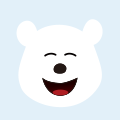
HarmonyOS API:@ohos.account.osAccount (系统帐号管理)
版本:v3.1 Beta
@ohos.account.osAccount (系统帐号管理)
更新时间: 2023-02-17 09:19
本模块提供管理系统帐号的基础能力,包括系统帐号的添加、删除、查询、设置、订阅、启动等功能。
说明
本模块首批接口从API version 7开始支持。后续版本的新增接口,采用上角标单独标记接口的起始版本。
导入模块
import account_osAccount from '@ohos.account.osAccount';
account_osAccount.getAccountManager
getAccountManager(): AccountManager
获取系统帐号管理对象。
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
系统帐号管理对象。 |
示例:
let accountManager = account_osAccount.getAccountManager();
OsAccountType
表示系统帐号类型的枚举。
系统能力: SystemCapability.Account.OsAccount。
名称 | 值 | 说明 |
ADMIN | 0 | 管理员帐号。 |
NORMAL | 1 | 普通帐号。 |
GUEST | 2 | 访客帐号。 |
AccountManager
系统帐号管理类。
checkMultiOsAccountEnabled9+
checkMultiOsAccountEnabled(callback: AsyncCallback<boolean>): void
判断是否支持多系统帐号。使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示支持多系统帐号;返回false表示不支持。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.checkMultiOsAccountEnabled((err, isEnabled) => {
if (err) {
console.log("checkMultiOsAccountEnabled failed, error: " + JSON.stringify(err));
} else {
console.log("checkMultiOsAccountEnabled successfully, isEnabled: " + isEnabled);
}
});
} catch (err) {
console.log("checkMultiOsAccountEnabled exception: " + JSON.stringify(err));
}
checkMultiOsAccountEnabled9+
checkMultiOsAccountEnabled(): Promise<boolean>
判断是否支持多系统帐号。使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示支持多系统帐号;返回false表示不支持。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
try {
let accountManager = account_osAccount.getAccountManager();
accountManager.checkMultiOsAccountEnabled().then((isEnabled) => {
console.log('checkMultiOsAccountEnabled successfully, isEnabled: ' + isEnabled);
}).catch((err) => {
console.log('checkMultiOsAccountEnabled failed, error: ' + JSON.stringify(err));
});
} catch (err) {
console.log('checkMultiOsAccountEnabled exception: ' + JSON.stringify(err));
}
checkOsAccountActivated9+
checkOsAccountActivated(localId: number, callback: AsyncCallback<boolean>): void
判断指定系统帐号是否处于激活状态。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS 或 ohos.permission.INTERACT_ACROSS_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示帐号已激活;返回false表示帐号未激活。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 判断ID为100的系统帐号是否处于激活状态
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.checkOsAccountActivated(localId, (err, isActivated) => {
if (err) {
console.log('checkOsAccountActivated failed, error:' + JSON.stringify(err));
} else {
console.log('checkOsAccountActivated successfully, isActivated:' + isActivated);
}
});
} catch (err) {
console.log('checkOsAccountActivated exception:' + JSON.stringify(err));
}
checkOsAccountActivated9+
checkOsAccountActivated(localId: number): Promise<boolean>
判断指定系统帐号是否处于激活状态。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS 或 ohos.permission.INTERACT_ACROSS_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示帐号已激活;返回false表示帐号未激活。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 判断ID为100的系统帐号是否处于激活状态
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.checkOsAccountActivated(localId).then((isActivated) => {
console.log('checkOsAccountActivated successfully, isActivated: ' + isActivated);
}).catch((err) => {
console.log('checkOsAccountActivated failed, error: ' + JSON.stringify(err));
});
} catch (err) {
console.log('checkOsAccountActivated exception:' + JSON.stringify(err));
}
checkConstraintEnabled9+
checkConstraintEnabled(localId: number, constraint: string, callback: AsyncCallback<boolean>): void
判断指定系统帐号是否具有指定约束。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
constraint | string | 是 | 指定的约束名称。 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示已使能指定的约束;返回false表示未使能指定的约束。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 判断ID为100的系统帐号是否有禁止使用Wi-Fi的约束
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
let constraint = "constraint.wifi";
try {
accountManager.checkConstraintEnabled(localId, constraint, (err, isEnabled)=>{
if (err) {
console.log("checkConstraintEnabled failed, error: " + JSON.stringify(err));
} else {
console.log("checkConstraintEnabled successfully, isEnabled: " + isEnabled);
}
});
} catch (err) {
console.log("checkConstraintEnabled exception: " + JSON.stringify(err));
}
checkConstraintEnabled9+
checkConstraintEnabled(localId: number, constraint: string): Promise<boolean>
判断指定系统帐号是否具有指定约束。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
constraint | string | 是 | 指定的约束名称。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示已使能指定的约束;返回false表示未使能指定的约束。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 判断ID为100的系统帐号是否有禁止使用Wi-Fi的约束
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
let constraint = "constraint.wifi";
try {
accountManager.checkConstraintEnabled(localId, constraint).then((isEnabled) => {
console.log("checkConstraintEnabled successfully, isEnabled: " + isEnabled);
}).catch((err) => {
console.log("checkConstraintEnabled failed, error: " + JSON.stringify(err));
});
} catch (err) {
console.log("checkConstraintEnabled exception: " + JSON.stringify(err));
}
checkOsAccountTestable9+
checkOsAccountTestable(callback: AsyncCallback<boolean>): void
检查当前系统帐号是否为测试帐号。使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示当前帐号为测试帐号;返回false表示当前帐号非测试帐号。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.checkOsAccountTestable((err, isTestable) => {
if (err) {
console.log("checkOsAccountTestable failed, error: " + JSON.stringify(err));
} else {
console.log("checkOsAccountTestable successfully, isTestable: " + isTestable);
}
});
} catch (err) {
console.log("checkOsAccountTestable error: " + JSON.stringify(err));
}
checkOsAccountTestable9+
checkOsAccountTestable(): Promise<boolean>
检查当前系统帐号是否为测试帐号。使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示当前帐号为测试帐号;返回false表示当前帐号非测试帐号。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.checkOsAccountTestable().then((isTestable) => {
console.log("checkOsAccountTestable successfully, isTestable: " + isTestable);
}).catch((err) => {
console.log("checkOsAccountTestable failed, error: " + JSON.stringify(err));
});
} catch (err) {
console.log('checkOsAccountTestable exception: ' + JSON.stringify(err));
}
checkOsAccountVerified9+
checkOsAccountVerified(callback: AsyncCallback<boolean>): void
检查当前系统帐号是否已验证。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS 或 ohos.permission.INTERACT_ACROSS_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示当前帐号已验证;返回false表示当前帐号未验证。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.checkOsAccountVerified((err, isVerified) => {
if (err) {
console.log("checkOsAccountVerified failed, error: " + JSON.stringify(err));
} else {
console.log("checkOsAccountVerified successfully, isVerified: " + isVerified);
}
});
} catch (err) {
console.log("checkOsAccountVerified exception: " + JSON.stringify(err));
}
checkOsAccountVerified9+
checkOsAccountVerified(localId: number, callback: AsyncCallback<boolean>): void
检查指定系统帐号是否已验证。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS 或 ohos.permission.INTERACT_ACROSS_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示指定帐号已验证;返回false表示指定帐号未验证。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例:
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.checkOsAccountVerified(localId, (err, isVerified) => {
if (err) {
console.log("checkOsAccountVerified failed, error: " + JSON.stringify(err));
} else {
console.log("checkOsAccountVerified successfully, isVerified: " + isVerified);
}
});
} catch (err) {
console.log("checkOsAccountVerified exception: " + err);
}
checkOsAccountVerified9+
checkOsAccountVerified(localId?: number): Promise<boolean>
检查指定系统帐号是否已验证。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS 或 ohos.permission.INTERACT_ACROSS_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 否 | 系统帐号ID。不填则检查当前系统帐号是否已验证。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示指定帐号已验证;返回false表示指定帐号未验证。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例:
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.checkOsAccountVerified(localId).then((isVerified) => {
console.log("checkOsAccountVerified successfully, isVerified: " + isVerified);
}).catch((err) => {
console.log("checkOsAccountVerified failed, error: " + JSON.stringify(err));
});
} catch (err) {
console.log('checkOsAccountVerified exception: ' + JSON.stringify(err));
}
getOsAccountCount9+
getOsAccountCount(callback: AsyncCallback<number>): void
获取已创建的系统帐号数量。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<number> | 是 | 回调函数。当获取成功时,err为null,data为已创建的系统帐号的数量;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getOsAccountCount((err, count) => {
if (err) {
console.log("getOsAccountCount failed, error: " + JSON.stringify(err));
} else {
console.log("getOsAccountCount successfully, count: " + count);
}
});
} catch (err) {
console.log("getOsAccountCount exception: " + JSON.stringify(err));
}
getOsAccountCount9+
getOsAccountCount(): Promise<number>
获取已创建的系统帐号数量。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<number> | Promise对象,返回已创建的系统帐号的数量。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getOsAccountCount().then((count) => {
console.log("getOsAccountCount successfully, count: " + count);
}).catch((err) => {
console.log("getOsAccountCount failed, error: " + JSON.stringify(err));
});
} catch(err) {
console.log('getOsAccountCount exception:' + JSON.stringify(err));
}
queryOsAccountLocalIdFromProcess9+
queryOsAccountLocalIdFromProcess(callback: AsyncCallback<number>): void
获取当前进程所属的系统帐号ID,使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<number> | 是 | 回调函数。当获取成功时,err为null,data为当前进程所属的系统帐号ID;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.queryOsAccountLocalIdFromProcess((err, localId) => {
if (err) {
console.log("queryOsAccountLocalIdFromProcess failed, error: " + JSON.stringify(err));
} else {
console.log("queryOsAccountLocalIdFromProcess successfully, localId: " + localId);
}
});
} catch (err) {
console.log("queryOsAccountLocalIdFromProcess exception: " + JSON.stringify(err));
}
queryOsAccountLocalIdFromProcess9+
queryOsAccountLocalIdFromProcess(): Promise<number>
获取当前进程所属的系统帐号ID,使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<number> | Promise对象,返回当前进程所属的系统帐号ID。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.queryOsAccountLocalIdFromProcess().then((localId) => {
console.log("queryOsAccountLocalIdFromProcess successfully, localId: " + localId);
}).catch((err) => {
console.log("queryOsAccountLocalIdFromProcess failed, error: " + JSON.stringify(err));
});
} catch (err) {
console.log('queryOsAccountLocalIdFromProcess exception: ' + JSON.stringify(err));
}
queryOsAccountLocalIdFromUid9+
queryOsAccountLocalIdFromUid(uid: number, callback: AsyncCallback<number>): void
根据uid查询对应的系统帐号ID,使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
uid | number | 是 | 进程uid。 |
callback | AsyncCallback<number> | 是 | 回调函数。如果查询成功,err为null,data为对应的系统帐号ID;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid uid. |
示例: 查询值为12345678的uid所属的系统帐号的帐号ID
let accountManager = account_osAccount.getAccountManager();
let uid = 12345678;
try {
accountManager.queryOsAccountLocalIdFromUid(uid, (err, localId) => {
if (err) {
console.log("queryOsAccountLocalIdFromUid failed, error: " + JSON.stringify(err));
}
console.log("queryOsAccountLocalIdFromUid successfully, localId: " + localId);
});
} catch (err) {
console.log("queryOsAccountLocalIdFromUid exception: " + JSON.stringify(err));
}
queryOsAccountLocalIdFromUid9+
queryOsAccountLocalIdFromUid(uid: number): Promise<number>
根据uid查询对应的系统帐号ID,使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
uid | number | 是 | 进程uid。 |
返回值:
类型 | 说明 |
Promise<number> | Promise对象,返回指定uid对应的系统帐号ID。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid uid. |
示例: 查询值为12345678的uid所属的系统帐号ID
let accountManager = account_osAccount.getAccountManager();
let uid = 12345678;
try {
accountManager.queryOsAccountLocalIdFromUid(uid).then((localId) => {
console.log("queryOsAccountLocalIdFromUid successfully, localId: " + localId);
}).catch((err) => {
console.log("queryOsAccountLocalIdFromUid failed, error: " + JSON.stringify(err));
});
} catch (err) {
console.log('queryOsAccountLocalIdFromUid exception: ' + JSON.stringify(err));
}
queryOsAccountLocalIdFromDomain9+
queryOsAccountLocalIdFromDomain(domainInfo: DomainAccountInfo, callback: AsyncCallback<number>): void
根据域帐号信息,获取与其关联的系统帐号ID。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
domainInfo | 是 | 域帐号信息。 | |
callback | AsyncCallback<number> | 是 | 回调函数。如果查询成功,err为null,data为域帐号关联的系统帐号ID;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid domainInfo. |
示例:
let domainInfo = {domain: 'testDomain', accountName: 'testAccountName'};
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.queryOsAccountLocalIdFromDomain(domainInfo, (err, localId) => {
if (err) {
console.log("queryOsAccountLocalIdFromDomain failed, error: " + JSON.stringify(err));
} else {
console.log("queryOsAccountLocalIdFromDomain successfully, localId: " + localId);
}
});
} catch (err) {
console.log('queryOsAccountLocalIdFromDomain exception: ' + JSON.stringify(err));
}
queryOsAccountLocalIdFromDomain9+
queryOsAccountLocalIdFromDomain(domainInfo: DomainAccountInfo): Promise<number>
根据域帐号信息,获取与其关联的系统帐号的帐号ID。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
domainInfo | 是 | 域帐号信息。 |
返回值:
类型 | 说明 |
Promise<number> | Promise对象,返回域帐号关联的系统帐号ID。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid domainInfo. |
示例:
let accountManager = account_osAccount.getAccountManager();
let domainInfo = {domain: 'testDomain', accountName: 'testAccountName'};
try {
accountManager.queryOsAccountLocalIdFromDomain(domainInfo).then((localId) => {
console.log("queryOsAccountLocalIdFromDomain successfully, localId: " + localId);
}).catch((err) => {
console.log("queryOsAccountLocalIdFromDomain failed, error: " + JSON.stringify(err));
});
} catch (err) {
console.log("queryOsAccountLocalIdFromDomain exception: " + JSON.stringify(err));
}
getOsAccountConstraints9+
getOsAccountConstraints(localId: number, callback: AsyncCallback<Array<string>>): void
获取指定系统帐号的全部约束。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
callback | AsyncCallback<Array<string>> | 是 | 回调函数,如果获取成功,err为null,data为该系统帐号的全部约束;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 获取ID为100的系统帐号的全部约束
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.getOsAccountConstraints(localId, (err, constraints) => {
if (err) {
console.log("getOsAccountConstraints failed, err: " + JSON.stringify(err));
} else {
console.log("getOsAccountConstraints successfully, constraints: " + JSON.stringify(constraints));
}
});
} catch (err) {
console.log('getOsAccountConstraints exception:' + JSON.stringify(err));
}
getOsAccountConstraints9+
getOsAccountConstraints(localId: number): Promise<Array<string>>
获取指定系统帐号的全部约束。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
返回值:
类型 | 说明 |
Promise<Array<string>> | Promise对象,返回指定系统帐号的全部约束。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 获取ID为100的系统帐号的全部约束
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.getOsAccountConstraints(localId).then((constraints) => {
console.log('getOsAccountConstraints, constraints: ' + constraints);
}).catch((err) => {
console.log('getOsAccountConstraints err: ' + JSON.stringify(err));
});
} catch (e) {
console.log('getOsAccountConstraints exception:' + JSON.stringify(e));
}
getActivatedOsAccountIds9+
getActivatedOsAccountIds(callback: AsyncCallback<Array<number>>): void
查询当前处于激活状态的系统帐号的ID列表。使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<Array<number>> | 是 | 回调函数。如果查询成功,err为null,data为当前处于激活状态的系统帐号的ID列表;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getActivatedOsAccountIds((err, idArray)=>{
console.log('getActivatedOsAccountIds err:' + JSON.stringify(err));
console.log('getActivatedOsAccountIds idArray length:' + idArray.length);
for(let i=0;i<idArray.length;i++) {
console.info('activated os account id: ' + idArray[i]);
}
});
} catch (e) {
console.log('getActivatedOsAccountIds exception:' + JSON.stringify(e));
}
getActivatedOsAccountIds9+
getActivatedOsAccountIds(): Promise<Array<number>>
查询当前处于激活状态的系统帐号的ID列表。使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<Array<number>> | Promise对象,返回当前处于激活状态的系统帐号的ID列表。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getActivatedOsAccountIds().then((idArray) => {
console.log('getActivatedOsAccountIds, idArray: ' + idArray);
}).catch((err) => {
console.log('getActivatedOsAccountIds err: ' + JSON.stringify(err));
});
} catch (e) {
console.log('getActivatedOsAccountIds exception:' + JSON.stringify(e));
}
getCurrentOsAccount9+
getCurrentOsAccount(callback: AsyncCallback<OsAccountInfo>): void
查询当前进程所属的系统帐号的信息。使用callback异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<OsAccountInfo> | 是 | 回调函数。如果查询成功,err为null,data为当前进程所属的系统帐号信息;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getCurrentOsAccount((err, curAccountInfo)=>{
console.log('getCurrentOsAccount err:' + JSON.stringify(err));
console.log('getCurrentOsAccount curAccountInfo:' + JSON.stringify(curAccountInfo));
});
} catch (e) {
console.log('getCurrentOsAccount exception:' + JSON.stringify(e));
}
getCurrentOsAccount9+
getCurrentOsAccount(): Promise<OsAccountInfo>
查询当前进程所属的系统帐号的信息。使用Promise异步回调。
需要权限: ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<OsAccountInfo> | Promise对象,返回当前进程所属的系统帐号信息。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getCurrentOsAccount().then((accountInfo) => {
console.log('getCurrentOsAccount, accountInfo: ' + JSON.stringify(accountInfo));
}).catch((err) => {
console.log('getCurrentOsAccount err: ' + JSON.stringify(err));
});
} catch (e) {
console.log('getCurrentOsAccount exception:' + JSON.stringify(e));
}
getOsAccountType9+
getOsAccountType(callback: AsyncCallback<OsAccountType>): void
查询当前进程所属的系统帐号的帐号类型。使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<OsAccountType> | 是 | 回调函数。如果查询成功,err为null,data为当前进程所属的系统帐号的帐号类型;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getOsAccountType((err, accountType) => {
console.log('getOsAccountType err: ' + JSON.stringify(err));
console.log('getOsAccountType accountType: ' + accountType);
});
} catch (e) {
console.log('getOsAccountType exception: ' + JSON.stringify(e));
}
getOsAccountType9+
getOsAccountType(): Promise<OsAccountType>
查询当前进程所属的系统帐号的帐号类型。使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<OsAccountType> | Promise对象,返回当前进程所属的系统帐号的帐号类型。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.getOsAccountType().then((accountType) => {
console.log('getOsAccountType, accountType: ' + accountType);
}).catch((err) => {
console.log('getOsAccountType err: ' + JSON.stringify(err));
});
} catch (e) {
console.log('getOsAccountType exception: ' + JSON.stringify(e));
}
queryDistributedVirtualDeviceId9+
queryDistributedVirtualDeviceId(callback: AsyncCallback<string>): void
获取分布式虚拟设备ID。使用callback异步回调。
需要权限: ohos.permission.DISTRIBUTED_DATASYNC or ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<string> | 是 | 回调函数。如果获取成功,err为null,data为分布式虚拟设备ID;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.queryDistributedVirtualDeviceId((err, virtualID) => {
console.log('queryDistributedVirtualDeviceId err: ' + JSON.stringify(err));
console.log('queryDistributedVirtualDeviceId virtualID: ' + virtualID);
});
} catch (e) {
console.log('queryDistributedVirtualDeviceId exception: ' + JSON.stringify(e));
}
queryDistributedVirtualDeviceId9+
queryDistributedVirtualDeviceId(): Promise<string>
获取分布式虚拟设备ID。使用Promise异步回调。
需要权限: ohos.permission.DISTRIBUTED_DATASYNC or ohos.permission.MANAGE_LOCAL_ACCOUNTS
系统能力: SystemCapability.Account.OsAccount
返回值:
类型 | 说明 |
Promise<string> | Promise对象,返回分布式虚拟设备ID。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
let accountManager = account_osAccount.getAccountManager();
try {
accountManager.queryDistributedVirtualDeviceId().then((virtualID) => {
console.log('queryDistributedVirtualDeviceId, virtualID: ' + virtualID);
}).catch((err) => {
console.log('queryDistributedVirtualDeviceId err: ' + JSON.stringify(err));
});
} catch (e) {
console.log('queryDistributedVirtualDeviceId exception: ' + JSON.stringify(e));
}
queryOsAccountLocalIdBySerialNumber9+
queryOsAccountLocalIdBySerialNumber(serialNumber: number, callback: AsyncCallback<number>): void
通过SN码查询与其关联的系统帐号的帐号ID。使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
serialNumber | number | 是 | 帐号SN码。 |
callback | AsyncCallback<number> | 是 | 回调函数。如果成功,err为null,data为与SN码关联的系统帐号的帐号ID;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid serialNumber. |
12300003 | Account not found. |
示例: 查询与SN码12345关联的系统帐号的ID
let accountManager = account_osAccount.getAccountManager();
let serialNumber = 12345;
try {
accountManager.queryOsAccountLocalIdBySerialNumber(serialNumber, (err, localId)=>{
console.log('ger localId err:' + JSON.stringify(err));
console.log('get localId:' + localId + ' by serialNumber: ' + serialNumber);
});
} catch (e) {
console.log('ger localId exception:' + JSON.stringify(e));
}
queryOsAccountLocalIdBySerialNumber9+
queryOsAccountLocalIdBySerialNumber(serialNumber: number): Promise<number>
通过SN码查询与其关联的系统帐号的帐号ID。使用Promise异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
serialNumber | number | 是 | 帐号SN码。 |
返回值:
类型 | 说明 |
Promise<number> | Promise对象,返回与SN码关联的系统帐号的帐号ID。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid serialNumber. |
12300003 | Account not found. |
示例: 查询与SN码12345关联的系统帐号的ID
let accountManager = account_osAccount.getAccountManager();
let serialNumber = 12345;
try {
accountManager.queryOsAccountLocalIdBySerialNumber(serialNumber).then((localId) => {
console.log('queryOsAccountLocalIdBySerialNumber localId: ' + localId);
}).catch((err) => {
console.log('queryOsAccountLocalIdBySerialNumber err: ' + JSON.stringify(err));
});
} catch (e) {
console.log('queryOsAccountLocalIdBySerialNumber exception: ' + JSON.stringify(e));
}
querySerialNumberByOsAccountLocalId9+
querySerialNumberByOsAccountLocalId(localId: number, callback: AsyncCallback<number>): void
通过系统帐号ID获取与该系统帐号关联的SN码。使用callback异步回调。
系统能力: SystemCapability.Account.OsAccount
参数:
参数名 | 类型 | 必填 | 说明 |
localId | number | 是 | 系统帐号ID。 |
callback | AsyncCallback<number> | 是 | 回调函数。如果获取成功,err为null,data为与该系统帐号关联的SN码;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid localId. |
12300003 | Account not found. |
示例: 获取ID为100的系统帐号关联的SN码
let accountManager = account_osAccount.getAccountManager();
let localId = 100;
try {
accountManager.querySerialNumberByOsAccountLocalId(localId, (err, serialNumber)=>{
console.log('ger serialNumber err:' + JSON.stringify(err));
console.log('get serialNumber:' + serialNumber + ' by localId: ' + localId);
});
} catch (e) {
console.log('ger serialNumber exception:' + JSON.stringify(e));
}
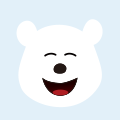