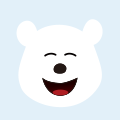
HarmonyOS Developer 常见组件开发指导
button开发指导
button是按钮组件,其类型包括胶囊按钮、圆形按钮、文本按钮、弧形按钮、下载按钮。具体用法请参考button API。
创建button组件
在pages/index目录下的hml文件中创建一个button组件。
设置button类型
通过设置button的type属性来选择按钮类型,如定义button为圆形按钮、文本按钮等。
说明
- button组件使用的icon图标如果来自云端路径,需要添加网络访问权限 ohos.permission.INTERNET。
如果需要添加ohos.permission.INTERNET权限,则在resources文件夹下的config.json文件里进行权限配置。
显示下载进度
为button组件添加progress方法,来实时显示下载进度条的进度。
说明
setProgress方法只支持button的类型为download。
场景示例
在本场景中,开发者可根据输入的文本内容进行button类型切换。
picker开发指导
picker是滑动选择器组件,类型支持普通选择器、日期选择器、时间选择器、时间日期选择器和多列文本选择器。具体用法请参考picker API。
创建picker组件
在pages/index目录下的hml文件中创建一个picker组件。
设置picker类型
通过设置picker的type属性来选择滑动选择器类型,如定义picker为日期选择器。
说明
普通选择器设置取值范围时,需要使用数据绑定的方式。
设置时间展现格式
picker的hours属性定义时间的展现格式,可选类型有12小时制和24小时制。
说明
- hours属性为12:按照12小时制显示,用上午和下午进行区分;
- hours属性为24:按照24小时制显示。
添加响应事件
对picker添加change和cancel事件,来对选择的内容进行确定和取消。
场景示例
在本场景中,开发者可以自定义填写当前的健康情况来进行打卡。
image开发指导
image是图片组件,用来渲染展示图片。具体用法请参考image API。
创建image组件
在pages/index目录下的hml文件中创建一个image组件。
设置image样式
通过设置width、height和object-fit属性定义图片的宽、高和缩放样式。
加载图片
图片成功加载时触发complete事件,返回加载的图源尺寸。加载失败则触发error事件,打印图片加载失败。
场景示例
在本场景中,开发者长按图片后将慢慢隐藏图片,当完全隐藏后再重新显示原始图片。定时器setInterval每隔一段时间改变图片透明度,实现慢慢隐藏的效果,当透明度为0时清除定时器,设置透明度为1。
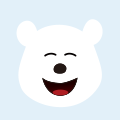