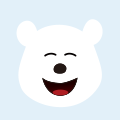
HarmonyOS API:@ohos.multimedia.image (图片处理)
版本:v3.1 Beta
@ohos.multimedia.image (图片处理)
更新时间: 2023-02-17 09:19
本模块提供图片处理效果,包括通过属性创建PixelMap、读取图像像素数据、读取区域内的图片数据等。
说明
本模块首批接口从API version 6开始支持。后续版本的新增接口,采用上角标单独标记接口的起始版本。
导入模块
import image from '@ohos.multimedia.image';
image.createPixelMap8+
createPixelMap(colors: ArrayBuffer, options: InitializationOptions): Promise<PixelMap>
通过属性创建PixelMap,默认采用BGRA_8888格式处理数据,通过Promise返回结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
colors | ArrayBuffer | 是 | BGRA_8888格式的颜色数组。 |
options | 是 | 创建像素的属性,包括透明度,尺寸,缩略值,像素格式和是否可编辑。 |
返回值:
类型 | 说明 |
Promise<PixelMap> | 返回Pixelmap。 当创建的pixelmap大小超过原图大小时,返回原图pixelmap大小。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
let opts = { editable: true, pixelFormat: 3, size: { height: 4, width: 6 } }
image.createPixelMap(color, opts).then((pixelmap) => {
console.log('Succeeded in creating pixelmap.');
}).catch(error => {
console.log('Failed to create pixelmap.');
})
image.createPixelMap8+
createPixelMap(colors: ArrayBuffer, options: InitializationOptions, callback: AsyncCallback<PixelMap>): void
通过属性创建PixelMap,默认采用BGRA_8888格式处理数据,通过回调函数返回结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
colors | ArrayBuffer | 是 | BGRA_8888格式的颜色数组。 |
options | 是 | 属性。 | |
callback | AsyncCallback<PixelMap> | 是 | 通过回调返回PixelMap对象。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
let opts = { editable: true, pixelFormat: 3, size: { height: 4, width: 6 } }
image.createPixelMap(color, opts, (error, pixelmap) => {
if(error) {
console.log('Failed to create pixelmap.');
} else {
console.log('Succeeded in creating pixelmap.');
}
})
PixelMap7+
图像像素类,用于读取或写入图像数据以及获取图像信息。在调用PixelMap的方法前,需要先通过createPixelMap创建一个PixelMap实例。
属性
系统能力: SystemCapability.Multimedia.Image.Core
名称 | 类型 | 可读 | 可写 | 说明 |
isEditable | boolean | 是 | 否 | 设定是否图像像素可被编辑。 |
readPixelsToBuffer7+
readPixelsToBuffer(dst: ArrayBuffer): Promise<void>
读取图像像素数据,结果写入ArrayBuffer里,使用Promise形式返回。指定BGRA_8888格式创建pixelmap,读取的像素数据与原数据保持一致。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
dst | ArrayBuffer | 是 | 缓冲区,函数执行结束后获取的图像像素数据写入到该内存区域内。缓冲区大小由getPixelBytesNumber接口获取。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,用于获取结果,失败时返回错误信息。 |
示例:
const readBuffer = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
pixelmap.readPixelsToBuffer(readBuffer).then(() => {
console.log('Succeeded in reading image pixel data.'); //符合条件则进入
}).catch(error => {
console.log('Failed to read image pixel data.'); //不符合条件则进入
})
readPixelsToBuffer7+
readPixelsToBuffer(dst: ArrayBuffer, callback: AsyncCallback<void>): void
读取图像像素数据,结果写入ArrayBuffer里,使用callback形式返回。指定BGRA_8888格式创建pixelmap,读取的像素数据与原数据保持一致。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
dst | ArrayBuffer | 是 | 缓冲区,函数执行结束后获取的图像像素数据写入到该内存区域内。缓冲区大小由getPixelBytesNumber接口获取。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
const readBuffer = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
pixelmap.readPixelsToBuffer(readBuffer, (err, res) => {
if(err) {
console.log('Failed to read image pixel data.'); //不符合条件则进入
} else {
console.log('Succeeded in reading image pixel data.'); //符合条件则进入
}
})
readPixels7+
readPixels(area: PositionArea): Promise<void>
读取区域内的图片数据,使用Promise形式返回读取结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
area | 是 | 区域大小,根据区域读取。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,用于获取读取结果,失败时返回错误信息。 |
示例:
const area = {
pixels: new ArrayBuffer(8),
offset: 0,
stride: 8,
region: { size: { height: 1, width: 2 }, x: 0, y: 0 }
}
pixelmap.readPixels(area).then(() => {
console.log('Succeeded in reading the image data in the area.'); //符合条件则进入
}).catch(error => {
console.log('Failed to read the image data in the area.'); //不符合条件则进入
})
readPixels7+
readPixels(area: PositionArea, callback: AsyncCallback<void>): void
读取区域内的图片数据,使用callback形式返回读取结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
area | 是 | 区域大小,根据区域读取。 | |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
let opts = { editable: true, pixelFormat: 3, size: { height: 4, width: 6 } }
image.createPixelMap(color, opts, (err, pixelmap) => {
if(pixelmap == undefined){
console.info('createPixelMap failed.');
} else {
const area = { pixels: new ArrayBuffer(8),
offset: 0,
stride: 8,
region: { size: { height: 1, width: 2 }, x: 0, y: 0 }};
pixelmap.readPixels(area, () => {
console.info('readPixels success');
})
}
})
writePixels7+
writePixels(area: PositionArea): Promise<void>
将PixelMap写入指定区域内,使用Promise形式返回写入结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
area | 是 | 区域,根据区域写入。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,用于获取写入结果,失败时返回错误信息。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
let opts = { editable: true, pixelFormat: 3, size: { height: 4, width: 6 } }
image.createPixelMap(color, opts)
.then( pixelmap => {
if (pixelmap == undefined) {
console.info('createPixelMap failed.');
}
const area = { pixels: new ArrayBuffer(8),
offset: 0,
stride: 8,
region: { size: { height: 1, width: 2 }, x: 0, y: 0 }
}
let bufferArr = new Uint8Array(area.pixels);
for (var i = 0; i < bufferArr.length; i++) {
bufferArr[i] = i + 1;
}
pixelmap.writePixels(area).then(() => {
console.info('Succeeded to write pixelmap into the specified area.');
})
}).catch(error => {
console.log('error: ' + error);
})
writePixels7+
writePixels(area: PositionArea, callback: AsyncCallback<void>): void
将PixelMap写入指定区域内,使用callback形式返回写入结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
area | 是 | 区域,根据区域写入。 | |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
const area = { pixels: new ArrayBuffer(8),
offset: 0,
stride: 8,
region: { size: { height: 1, width: 2 }, x: 0, y: 0 }
}
let bufferArr = new Uint8Array(area.pixels);
for (var i = 0; i < bufferArr.length; i++) {
bufferArr[i] = i + 1;
}
pixelmap.writePixels(area, (error) => {
if (error != undefined) {
console.info('Failed to write pixelmap into the specified area.');
} else {
console.info('Succeeded to write pixelmap into the specified area.');
}
})
writeBufferToPixels7+
writeBufferToPixels(src: ArrayBuffer): Promise<void>
读取缓冲区中的图片数据,结果写入PixelMap中,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
src | ArrayBuffer | 是 | 图像像素数据。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,用于获取结果,失败时返回错误信息。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
for (var i = 0; i < bufferArr.length; i++) {
bufferArr[i] = i + 1;
}
pixelmap.writeBufferToPixels(color).then(() => {
console.log("Succeeded in writing data from a buffer to a PixelMap.");
}).catch((err) => {
console.error("Failed to write data from a buffer to a PixelMap.");
})
writeBufferToPixels7+
writeBufferToPixels(src: ArrayBuffer, callback: AsyncCallback<void>): void
读取缓冲区中的图片数据,结果写入PixelMap中,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
src | ArrayBuffer | 是 | 图像像素数据。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
for (var i = 0; i < bufferArr.length; i++) {
bufferArr[i] = i + 1;
}
pixelmap.writeBufferToPixels(color, function(err) {
if (err) {
console.error("Failed to write data from a buffer to a PixelMap.");
return;
} else {
console.log("Succeeded in writing data from a buffer to a PixelMap.");
}
});
getImageInfo7+
getImageInfo(): Promise<ImageInfo>
获取图像像素信息,使用Promise形式返回获取的图像像素信息。
系统能力: SystemCapability.Multimedia.Image.Core
返回值:
类型 | 说明 |
Promise<ImageInfo> | Promise实例,用于异步获取图像像素信息,失败时返回错误信息。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let opts = { editable: true, pixelFormat: 2, size: { height: 6, width: 8 } }
image.createPixelMap(color, opts).then(pixelmap => {
if (pixelmap == undefined) {
console.error("Failed to obtain the image pixel map information.");
}
pixelmap.getImageInfo().then(imageInfo => {
if (imageInfo == undefined) {
console.error("Failed to obtain the image pixel map information.");
}
if (imageInfo.size.height == 4 && imageInfo.size.width == 6) {
console.log("Succeeded in obtaining the image pixel map information.");
}
})
})
getImageInfo7+
getImageInfo(callback: AsyncCallback<ImageInfo>): void
获取图像像素信息,使用callback形式返回获取的图像像素信息。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<ImageInfo> | 是 | 获取图像像素信息回调,异步返回图像像素信息,失败时返回错误信息。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let opts = { editable: true, pixelFormat: 3, size: { height: 4, width: 6 } }
image.createPixelMap(color, opts, (err, pixelmap) => {
if (pixelmap == undefined) {
console.error("Failed to obtain the image pixel map information.");
}
pixelmap.getImageInfo((err, imageInfo) => {
if (imageInfo == undefined) {
console.error("Failed to obtain the image pixel map information.");
}
if (imageInfo.size.height == 4 && imageInfo.size.width == 6) {
console.log("Succeeded in obtaining the image pixel map information.");
}
})
})
getBytesNumberPerRow7+
getBytesNumberPerRow(): number
获取图像像素每行字节数。
系统能力: SystemCapability.Multimedia.Image.Core
返回值:
类型 | 说明 |
number | 图像像素的行字节数。 |
示例:
const color = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
let bufferArr = new Uint8Array(color);
let opts = { editable: true, pixelFormat: 3, size: { height: 4, width: 6 } }
image.createPixelMap(color, opts, (err,pixelmap) => {
let rowCount = pixelmap.getBytesNumberPerRow();
})
getPixelBytesNumber7+
getPixelBytesNumber(): number
获取图像像素的总字节数。
系统能力: SystemCapability.Multimedia.Image.Core
返回值:
类型 | 说明 |
number | 图像像素的总字节数。 |
示例:
let pixelBytesNumber = pixelmap.getPixelBytesNumber();
getDensity9+
getDensity():number
获取当前图像像素的密度。
系统能力: SystemCapability.Multimedia.Image.Core
返回值:
类型 | 说明 |
number | 图像像素的密度。 |
示例:
let getDensity = pixelmap.getDensity();
opacity9+
opacity(rate: number, callback: AsyncCallback<void>): void
通过设置透明比率来让PixelMap达到对应的透明效果,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
rate | number | 是 | 透明比率的值,取值范围:0-1。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
var rate = 0.5;
pixelmap.opacity(rate, (err) => {
if (err) {
console.error("Failed to set opacity.");
return;
} else {
console.log("Succeeded in setting opacity.");
}
})
opacity9+
opacity(rate: number): Promise<void>
通过设置透明比率来让PixelMap达到对应的透明效果,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
rate | number | 是 | 透明比率的值,取值范围:0-1。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,用于获取结果,失败时返回错误信息。 |
示例:
async function Demo() {
await pixelmap.opacity(0.5);
}
createAlphaPixelmap9+
createAlphaPixelmap(): Promise<PixelMap>
根据Alpha通道的信息,来生成一个仅包含Alpha通道信息的pixelmap,可用于阴影效果,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
返回值:
类型 | 说明 |
Promise<PixelMap> | Promise实例,返回pixelmap。 |
示例:
async function Demo() {
await pixelmap.createAlphaPixelmap();
}
createAlphaPixelmap9+
createAlphaPixelmap(callback: AsyncCallback<PixelMap>): void
根据Alpha通道的信息,来生成一个仅包含Alpha通道信息的pixelmap,可用于阴影效果,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<PixelMap> | 是 | 获取回调,异步返回结果。 |
示例:
pixelmap.createAlphaPixelmap((err, alphaPixelMap) => {
if (alphaPixelMap == undefined) {
console.info('Failed to obtain new pixel map.');
} else {
console.info('Succeed in obtaining new pixel map.');
}
})
scale9+
scale(x: number, y: number, callback: AsyncCallback<void>): void
根据输入的宽高对图片进行缩放,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
x | number | 是 | 宽度的缩放值,其值为输入的倍数。 |
y | number | 是 | 高度的缩放值,其值为输入的倍数。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
async function Demo() {
await pixelmap.scale(2.0, 1.0);
}
scale9+
scale(x: number, y: number): Promise<void>
根据输入的宽高对图片进行缩放,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
x | number | 是 | 宽度的缩放值,其值为输入的倍数。 |
y | number | 是 | 高度的缩放值,其值为输入的倍数。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,异步返回结果。 |
示例:
async function Demo() {
await pixelmap.scale(2.0, 1.0);
}
translate9+
translate(x: number, y: number, callback: AsyncCallback<void>): void
根据输入的坐标对图片进行位置变换,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
x | number | 是 | 区域横坐标。 |
y | number | 是 | 区域纵坐标。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
async function Demo() {
await pixelmap.translate(3.0, 1.0);
}
translate9+
translate(x: number, y: number): Promise<void>
根据输入的坐标对图片进行位置变换,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
x | number | 是 | 区域横坐标。 |
y | number | 是 | 区域纵坐标。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,异步返回结果。 |
示例:
async function Demo() {
await pixelmap.translate(3.0, 1.0);
}
rotate9+
rotate(angle: number, callback: AsyncCallback<void>): void
根据输入的角度对图片进行旋转,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
angle | number | 是 | 图片旋转的角度。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
async function Demo() {
await pixelmap.rotate(90.0);
}
rotate9+
rotate(angle: number): Promise<void>
根据输入的角度对图片进行旋转,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
angle | number | 是 | 图片旋转的角度。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,异步返回结果。 |
示例:
async function Demo() {
await pixelmap.rotate(90.0);
}
flip9+
flip(horizontal: boolean, vertical: boolean, callback: AsyncCallback<void>): void
根据输入的条件对图片进行翻转,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
horizontal | boolean | 是 | 水平翻转。 |
vertical | boolean | 是 | 垂直翻转。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
async function Demo() {
await pixelmap.flip(false, true);
}
flip9+
flip(horizontal: boolean, vertical: boolean): Promise<void>
根据输入的条件对图片进行翻转,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
horizontal | boolean | 是 | 水平翻转。 |
vertical | boolean | 是 | 垂直翻转。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,异步返回结果。 |
示例:
async function Demo() {
await pixelmap.flip(false, true);
}
crop9+
crop(region: Region, callback: AsyncCallback<void>): void
根据输入的尺寸对图片进行裁剪,使用callback形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
region | Region | 是 | 裁剪的尺寸。 |
callback | AsyncCallback<void> | 是 | 获取回调,失败时返回错误信息。 |
示例:
async function Demo() {
await pixelmap.crop({ x: 0, y: 0, size: { height: 100, width: 100 } });
}
crop9+
crop(region: Region): Promise<void>
根据输入的尺寸对图片进行裁剪,使用Promise形式返回。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
region | Region | 是 | 裁剪的尺寸。 |
返回值:
类型 | 说明 |
Promise<void> | Promise实例,异步返回结果。 |
示例:
async function Demo() {
await pixelmap.crop({ x: 0, y: 0, size: { height: 100, width: 100 } });
}
release7+
release():Promise<void>
释放PixelMap对象,使用Promise形式返回释放结果。
系统能力: SystemCapability.Multimedia.Image.Core
返回值:
类型 | 说明 |
Promise<void> | Promise实例,异步返回释放结果。 |
示例:
pixelmap.release().then(() => {
console.log('Succeeded in releasing pixelmap object.');
}).catch(error => {
console.log('Failed to release pixelmap object.');
})
release7+
release(callback: AsyncCallback<void>): void
释放PixelMap对象,使用callback形式返回释放结果。
系统能力: SystemCapability.Multimedia.Image.Core
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<void> | 是 | 异步返回释放结果。 |
示例:
pixelmap.release(() => {
console.log('Succeeded in releasing pixelmap object.');
})
image.createImageSource
createImageSource(uri: string): ImageSource
通过传入的uri创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
uri | string | 是 | 图片路径,当前仅支持应用沙箱路径。 当前支持格式有:.jpg .png .gif .bmp .webp RAW。 |
返回值:
类型 | 说明 |
返回ImageSource类实例,失败时返回undefined。 |
示例:
let path = this.context.getApplicationContext().fileDirs + "test.jpg";
const imageSourceApi = image.createImageSource(path);
image.createImageSource9+
createImageSource(uri: string, options: SourceOptions): ImageSource
通过传入的uri创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
uri | string | 是 | 图片路径,当前仅支持应用沙箱路径。 当前支持格式有:.jpg .png .gif .bmp .webp RAW。 |
options | 是 | 图片属性,包括图片序号与默认属性值。 |
返回值:
类型 | 说明 |
返回ImageSource类实例,失败时返回undefined。 |
示例:
var sourceOptions = { sourceDensity: 120 };
let imageSource = image.createImageSource('test.png', sourceOptions);
image.createImageSource7+
createImageSource(fd: number): ImageSource
通过传入文件描述符来创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
fd | number | 是 | 文件描述符fd。 |
返回值:
类型 | 说明 |
返回ImageSource类实例,失败时返回undefined。 |
示例:
const imageSourceApi = image.createImageSource(0);
image.createImageSource9+
createImageSource(fd: number, options: SourceOptions): ImageSource
通过传入文件描述符来创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
fd | number | 是 | 文件描述符fd。 |
options | 是 | 图片属性,包括图片序号与默认属性值。 |
返回值:
类型 | 说明 |
返回ImageSource类实例,失败时返回undefined。 |
示例:
var sourceOptions = { sourceDensity: 120 };
const imageSourceApi = image.createImageSource(0, sourceOptions);
image.createImageSource9+
createImageSource(buf: ArrayBuffer): ImageSource
通过缓冲区创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
buf | ArrayBuffer | 是 | 图像缓冲区数组。 |
示例:
const buf = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
const imageSourceApi = image.createImageSource(buf);
image.createImageSource9+
createImageSource(buf: ArrayBuffer, options: SourceOptions): ImageSource
通过缓冲区创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
buf | ArrayBuffer | 是 | 图像缓冲区数组。 |
options | 是 | 图片属性,包括图片序号与默认属性值。 |
返回值:
类型 | 说明 |
返回ImageSource类实例,失败时返回undefined。 |
示例:
const data = new ArrayBuffer(112);
const imageSourceApi = image.createImageSource(data);
image.CreateIncrementalSource9+
CreateIncrementalSource(buf: ArrayBuffer): ImageSource
通过缓冲区以增量的方式创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
buf | ArrayBuffer | 是 | 增量数据。 |
返回值:
类型 | 说明 |
返回图片源,失败时返回undefined。 |
示例:
const buf = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
const imageSourceIncrementalSApi = image.CreateIncrementalSource(buf);
image.CreateIncrementalSource9+
CreateIncrementalSource(buf: ArrayBuffer, options?: SourceOptions): ImageSource
通过缓冲区以增量的方式创建图片源实例。
系统能力: SystemCapability.Multimedia.Image.ImageSource
参数:
参数名 | 类型 | 必填 | 说明 |
buf | ArrayBuffer | 是 | 增量数据。 |
options | 否 | 图片属性,包括图片序号与默认属性值。 |
返回值:
类型 | 说明 |
返回图片源,失败时返回undefined。 |
示例:
const buf = new ArrayBuffer(96); //96为需要创建的像素buffer大小,取值为:height * width *4
const imageSourceIncrementalSApi = image.CreateIncrementalSource(buf);
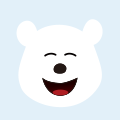