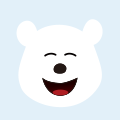
回复
1.在项目根目录下的build.gradle文件中,
allprojects {
repositories {
maven {
url 'https://s01.oss.sonatype.org/content/repositories/release/'
}
}
}
2.在entry模块的build.gradle文件中,
dependencies {
implementation 'com.gitee.chinasoft_ohos:Fetch:0.0.1-SNAPSHOT'
......
}
在sdk6,DevEco Studio 2.2 Beta1下项目可直接运行 如无法运行,删除项目.gradle,.idea,build,gradle,build.gradle文件, 并依据自己的版本创建新项目,将新项目的对应文件复制到根目录下
1 添加权限
"name": "ohos.permission.WRITE_USER_STORAGE",
"name": "ohos.permission.READ_USER_STORAGE",
2 获取 Fetch 的实例并请求下载
FetchConfiguration fetchConfiguration = new FetchConfiguration.Builder(this)
.setDownloadConcurrentLimit(3)
.build();
Fetch fetch = Fetch.Impl.getInstance(fetchConfiguration);
String url = "http:www.example.com/test.txt";
String file = "/downloads/test.txt";
final Request request = new Request(url, file);
request.setPriority(Priority.HIGH);
request.setNetworkType(NetworkType.ALL);
request.addHeader("clientKey", "SD78DF93_3947&MVNGHE1WONG");
fetch.enqueue(request, updatedRequest -> {
//Request was successfully enqueued for download.
}, error -> {
//An error occurred enqueuing the request.
});
3 使用 Fetch 可以非常轻松地跟踪下载进度和状态。只需将 FetchListener 添加到您的 Fetch 实例,只要下载的状态或进度发生变化,就会通知侦听器。
FetchListener fetchListener = new FetchListener() {
@Override
public void onQueued(@NotNull Download download, boolean waitingOnNetwork) {
if (request.getId() == download.getId()) {
showDownloadInList(download);
}
}
@Override
public void onCompleted(@NotNull Download download) {
}
@Override
public void onError(@NotNull Download download) {
Error error = download.getError();
}
@Override
public void onProgress(@NotNull Download download, long etaInMilliSeconds, long downloadedBytesPerSecond) {
if (request.getId() == download.getId()) {
updateDownload(download, etaInMilliSeconds);
}
int progress = download.getProgress();
}
@Override
public void onPaused(@NotNull Download download) {
}
@Override
public void onResumed(@NotNull Download download) {
}
@Override
public void onCancelled(@NotNull Download download) {
}
@Override
public void onRemoved(@NotNull Download download) {
}
@Override
public void onDeleted(@NotNull Download download) {
}
};
fetch.addListener(fetchListener);
//Remove listener when done.
fetch.removeListener(fetchListener);
4 Fetch 支持使用请求的 id 暂停和恢复下载。请求的 id 是将请求映射到获取下载的唯一标识符。Fetch 返回的下载将具有与开始下载的请求 ID 匹配的 ID。
Request request1 = new Request(url, file);
Request request2 = new Request(url2, file2);
fetch.pause(request1.getId());
...
fetch.resume(request2.getId());
5 您可以通过多种方式查询 Fetch 以获取下载信息。
//Query all downloads
fetch.getDownloads(new Func<List<? extends Download>>() {
@Override
public void call(List<? extends Download> downloads) {
//Access all downloads here
}
});
//Get all downloads with a status
fetch.getDownloadsWithStatus(Status.DOWNLOADING, new Func<List<? extends Download>>() {
@Override
public void call(List<? extends Download> downloads) {
//Access downloads that are downloading
}
});
// You can also access grouped downloads
int groupId = 52687447745;
fetch.getDownloadsInGroup(groupId, new Func<List<? extends Download>>() {
@Override
public void call(List<? extends Download> downloads) {
//Access grouped downloads
}
});
6 完成 Fetch 实例后,只需将其释放即可。
//do work
fetch.close();
//do more work
设置 OkHttp 下载器以供 Fetch 使用。
OkHttpClient okHttpClient = new OkHttpClient.Builder().build();
FetchConfiguration fetchConfiguration = new FetchConfiguration.Builder(this)
.setDownloadConcurrentLimit(10)
.setHttpDownloader(new OkHttpDownloader(okHttpClient))
.build();
Fetch fetch = Fetch.Impl.getInstance(fetchConfiguration);
RxFetch 使用 rxJava2 函数方法使下载请求和查询下载变得非常容易。
FetchConfiguration fetchConfiguration = new FetchConfiguration.Builder(this).build();
Rxfetch rxFetch = RxFetch.Impl.getInstance(fetchConfiguration);
rxFetch.getDownloads()
.asFlowable()
.subscribe(new Consumer<List<Download>>() {
@Override
public void accept(List<Download> downloads) throws Exception {
//Access results
}
}, new Consumer<Throwable>() {
@Override
public void accept(Throwable throwable) throws Exception {
//An error occurred
final Error error = FetchErrorUtils.getErrorFromThrowable(throwable);
}
});
启动一个 FetchFileServer 实例并添加它可以为连接的客户端提供服务的资源文件。
FetchFileServer fetchFileServer = new FetchFileServer.Builder(this)
.build();
fetchFileServer.start(); //listen for client connections
File file = new File("/downloads/testfile.txt");
FileResource fileResource = new FileResource();
fileResource.setFile(file.getAbsolutePath());
fileResource.setLength(file.length());
fileResource.setName("testfile.txt");
fileResource.setId(UUID.randomUUID().hashCode());
fetchFileServer.addFileResource(fileResource);
使用 Fetch 从 FetchFileServer 下载文件很容易。
FetchConfiguration fetchConfiguration = new FetchConfiguration.Builder(this)
.setFileServerDownloader(new FetchFileServerDownloader()) //have to set the file server downloader
.build();
fetch = Fetch.Impl.getInstance(fetchConfiguration);
fetch.addListener(fetchListener);
String file = "/downloads/sample.txt";
String url = new FetchFileServerUrlBuilder()
.setHostInetAddress("127.0.0.1", 6886) //file server ip and port
.setFileResourceIdentifier("testfile.txt") //file resource name or id
.create();
Request request = new Request(url, file);
fetch.enqueue(request, request1 -> {
//Request enqueued for download
}, error -> {
//Error while enqueuing download
});
private FetchListener fetchListener = new AbstractFetchListener() {
@Override
public void onProgress(@NotNull Download download, long etaInMilliSeconds, long downloadedBytesPerSecond) {
super.onProgress(download, etaInMilliSeconds, downloadedBytesPerSecond);
Log.d("TestActivity", "Progress: " + download.getProgress());
}
@Override
public void onError(@NotNull Download download) {
super.onError(download);
Log.d("TestActivity", "Error: " + download.getError().toString());
}
@Override
public void onCompleted(@NotNull Download download) {
super.onCompleted(download);
Log.d("TestActivity", "Completed ");
}
};
运行迁移器。
if (!didMigrationRun()) {
//Migration has to run on a background thread
new Thread(new Runnable() {
@Override
public void run() {
try {
final List<DownloadTransferPair> transferredDownloads = FetchMigrator.migrateFromV1toV2(getApplicationContext(), APP_FETCH_NAMESPACE);
//TODO: update external references of ids
for (DownloadTransferPair transferredDownload : transferredDownloads) {
Log.d("newDownload", transferredDownload.getNewDownload().toString());
Log.d("oldId in Fetch v1", transferredDownload.getOldID() + "");
}
FetchMigrator.deleteFetchV1Database(getApplicationContext());
setMigrationDidRun(true);
//Setup and Run Fetch2 after the migration
} catch (SQLException e) {
e.printStackTrace();
}
}
}).start();
} else {
//Setup and Run Fetch2 normally
}
CodeCheck代码测试无异常
CloudTest代码测试无异常
病毒安全检测通过
当前版本demo功能与原组件基本无差异
Copyright (C) 2017 Tonyo Francis.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.