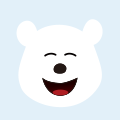
HarmonyOS API:@ohos.notificationManager (模块)
版本:v3.1 Beta
@ohos.notificationManager (NotificationManager模块)
更新时间: 2023-02-17 09:19
本模块提供通知管理的能力,包括发布、取消发布通知,创建、获取、移除通知通道,获取通知的使能状态、角标使能状态,获取通知的相关信息等。
说明
本模块首批接口从API version 9开始支持。后续版本的新增接口,采用上角标单独标记接口的起始版本。
导入模块
Notification.publish
publish(request: NotificationRequest, callback: AsyncCallback<void>): void
发布通知(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
request | 是 | 设置要发布通知内容的NotificationRequest对象。 | |
callback | AsyncCallback<void> | 是 | 被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600004 | Notification is not enabled. |
1600005 | Notification slot is not enabled. |
1600009 | Over max number notifications per second. |
示例:
Notification.publish
publish(request: NotificationRequest): Promise<void>
发布通知(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
request | 是 | 设置要发布通知内容的NotificationRequest对象。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600004 | Notification is not enabled. |
1600005 | Notification slot is not enabled. |
1600009 | Over max number notifications per second. |
示例:
Notification.cancel
cancel(id: number, label: string, callback: AsyncCallback<void>): void
取消与指定id和label相匹配的已发布通知(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
id | number | 是 | 通知ID。 |
label | string | 是 | 通知标签。 |
callback | AsyncCallback<void> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600007 | The notification is not exist. |
示例:
Notification.cancel
cancel(id: number, label?: string): Promise<void>
取消与指定id相匹配的已发布通知,label可以指定也可以不指定(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
id | number | 是 | 通知ID。 |
label | string | 否 | 通知标签。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600007 | The notification is not exist. |
示例:
Notification.cancel
cancel(id: number, callback: AsyncCallback<void>): void
取消与指定id相匹配的已发布通知(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
id | number | 是 | 通知ID。 |
callback | AsyncCallback<void> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600007 | The notification is not exist. |
示例:
Notification.cancelAll
cancelAll(callback: AsyncCallback<void>): void
取消所有已发布的通知(callback形式)。
系统能力:SystemCapability.Notification.Notification
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
参数:
名称 | 类型 | 必填 | 描述 |
callback | AsyncCallback<void> | 是 | 表示被指定的回调方法。 |
示例:
Notification.cancelAll
cancelAll(): Promise<void>
取消所有已发布的通知(Promise形式)。
系统能力:SystemCapability.Notification.Notification
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.addSlot
addSlot(type: SlotType, callback: AsyncCallback<void>): void
创建通知通道(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
type | 是 | 要创建的通知通道的类型。 | |
callback | AsyncCallback<void> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.addSlot
addSlot(type: SlotType): Promise<void>
创建通知通道(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
type | 是 | 要创建的通知通道的类型。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getSlot
getSlot(slotType: SlotType, callback: AsyncCallback<NotificationSlot>): void
获取一个通知通道(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
slotType | 是 | 通知渠道类型,目前分为社交通信、服务提醒、内容咨询和其他类型。 | |
callback | AsyncCallback<NotificationSlot> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getSlot
getSlot(slotType: SlotType): Promise<NotificationSlot>
获取一个通知通道(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
slotType | 是 | 通知渠道类型,目前分为社交通信、服务提醒、内容咨询和其他类型。 |
返回值:
类型 | 说明 |
Promise<NotificationSlot> | 以Promise形式返回获取一个通知通道。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getSlots
getSlots(callback: AsyncCallback<Array<NotificationSlot>>): void
获取此应用程序的所有通知通道(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
callback | AsyncCallback<Array<NotificationSlot>> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getSlots
getSlots(): Promise<Array<NotificationSlot>>
获取此应用程序的所有通知通道(Promise形式)。
系统能力:SystemCapability.Notification.Notification
返回值:
类型 | 说明 |
Promise<Array<NotificationSlot>> | 以Promise形式返回获取此应用程序的所有通知通道的结果。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.removeSlot
removeSlot(slotType: SlotType, callback: AsyncCallback<void>): void
根据通知通道类型删除创建的通知通道(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
slotType | 是 | 通知渠道类型,目前分为社交通信、服务提醒、内容咨询和其他类型。 | |
callback | AsyncCallback<void> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.removeSlot
removeSlot(slotType: SlotType): Promise<void>
根据通知通道类型删除创建的通知通道(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
slotType | 是 | 通知渠道类型,目前分为社交通信、服务提醒、内容咨询和其他类型。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.removeAllSlots
removeAllSlots(callback: AsyncCallback<void>): void
删除所有通知通道(callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
callback | AsyncCallback<void> | 是 | 表示被指定的回调方法。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.removeAllSlots
removeAllSlots(): Promise<void>
删除所有通知通道(Promise形式)。
系统能力:SystemCapability.Notification.Notification
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getActiveNotificationCount
getActiveNotificationCount(callback: AsyncCallback<number>): void
获取当前应用的活动通知数(Callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
callback | AsyncCallback<number> | 是 | 获取活动通知数回调函数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getActiveNotificationCount
getActiveNotificationCount(): Promise<number>
获取当前应用的活动通知数(Promise形式)。
系统能力:SystemCapability.Notification.Notification
返回值:
类型 | 说明 |
Promise<number> | 以Promise形式返回获取当前应用的活动通知数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getActiveNotifications
getActiveNotifications(callback: AsyncCallback<Array<NotificationRequest>>): void
获取当前应用的活动通知(Callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
callback | AsyncCallback<Array<NotificationRequest>> | 是 | 获取当前应用的活动通知回调函数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.getActiveNotifications
getActiveNotifications(): Promise<Array<NotificationRequest>>
获取当前应用的活动通知(Promise形式)。
系统能力:SystemCapability.Notification.Notification
返回值:
类型 | 说明 |
Promise<Array<NotificationRequest>> | 以Promise形式返回获取当前应用的活动通知。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.cancelGroup
cancelGroup(groupName: string, callback: AsyncCallback<void>): void
取消本应用指定组通知(Callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
groupName | string | 是 | 指定通知组名称。 |
callback | AsyncCallback<void> | 是 | 取消本应用指定组通知回调函数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.cancelGroup
cancelGroup(groupName: string): Promise<void>
取消本应用指定组通知(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
名称 | 类型 | 必填 | 描述 |
groupName | string | 是 | 指定通知组名称。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.isSupportTemplate
isSupportTemplate(templateName: string, callback: AsyncCallback<boolean>): void
查询模板是否存在(Callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
参数名 | 类型 | 必填 | 说明 |
templateName | string | 是 | 模板名称。 |
callback | AsyncCallback<boolean> | 是 | 查询模板是否存在的回调函数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600011 | Read template config failed. |
示例:
Notification.isSupportTemplate
isSupportTemplate(templateName: string): Promise<boolean>
查询模板是否存在(Promise形式)。
系统能力:SystemCapability.Notification.Notification
参数:
参数名 | 类型 | 必填 | 说明 |
templateName | string | 是 | 模板名称。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise方式返回模板是否存在的结果。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600011 | Read template config failed. |
示例:
Notification.requestEnableNotification
requestEnableNotification(callback: AsyncCallback<void>): void
应用请求通知使能(Callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<void> | 是 | 应用请求通知使能的回调函数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.requestEnableNotification
requestEnableNotification(): Promise<void>
应用请求通知使能(Promise形式)。
系统能力:SystemCapability.Notification.Notification
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
示例:
Notification.isDistributedEnabled
isDistributedEnabled(callback: AsyncCallback<boolean>): void
获取设备是否支持分布式通知(Callback形式)。
系统能力:SystemCapability.Notification.Notification
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<boolean> | 是 | 设备是否支持分布式通知的回调函数。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600010 | Distributed operation failed. |
示例:
Notification.isDistributedEnabled
isDistributedEnabled(): Promise<boolean>
获取设备是否支持分布式通知(Promise形式)。
系统能力:SystemCapability.Notification.Notification
返回值:
类型 | 说明 |
Promise<boolean> | Promise方式返回设备是否支持分布式通知的结果。 true 支持。 false 不支持。 |
错误码:
错误码ID | 错误信息 |
1600001 | Internal error. |
1600002 | Marshalling or unmarshalling error. |
1600003 | Failed to connect service. |
1600010 | Distributed operation failed. |
示例:
ContentType
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 值 | 说明 |
NOTIFICATION_CONTENT_BASIC_TEXT | NOTIFICATION_CONTENT_BASIC_TEXT | 普通类型通知。 |
NOTIFICATION_CONTENT_LONG_TEXT | NOTIFICATION_CONTENT_LONG_TEXT | 长文本类型通知。 |
NOTIFICATION_CONTENT_PICTURE | NOTIFICATION_CONTENT_PICTURE | 图片类型通知。 |
NOTIFICATION_CONTENT_CONVERSATION | NOTIFICATION_CONTENT_CONVERSATION | 社交类型通知。 |
NOTIFICATION_CONTENT_MULTILINE | NOTIFICATION_CONTENT_MULTILINE | 多行文本类型通知。 |
SlotLevel
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 值 | 说明 |
LEVEL_NONE | 0 | 表示关闭通知功能。 |
LEVEL_MIN | 1 | 表示通知功能已启用,但状态栏中不显示通知图标,且没有横幅或提示音。 |
LEVEL_LOW | 2 | 表示通知功能已启用,且状态栏中显示通知图标,但没有横幅或提示音。 |
LEVEL_DEFAULT | 3 | 表示通知功能已启用,状态栏中显示通知图标,没有横幅但有提示音。 |
LEVEL_HIGH | 4 | 表示通知功能已启用,状态栏中显示通知图标,有横幅和提示音。 |
BundleOption
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
bundle | 是 | 是 | string | 包名。 |
uid | 是 | 是 | number | 用户id。 |
SlotType
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 值 | 说明 |
UNKNOWN_TYPE | 0 | 未知类型。 |
SOCIAL_COMMUNICATION | 1 | 社交类型。 |
SERVICE_INFORMATION | 2 | 服务类型。 |
CONTENT_INFORMATION | 3 | 内容类型。 |
OTHER_TYPES | 0xFFFF | 其他类型。 |
NotificationActionButton
描述通知中显示的操作按钮。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 说明 |
title | 是 | 是 | string | 按钮标题。 |
wantAgent | 是 | 是 | WantAgent | 点击按钮时触发的WantAgent。 |
extras | 是 | 是 | { [key: string]: any } | 按钮扩展信息。 |
userInput | 是 | 是 | 用户输入对象实例。 |
NotificationBasicContent
描述普通文本通知。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
title | 是 | 是 | string | 通知标题。 |
text | 是 | 是 | string | 通知内容。 |
additionalText | 是 | 是 | string | 通知次要内容,是对通知内容的补充。 |
NotificationLongTextContent
描述长文本通知。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
title | 是 | 是 | string | 通知标题。 |
text | 是 | 是 | string | 通知内容。 |
additionalText | 是 | 是 | string | 通知次要内容,是对通知内容的补充。 |
longText | 是 | 是 | string | 通知的长文本。 |
briefText | 是 | 是 | string | 通知概要内容,是对通知内容的总结。 |
expandedTitle | 是 | 是 | string | 通知展开时的标题。 |
NotificationMultiLineContent
描述多行文本通知。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
title | 是 | 是 | string | 通知标题。 |
text | 是 | 是 | string | 通知内容。 |
additionalText | 是 | 是 | string | 通知次要内容,是对通知内容的补充。 |
briefText | 是 | 是 | string | 通知概要内容,是对通知内容的总结。 |
longTitle | 是 | 是 | string | 通知展开时的标题。 |
lines | 是 | 是 | Array<string> | 通知的多行文本。 |
NotificationPictureContent
描述附有图片的通知。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
title | 是 | 是 | string | 通知标题。 |
text | 是 | 是 | string | 通知内容。 |
additionalText | 是 | 是 | string | 通知次要内容,是对通知内容的补充。 |
briefText | 是 | 是 | string | 通知概要内容,是对通知内容的总结。 |
expandedTitle | 是 | 是 | string | 通知展开时的标题。 |
picture | 是 | 是 | image.PixelMap | 通知的图片内容。 |
NotificationContent
描述通知类型。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
contentType | 是 | 是 | 通知内容类型。 | |
normal | 是 | 是 | 基本类型通知内容。 | |
longText | 是 | 是 | 长文本类型通知内容。 | |
multiLine | 是 | 是 | 多行类型通知内容。 | |
picture | 是 | 是 | 图片类型通知内容。 |
NotificationRequest
描述通知的请求。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 说明 |
content | 是 | 是 | 通知内容。 | |
id | 是 | 是 | number | 通知ID。 |
slotType | 是 | 是 | 通道类型。 | |
isOngoing | 是 | 是 | boolean | 是否进行时通知。 |
isUnremovable | 是 | 是 | boolean | 是否可移除。 |
deliveryTime | 是 | 是 | number | 通知发送时间。 |
tapDismissed | 是 | 是 | boolean | 通知是否自动清除。 |
autoDeletedTime | 是 | 是 | number | 自动清除的时间。 |
wantAgent | 是 | 是 | WantAgent | WantAgent封装了应用的行为意图,点击通知时触发该行为。 |
extraInfo | 是 | 是 | {[key: string]: any} | 扩展参数。 |
color | 是 | 是 | number | 通知背景颜色。暂不支持。 |
colorEnabled | 是 | 是 | boolean | 通知背景颜色是否使能。暂不支持。 |
isAlertOnce | 是 | 是 | boolean | 设置是否仅有一次此通知警报。 |
isStopwatch | 是 | 是 | boolean | 是否显示已用时间。 |
isCountDown | 是 | 是 | boolean | 是否显示倒计时时间。 |
isFloatingIcon | 是 | 是 | boolean | 是否显示状态栏图标。 |
label | 是 | 是 | string | 通知标签。 |
badgeIconStyle | 是 | 是 | number | 通知角标类型。 |
showDeliveryTime | 是 | 是 | boolean | 是否显示分发时间。 |
actionButtons | 是 | 是 | Array<NotificationActionButton> | 通知按钮,最多两个按钮。 |
smallIcon | 是 | 是 | PixelMap | 通知小图标。 |
largeIcon | 是 | 是 | PixelMap | 通知大图标。 |
creatorBundleName | 是 | 否 | string | 创建通知的包名。 |
creatorUid | 是 | 否 | number | 创建通知的UID。 |
creatorPid | 是 | 否 | number | 创建通知的PID。 |
creatorUserId | 是 | 否 | number | 创建通知的UserId。 |
hashCode | 是 | 否 | string | 通知唯一标识。 |
groupName | 是 | 是 | string | 组通知名称。 |
template | 是 | 是 | 通知模板。 | |
distributedOption | 是 | 是 | 分布式通知的选项。 | |
notificationFlags | 是 | 否 | NotificationFlags | 获取NotificationFlags。 |
removalWantAgent | 是 | 是 | WantAgent | 当移除通知时,通知将被重定向到的WantAgent实例。 |
badgeNumber | 是 | 是 | number | 应用程序图标上显示的通知数。 |
DistributedOptions
描述分布式选项。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
isDistributed | 是 | 是 | boolean | 是否为分布式通知。 |
supportDisplayDevices | 是 | 是 | Array<string> | 可以同步通知到的设备类型。 |
supportOperateDevices | 是 | 是 | Array<string> | 可以打开通知的设备。 |
NotificationSlot
描述通知槽
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
type | 是 | 是 | 通道类型。 | |
level | 是 | 是 | number | 通知级别,不设置则根据通知渠道类型有默认值。 |
desc | 是 | 是 | string | 通知渠道描述信息。 |
badgeFlag | 是 | 是 | boolean | 是否显示角标。 |
bypassDnd | 是 | 是 | boolean | 置是否在系统中绕过免打扰模式。 |
lockscreenVisibility | 是 | 是 | number | 在锁定屏幕上显示通知的模式。 |
vibrationEnabled | 是 | 是 | boolean | 是否可振动。 |
sound | 是 | 是 | string | 通知提示音。 |
lightEnabled | 是 | 是 | boolean | 是否闪灯。 |
lightColor | 是 | 是 | number | 通知灯颜色。 |
vibrationValues | 是 | 是 | Array<number> | 通知振动样式。 |
enabled9+ | 是 | 否 | boolean | 此通知插槽中的启停状态。 |
NotificationTemplate
通知模板。
系统能力:以下各项对应的系统能力均为SystemCapability.Notification.Notification
名称 | 参数类型 | 可读 | 可写 | 说明 |
name | string | 是 | 是 | 模板名称。 |
data | {[key:string]: Object} | 是 | 是 | 模板数据。 |
NotificationUserInput
保存用户输入的通知消息。
系统能力:SystemCapability.Notification.Notification
名称 | 可读 | 可写 | 类型 | 描述 |
inputKey | 是 | 是 | string | 用户输入时用于标识此输入的key。 |
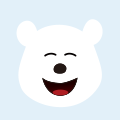