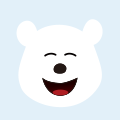
回复
xUtils 包含了orm, http(s), image, view注解, 特性强大, 方便扩展.
orm
: 高效稳定的orm工具, 使得http接口实现时更方便的支持cookie和缓存.http(s)
: 基于UrlConnection实现.image
: 有了http(s)
及其下载缓存的支持, image
模块的实现相当的简洁.view注解
: view注解模块仅仅400多行代码却灵活的支持了各种View注入和事件绑定.方式一:
通过library生成har包,添加har包到libs文件夹内
在entry的gradle内添加如下代码
implementation fileTree(dir: 'libs', include: ['*.jar', '*.har'])
方式二:
allprojects{
repositories{
mavenCentral()
}
}
implementation 'io.openharmony.tpc.thirdlib:xUtils3:1.0.0'
通过DevEco studio,并下载SDK 将项目中的build.gradle文件中dependencies→classpath版本改为对应的版本(即你的IDE新建项目中所用的版本)
// 在application的onCreate中初始化
@Override
public void onCreate() {
super.onCreate();
x.Ext.init(this);
x.Ext.setDebug(BuildConfig.DEBUG); // 是否输出debug日志, 开启debug会影响性能.
...
}
/**
* 1. 方法必须私有限定,
* 2. 方法参数形式必须和type对应的Listener接口一致.
* 3. 注解参数value支持数组: value={id1, id2, id3}
* 4. 其它参数说明见{@link org.xutils.event.annotation.Event}类的说明.
**/
@Event(value = {ResourceTable.Id_btn_viewbind})
private void onTest1Click(Component view) {
...
}
/**
* 数据库操作示例
*/
public class DbAbilitySlice extends AbilitySlice {
@ViewInject(ResourceTable.Id_btn_add)
Button btnDbAdd;
@ViewInject(ResourceTable.Id_btn_del)
Button btnDbDel;
@ViewInject(ResourceTable.Id_btn_update)
Button btnDbUpdate;
@ViewInject(ResourceTable.Id_btn_select)
Button btnDbSelect;
@ViewInject(ResourceTable.Id_text_show)
Text txtShow;
DbManager.DaoConfig daoConfig;
@Override
public void onStart(Intent intent) {
super.onStart(intent);
super.setUIContent(ResourceTable.Layout_ability_db);
x.view().inject(this);
daoConfig = new DbManager.DaoConfig()
.setDbName("testaa.db")
// 不设置dbDir时, 默认存储在app的私有目录.
.setDbDir(new File("/sdcard")) // "sdcard"的写法并非最佳实践, 这里为了简单, 先这样写了.
.setDbVersion(2)
.setDbOpenListener(new DbManager.DbOpenListener() {
@Override
public void onDbOpened(DbManager db) {
// 开启WAL, 对写入加速提升巨大
// db.getDatabase().enableWriteAheadLogging();
}
})
.setDbUpgradeListener(new DbManager.DbUpgradeListener() {
@Override
public void onUpgrade(DbManager db, int oldVersion, int newVersion) {
// TODO: ...
// db.addColumn(...);
// db.dropTable(...);
// ...
// or
// db.dropDb();
}
});
}
@Event(value = {ResourceTable.Id_btn_add, ResourceTable.Id_btn_del, ResourceTable.Id_btn_update, ResourceTable.Id_btn_select})
private void onTest1Click(Component view) {
if (view.getId() == ResourceTable.Id_btn_add) {
dbSave();
}
if (view.getId() == ResourceTable.Id_btn_del) {
dbDel();
}
if (view.getId() == ResourceTable.Id_btn_update) {
dbUpdate();
}
if (view.getId() == ResourceTable.Id_btn_select) {
dbSelect();
}
}
/**
* 添加
*/
private void dbSave() {
try {
btnDbAdd.setText("添加");
DbManager db = x.getDb(daoConfig);
ArrayList<ChildInfo> childInfos = new ArrayList<>();
childInfos.add(new ChildInfo("zhangsan"));
childInfos.add(new ChildInfo("lisi"));
childInfos.add(new ChildInfo("wangwu"));
childInfos.add(new ChildInfo("zhaoliu"));
childInfos.add(new ChildInfo("qianqi"));
childInfos.add(new ChildInfo("sunba"));
db.save(childInfos);
btnDbAdd.setText("添加成功");
} catch (Exception e) {
}
}
/**
* 删除
*/
private void dbDel() {
try {
btnDbDel.setText("删除");
DbManager db = x.getDb(daoConfig);
db.delete(ChildInfo.class);
btnDbDel.setText("删除成功");
} catch (Exception e) {
}
}
/**
* 修改
*/
private void dbUpdate() {
try {
btnDbUpdate.setText("修改");
DbManager db = x.getDb(daoConfig);
ChildInfo first = db.findFirst(ChildInfo.class);
first.setcName("zhansan2");
db.update(first, "c_name"); //c_name:表中的字段名
btnDbUpdate.setText("修改成功");
} catch (Exception e) {
}
}
/**
* 查询
*/
private void dbSelect() {
try {
btnDbSelect.setText("查询");
DbManager db = x.getDb(daoConfig);
String result = "";
// ChildInfo first = db.findFirst(ChildInfo.class);
// List<ChildInfo> all = db.selector(ChildInfo.class).where("id", ">", 0).and("id", "<", 4).findAll();
List<ChildInfo> all = db.selector(ChildInfo.class).findAll();
for (ChildInfo childInfo : all) {
result += childInfo.toString()+"\n";
}
btnDbSelect.setText("查询成功");
txtShow.setText("查询结果:"+result);
} catch (Exception e) {
}
}
@Event(value = {ResourceTable.Id_btn_http_get})
private void onTest2Click(View view) {
RequestParams params = new RequestParams("https://www.baidu.com/s");
// params.setSslSocketFactory(...); // 如果需要自定义SSL
params.addQueryStringParameter("wd", "xUtils");
x.http().get(params, new Callback.CommonCallback<String>() {
@Override
public void onSuccess(String result) {
Toast.makeText(x.app(), result, Toast.LENGTH_LONG).show();
}
@Override
public void onError(Throwable ex, boolean isOnCallback) {
Toast.makeText(x.app(), ex.getMessage(), Toast.LENGTH_LONG).show();
}
@Override
public void onCancelled(CancelledException cex) {
Toast.makeText(x.app(), "cancelled", Toast.LENGTH_LONG).show();
}
@Override
public void onFinished() {
}
});
}
x.image().bind(imageView, url, imageOptions, new Callback.CommonCallback<PixelMap>() {...});
Copyright 2014-2015 wyouflf
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.