启用熔断降级
degrade-type: sentinel
# 全局sentinel降级配置
global-sentinel-degrade:
# 是否开启
enable: true
# ...其他sentinel全局配置
Resilience4j
配置degrade-type=resilience4j
开启。然后在相关接口或者方法上声明@Resilience4jDegrade
即可。
记得手动引入Resilience4j
依赖:
<dependency>
<groupId>io.github.resilience4j</groupId>
<artifactId>resilience4j-circuitbreaker</artifactId>
<version>1.7.1</version>
</dependency>
通过以下配置可开启全局resilience4j熔断降级:
retrofit:
# 熔断降级配置
degrade:
# 熔断降级类型。默认none,表示不启用熔断降级
degrade-type: resilience4j
# 全局resilience4j降级配置
global-resilience4j-degrade:
# 是否开启
enable: true
# 根据该名称从#{@link CircuitBreakerConfigRegistry}获取CircuitBreakerConfig,作为全局熔断配置
circuit-breaker-config-name: defaultCircuitBreakerConfig
熔断配置管理:
1.实现CircuitBreakerConfigRegistrar
接口,注册CircuitBreakerConfig
。
@Component
public class CustomCircuitBreakerConfigRegistrar implements CircuitBreakerConfigRegistrar {
@Override
public void register(CircuitBreakerConfigRegistry registry) {
// 替换默认的CircuitBreakerConfig
registry.register(Constants.DEFAULT_CIRCUIT_BREAKER_CONFIG, CircuitBreakerConfig.ofDefaults());
// 注册其它的CircuitBreakerConfig
registry.register("testCircuitBreakerConfig", CircuitBreakerConfig.custom()
.slidingWindowType(CircuitBreakerConfig.SlidingWindowType.TIME_BASED)
.failureRateThreshold(20)
.minimumNumberOfCalls(5)
.permittedNumberOfCallsInHalfOpenState(5)
.build());
}
}
2.通过circuitBreakerConfigName
指定CircuitBreakerConfig
。包括retrofit.degrade.global-resilience4j-degrade.circuit-breaker-config-name
或者@Resilience4jDegrade.circuitBreakerConfigName
扩展熔断降级
如果用户需要使用其他的熔断降级实现,继承BaseRetrofitDegrade
,并将其配置Spring Bean
。
配置fallback或者fallbackFactory (可选)
如果@RetrofitClient
不设置fallback
或者fallbackFactory
,当触发熔断时,会直接抛出RetrofitBlockException
异常。用户可以通过设置fallback
或者fallbackFactory
来定制熔断时的方法返回值。
注意:
fallback
类必须是当前接口的实现类,fallbackFactory
必须是FallbackFactory<T>
实现类,泛型参数类型为当前接口类型。另外,fallback
和fallbackFactory
实例必须配置成Spring Bean
。
fallbackFactory
相对于fallback
,主要差别在于能够感知每次熔断的异常原因(cause),参考示例如下:
@Slf4j
@Service
public class HttpDegradeFallback implements HttpDegradeApi {
@Override
public Result<Integer> test() {
Result<Integer> fallback = new Result<>();
fallback.setCode(100)
.setMsg("fallback")
.setBody(1000000);
return fallback;
}
}
@Slf4j
@Service
public class HttpDegradeFallbackFactory implements FallbackFactory<HttpDegradeApi> {
@Override
public HttpDegradeApi create(Throwable cause) {
log.error("触发熔断了! ", cause.getMessage(), cause);
return new HttpDegradeApi() {
@Override
public Result<Integer> test() {
Result<Integer> fallback = new Result<>();
fallback.setCode(100)
.setMsg("fallback")
.setBody(1000000);
return fallback;
}
};
}
}
错误解码器
在HTTP
发生请求错误(包括发生异常或者响应数据不符合预期)的时候,错误解码器可将HTTP
相关信息解码到自定义异常中。你可以在@RetrofitClient
注解的errorDecoder()
指定当前接口的错误解码器,自定义错误解码器需要实现ErrorDecoder
接口:
微服务之间的HTTP调用
继承ServiceInstanceChooser
用户可以自行实现ServiceInstanceChooser
接口,完成服务实例的选取逻辑,并将其配置成Spring Bean
。对于Spring Cloud
应用,可以使用如下实现。
@Service
public class SpringCloudServiceInstanceChooser implements ServiceInstanceChooser {
private LoadBalancerClient loadBalancerClient;
@Autowired
public SpringCloudServiceInstanceChooser(LoadBalancerClient loadBalancerClient) {
this.loadBalancerClient = loadBalancerClient;
}
/**
* Chooses a ServiceInstance URI from the LoadBalancer for the specified service.
*
* @param serviceId The service ID to look up the LoadBalancer.
* @return Return the uri of ServiceInstance
*/
@Override
public URI choose(String serviceId) {
ServiceInstance serviceInstance = loadBalancerClient.choose(serviceId);
Assert.notNull(serviceInstance, "can not found service instance! serviceId=" + serviceId);
return serviceInstance.getUri();
}
}
指定serviceId
和path
@RetrofitClient(serviceId = "${jy-helicarrier-api.serviceId}", path = "/m/count")
public interface ApiCountService {}
全局拦截器
全局应用拦截器
如果我们需要对整个系统的的HTTP
请求执行统一的拦截处理,可以实现全局拦截器GlobalInterceptor
, 并配置成spring Bean
。
@Component
public class SourceGlobalInterceptor implements GlobalInterceptor {
@Autowired
private TestService testService;
@Override
public Response intercept(Chain chain) throws IOException {
Request request = chain.request();
Request newReq = request.newBuilder()
.addHeader("source", "test")
.build();
testService.test();
return chain.proceed(newReq);
}
}
全局网络拦截器
实现NetworkInterceptor
接口,并配置成spring Bean
。
调用适配器
Retrofit
可以通过CallAdapterFactory
将Call<T>
对象适配成接口方法的返回值类型。组件扩展了一些CallAdapterFactory
实现:
-
BodyCallAdapterFactory
-
- 同步执行
HTTP
请求,将响应体内容适配成方法的返回值类型。 - 任意方法返回值类型都可以使用
BodyCallAdapterFactory
,优先级最低。
-
ResponseCallAdapterFactory
-
- 同步执行
HTTP
请求,将响应体内容适配成Retrofit.Response<T>
返回。 - 只有方法返回值类型为
Retrofit.Response<T>
,才可以使用ResponseCallAdapterFactory
。
- 响应式编程相关
CallAdapterFactory
Retrofit
会根据方法返回值类型选择对应的CallAdapterFactory
执行适配处理,目前支持的返回值类型如下:
-
String
:将Response Body
适配成String
返回。 - 基础类型(
Long
/Integer
/Boolean
/Float
/Double
):将Response Body
适配成上述基础类型 - 任意
Java
类型:将Response Body
适配成对应的Java
对象返回 -
CompletableFuture<T>
: 将Response Body
适配成CompletableFuture<T>
对象返回 -
Void
: 不关注返回类型可以使用Void
-
Response<T>
: 将Response
适配成Response<T>
对象返回 -
Call<T>
: 不执行适配处理,直接返回Call<T>
对象 -
Mono<T>
:Project Reactor
响应式返回类型 -
Single<T>
:Rxjava
响应式返回类型(支持Rxjava2/Rxjava3
) -
Completable
:Rxjava
响应式返回类型,HTTP
请求没有响应体(支持Rxjava2/Rxjava3
)
@RetrofitClient(baseUrl = "${test.baseUrl}")
public interface HttpApi {
@POST("getString")
String getString(@Body Person person);
@GET("person")
Result<Person> getPerson(@Query("id") Long id);
@GET("person")
CompletableFuture<Result<Person>> getPersonCompletableFuture(@Query("id") Long id);
@POST("savePerson")
Void savePersonVoid(@Body Person person);
@GET("person")
Response<Result<Person>> getPersonResponse(@Query("id") Long id);
@GET("person")
Call<Result<Person>> getPersonCall(@Query("id") Long id);
@GET("person")
Mono<Result<Person>> monoPerson(@Query("id") Long id);
@GET("person")
Single<Result<Person>> singlePerson(@Query("id") Long id);
@GET("ping")
Completable ping();
}
可以通过继承CallAdapter.Factory
扩展CallAdapter
。
组件支持通过retrofit.global-call-adapter-factories
配置全局调用适配器工厂:
retrofit:
# 全局转换器工厂(组件扩展的`CallAdaptorFactory`工厂已经内置,这里请勿重复配置)
global-call-adapter-factories:
# ...
针对每个Java接口,还可以通过@RetrofitClient.callAdapterFactories
指定当前接口采用的CallAdapter.Factory
。
建议:将
CallAdapter.Factory
配置成Spring Bean
数据转码器
Retrofit
使用Converter
将@Body
注解的对象转换成Request Body
,将Response Body
转换成一个Java
对象,可以选用以下几种Converter
:
- Gson: com.squareup.Retrofit:converter-gson
- Jackson: com.squareup.Retrofit:converter-jackson
- Moshi: com.squareup.Retrofit:converter-moshi
- Protobuf: com.squareup.Retrofit:converter-protobuf
- Wire: com.squareup.Retrofit:converter-wire
- Simple XML: com.squareup.Retrofit:converter-simplexml
- JAXB: com.squareup.retrofit2:converter-jaxb
- fastJson:com.alibaba.fastjson.support.retrofit.Retrofit2ConverterFactory
组件支持通过retrofit.global-converter-factories
配置全局Converter.Factory
,默认的是retrofit2.converter.jackson.JacksonConverterFactory
。
如果需要修改Jackson
配置,自行覆盖JacksonConverterFactory
的bean
配置即可。
retrofit:
# 全局转换器工厂
global-converter-factories:
- com.github.lianjiatech.retrofit.spring.boot.core.BasicTypeConverterFactory
- retrofit2.converter.jackson.JacksonConverterFactory
针对每个Java
接口,还可以通过@RetrofitClient.converterFactories
指定当前接口采用的Converter.Factory
。
建议:将
Converter.Factory
配置成Spring Bean
。
元注解
@RetrofitClient
、@Retry
、@Logging
、@Resilience4jDegrade
等注解支持元注解、继承以及@AliasFor
。
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@Documented
@Inherited
@RetrofitClient(baseUrl = "${test.baseUrl}")
@Logging(logLevel = LogLevel.WARN)
@Retry(intervalMs = 200)
public @interface MyRetrofitClient {
@AliasFor(annotation = RetrofitClient.class, attribute = "converterFactories")
Class<? extends Converter.Factory>[] converterFactories() default {GsonConverterFactory.class};
@AliasFor(annotation = Logging.class, attribute = "logStrategy")
LogStrategy logStrategy() default LogStrategy.BODY;
}
其他功能示例
form参数
@FormUrlEncoded
@POST("token/verify")
Object tokenVerify(@Field("source") String source,@Field("signature") String signature,@Field("token") String token);
@FormUrlEncoded
@POST("message")
CompletableFuture<Object> sendMessage(@FieldMap Map<String, Object> param);
文件上传
创建MultipartBody.Part
// 对文件名使用URLEncoder进行编码
public ResponseEntity importTerminology(MultipartFile file){
String fileName=URLEncoder.encode(Objects.requireNonNull(file.getOriginalFilename()),"utf-8");
okhttp3.RequestBody requestBody=okhttp3.RequestBody.create(MediaType.parse("multipart/form-data"),file.getBytes());
MultipartBody.Part part=MultipartBody.Part.createFormData("file",fileName,requestBody);
apiService.upload(part);
return ok().build();
}
HTTP
上传接口
@POST("upload")
@Multipart
Void upload(@Part MultipartBody.Part file);
文件下载
HTTP
下载接口
@RetrofitClient(baseUrl = "https://.ljcdn.com/hc-picture/")
public interface DownloadApi {
@GET("{fileKey}")
Response<ResponseBody> download(@Path("fileKey") String fileKey);
}
HTTP
下载使用
@SpringBootTest(classes = RetrofitTestApplication.class)
@RunWith(SpringRunner.class)
public class DownloadTest {
@Autowired
DownloadApi downLoadApi;
@Test
public void download() throws Exception {
String fileKey = "6302d742-ebc8-4649-95cf-62ccf57a1add";
Response<ResponseBody> response = downLoadApi.download(fileKey);
ResponseBody responseBody = response.body();
// 二进制流
InputStream is = responseBody.byteStream();
// 具体如何处理二进制流,由业务自行控制。这里以写入文件为例
File tempDirectory = new File("temp");
if (!tempDirectory.exists()) {
tempDirectory.mkdir();
}
File file = new File(tempDirectory, UUID.randomUUID().toString());
if (!file.exists()) {
file.createNewFile();
}
FileOutputStream fos = new FileOutputStream(file);
byte[] b = new byte[1024];
int length;
while ((length = is.read(b)) > 0) {
fos.write(b, 0, length);
}
is.close();
fos.close();
}
}
动态URL
使用@url
注解可实现动态URL。此时,baseUrl
配置任意合法url即可。例如:http://github.com/
。运行时只会根据@Url
地址发起请求。
注意:
@url
必须放在方法参数的第一个位置,另外,@GET
、@POST
等注解上,不需要定义端点路径。
@GET
Map<String, Object> test3(@Url String url,@Query("name") String name);
DELETE
请求添加请求体
@HTTP(method = "DELETE", path = "/user/delete", hasBody = true)
GET
请求添加请求体
okhttp3
自身不支持GET
请求添加请求体,源码如下:
作者给出了具体原因,可以参考:
但是,如果实在需要这么做,可以使用:@HTTP(method = "get", path = "/user/get", hasBody = true)
,使用小写get
绕过上述限制。
文章转载自公众号: 码猿技术专栏
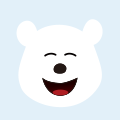