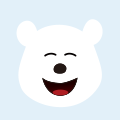
HarmonyOS API:@ohos.account.appAccount (应用帐号管理)
版本:v3.1 Beta
@ohos.account.appAccount (应用帐号管理)
更新时间: 2023-03-03 17:21
本模块提供应用帐号信息的添加、删除、修改和查询基础能力,并支持应用间鉴权和分布式数据同步功能。
说明
本模块首批接口从API version 7开始支持。后续版本的新增接口,采用上角标单独标记接口的起始版本。
导入模块
account_appAccount.createAppAccountManager
createAppAccountManager(): AppAccountManager
创建应用帐号管理器对象。
系统能力: SystemCapability.Account.AppAccount
返回值:
类型 | 说明 |
AppAccountManager | 应用帐号管理器对象。 |
示例:
AppAccountManager
应用帐号管理器类。
createAccount9+
createAccount(name: string, callback: AsyncCallback<void>): void;
根据帐号名创建应用帐号。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
callback | AsyncCallback<void> | 是 | 回调函数。当创建成功时,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300004 | Account already exists. |
12300007 | The number of accounts reaches the upper limit. |
示例:
createAccount9+
createAccount(name: string, options: CreateAccountOptions, callback: AsyncCallback<void>): void
根据帐号名和可选项创建应用帐号。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
options | 是 | 创建应用帐号的选项,可提供自定义数据,但不建议包含敏感数据(如密码、Token等)。 | |
callback | AsyncCallback<void> | 是 | 回调函数。当创建成功时,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or options. |
12300004 | Account already exists. |
12300007 | The number of accounts reaches the upper limit. |
示例:
createAccount9+
createAccount(name: string, options?: CreateAccountOptions): Promise<void>
根据帐号名和可选项创建应用帐号。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
options | 否 | 创建应用帐号的选项,可提供自定义数据,但不建议包含敏感数据(如密码、Token等)。不填无影响。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or options. |
12300004 | Account already exists. |
12300007 | The number of accounts reaches the upper limit. |
12400003 | The number of custom data reaches the upper limit. |
示例:
createAccountImplicitly9+
createAccountImplicitly(owner: string, callback: AuthCallback): void
根据指定的帐号所有者隐式地创建应用帐号。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
owner | string | 是 | 应用帐号所有者的包名。 |
callback | 是 | 认证器回调对象,返回创建结果。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid owner. |
12300007 | The number of accounts reaches the upper limit. |
12300010 | Account service busy. |
12300113 | Authenticator service not found. |
12300114 | Authenticator service exception. |
示例:
createAccountImplicitly9+
createAccountImplicitly(owner: string, options: CreateAccountImplicitlyOptions, callback: AuthCallback): void
根据指定的帐号所有者和可选项隐式地创建应用帐号。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
owner | string | 是 | 应用帐号所有者的包名。 |
options | 是 | 隐式创建帐号的选项。 | |
callback | 是 | 认证器回调对象,返回创建结果。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or options. |
12300007 | The number of accounts reaches the upper limit. |
12300010 | Account service busy. |
12300113 | Authenticator service not found. |
12300114 | Authenticator service exception. |
示例:
removeAccount9+
removeAccount(name: string, callback: AsyncCallback<void>): void
删除应用帐号。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
callback | AsyncCallback<void> | 是 | 回调函数。当删除成功时,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300003 | Account not found. |
示例:
removeAccount9+
removeAccount(name: string): Promise<void>
删除应用帐号。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300003 | Account not found. |
示例:
setAppAccess9+
setAppAccess(name: string, bundleName: string, isAccessible: boolean, callback: AsyncCallback<void>): void
设置指定应用对特定帐号的访问权限。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
bundleName | string | 是 | 第三方应用的包名。 |
isAccessible | boolean | 是 | 是否可访问。true表示允许访问,false表示禁止访问。 |
callback | AsyncCallback<void> | 是 | 回调函数,如果设置成功,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or bundleName. |
12300003 | Account not found. |
12400001 | Application not found. |
示例:
setAppAccess9+
setAppAccess(name: string, bundleName: string, isAccessible: boolean): Promise<void>
设置指定应用对特定帐号的数据访问权限。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
bundleName | string | 是 | 第三方应用的包名。 |
isAccessible | boolean | 是 | 是否可访问。true表示允许访问,false表示禁止访问。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or bundleName. |
12300003 | Account not found. |
12400001 | Application not found. |
示例:
checkAppAccess9+
checkAppAccess(name: string, bundleName: string, callback: AsyncCallback<boolean>): void
检查指定应用对特定帐号的数据是否可访问。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
bundleName | string | 是 | 第三方应用的包名。 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示指定应用可访问特定帐号的数据;返回false表示不可访问。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or bundleName. |
12300003 | Account not found. |
12400001 | Application not found. |
示例:
checkAppAccess9+
checkAppAccess(name: string, bundleName: string): Promise<boolean>
检查指定应用对特定帐号的数据是否可访问。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
bundleName | string | 是 | 第三方应用的包名。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示指定应用可访问特定帐号的数据;返回false表示不可访问。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or bundleName. |
12300003 | Account not found. |
12400001 | Application not found. |
示例:
setDataSyncEnabled9+
setDataSyncEnabled(name: string, isEnabled: boolean, callback: AsyncCallback<void>): void
开启或禁止指定应用帐号的数据同步功能。使用callback异步回调。
需要权限: ohos.permission.DISTRIBUTED_DATASYNC
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
isEnabled | boolean | 是 | 是否开启数据同步。 |
callback | AsyncCallback<void> | 是 | 回调函数。当开启或禁止成功时,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300003 | Account not found. |
示例:
setDataSyncEnabled9+
setDataSyncEnabled(name: string, isEnabled: boolean): Promise<void>
开启或禁止指定应用帐号的数据同步功能。使用Promise异步回调。
需要权限: ohos.permission.DISTRIBUTED_DATASYNC
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
isEnabled | boolean | 是 | 是否开启数据同步。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300003 | Account not found. |
示例:
checkDataSyncEnabled9+
checkDataSyncEnabled(name: string, callback: AsyncCallback<boolean>): void
检查指定应用帐号是否开启数据同步功能。使用callback异步回调。
需要权限: ohos.permission.DISTRIBUTED_DATASYNC
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
callback | AsyncCallback<boolean> | 是 | 回调函数。返回true表示指定应用帐号已开启数据同步功能;返回false表示未开启。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300003 | Account not found. |
示例:
checkDataSyncEnabled9+
checkDataSyncEnabled(name: string): Promise<boolean>
检查指定应用帐号是否开启数据同步功能。使用Promise异步回调。
需要权限: ohos.permission.DISTRIBUTED_DATASYNC
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示指定应用帐号已开启数据同步功能;返回false表示未开启。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name. |
12300003 | Account not found. |
示例:
setCredential9+
setCredential(name: string, credentialType: string, credential: string,callback: AsyncCallback<void>): void
设置指定应用帐号的凭据。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
credentialType | string | 是 | 凭据类型。 |
credential | string | 是 | 凭据取值。 |
callback | AsyncCallback<void> | 是 | 回调函数。当凭据设置成功时,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or credentialType or credential. |
12300003 | Account not found. |
示例:
setCredential9+
setCredential(name: string, credentialType: string, credential: string): Promise<void>
设置指定应用帐号的凭据。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
credentialType | string | 是 | 凭据类型。 |
credential | string | 是 | 凭据取值。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or credentialType or credential. |
12300003 | Account not found. |
示例:
getCredential9+
getCredential(name: string, credentialType: string, callback: AsyncCallback<string>): void
获取指定应用帐号的凭据。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
credentialType | string | 是 | 凭据类型。 |
callback | AsyncCallback<string> | 是 | 回调函数。当获取凭据成功时,err为null,data为指定应用帐号的凭据;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or credentialType. |
12300003 | Account not found. |
12300102 | Credential not found. |
示例:
getCredential9+
getCredential(name: string, credentialType: string): Promise<string>
获取指定应用帐号的凭据。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
credentialType | string | 是 | 凭据类型。 |
返回值:
类型 | 说明 |
Promise<string> | Promise对象,返回指定应用帐号的凭据。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or credentialType. |
12300003 | Account not found. |
12300102 | Credential not found. |
示例:
setCustomData9+
setCustomData(name: string, key: string, value: string, callback: AsyncCallback<void>): void
设置指定应用帐号的自定义数据。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
key | string | 是 | 自定义数据的键名。 |
value | string | 是 | 自定义数据的取值。 |
callback | AsyncCallback<void> | 是 | 回调函数。当设置自定义数据成功时,err为null,否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or key or value. |
12300003 | Account not found. |
12400003 | The number of custom data reaches the upper limit. |
示例:
setCustomData9+
setCustomData(name: string, key: string, value: string): Promise<void>
设置指定应用帐号的自定义数据。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
key | string | 是 | 自定义数据的键名。 |
value | string | 是 | 自定义数据的取值。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or key or value. |
12300003 | Account not found. |
12400003 | The number of custom data reaches the upper limit. |
示例:
getCustomData9+
getCustomData(name: string, key: string, callback: AsyncCallback<string>): void
根据指定键名获取特定应用帐号的自定义数据。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
key | string | 是 | 自定义数据的键名。 |
callback | AsyncCallback<string> | 是 | 回调函数。当获取成功时,err为null,data为自定义数据的取值;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or key. |
12300003 | Account not found. |
12400002 | Custom data not found. |
示例:
getCustomData9+
getCustomData(name: string, key: string): Promise<string>
根据指定键名获取特定应用帐号的自定义数据。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
key | string | 是 | 自定义数据的键名。 |
返回值:
类型 | 说明 |
Promise<string> | Promise对象,返回自定义数据的取值。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or key. |
12300003 | Account not found. |
12400002 | Custom data not found. |
示例:
getCustomDataSync9+
getCustomDataSync(name: string, key: string): string;
根据指定键名获取特定应用帐号的自定义数据。使用同步方式返回结果。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
key | string | 是 | 自定义数据的键名。 |
返回值:
类型 | 说明 |
string | 自定义数据的取值。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or key. |
12300003 | Account not found. |
12400002 | Custom data not found. |
示例:
getAllAccounts9+
getAllAccounts(callback: AsyncCallback<Array<AppAccountInfo>>): void
获取所有可访问的应用帐号信息。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
callback | AsyncCallback<Array<AppAccountInfo>> | 是 | 回调函数。当查询成功时,err为null,data为获取到的应用帐号信息列表;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
getAllAccounts9+
getAllAccounts(): Promise<Array<AppAccountInfo>>
获取所有可访问的应用帐号信息。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
返回值:
类型 | 说明 |
Promise<Array<AppAccountInfo>> | Promise对象,返回全部应用已授权帐号信息对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
示例:
getAccountsByOwner9+
getAccountsByOwner(owner: string, callback: AsyncCallback<Array<AppAccountInfo>>): void
根据应用帐号所有者获取调用方可访问的应用帐号列表。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
owner | string | 是 | 应用帐号所有者的包名。 |
callback | AsyncCallback<Array<AppAccountInfo>> | 是 | 回调函数。如果获取成功,err为null,data为获取到的应用帐号列表;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid owner. |
12400001 | Application not found. |
示例:
getAccountsByOwner9+
getAccountsByOwner(owner: string): Promise<Array<AppAccountInfo>>
根据应用帐号所有者获取调用方可访问的应用帐号列表。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
owner | string | 是 | 应用帐号所有者的包名。 |
返回值:
类型 | 说明 |
Promise<Array<AppAccountInfo>> | Promise对象,返回获取到的应用帐号列表。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid owner. |
12400001 | Application not found. |
示例:
on('accountChange')9+
on(type: 'accountChange', owners: Array<string>, callback: Callback<Array<AppAccountInfo>>): void
订阅指定应用的帐号信息变更事件。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
type | 'accountChange' | 是 | 事件回调类型,支持的事件为'accountChange',当目标应用更新帐号信息时,触发该事件。 |
owners | Array<string> | 是 | 应用帐号所有者的包名列表。 |
callback | Callback<Array<AppAccountInfo>> | 是 | 回调函数,返回信息发生变更的应用帐号列表。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid type or owners. |
12300011 | Callback has been registered. |
12400001 | Application not found. |
示例:
off('accountChange')9+
off(type: 'accountChange', callback?: Callback<Array<AppAccountInfo>>): void
取消订阅帐号信息变更事件。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
type | 'accountChange' | 是 | 事件回调类型,支持的事件为'accountChange',当帐号所有者更新帐号信息时,触发该事件。 |
callback | Callback<Array<AppAccountInfo>> | 否 | 回调函数,返回信息发生变更的应用帐号列表。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid type. |
12300012 | Callback has not been registered. |
示例:
auth9+
auth(name: string, owner: string, authType: string, callback: AuthCallback): void
对应用帐号进行鉴权以获取授权令牌。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
owner | string | 是 | 应用帐号所有者的包名。 |
authType | string | 是 | 鉴权类型。 |
callback | 是 | 回调对象,返回鉴权结果。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or owner or authType. |
12300003 | Account not found. |
12300010 | Account service busy. |
12300113 | Authenticator service not found. |
12300114 | Authenticator service exception. |
示例:
auth9+
auth(name: string, owner: string, authType: string, options: {[key: string]: Object}, callback: AuthCallback): void
对应用帐号进行鉴权以获取授权令牌。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
owner | string | 是 | 应用帐号所有者的包名。 |
authType | string | 是 | 鉴权类型。 |
options | {[key: string]: Object} | 是 | 鉴权所需的可选项。 |
callback | 是 | 回调对象,返回鉴权结果。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or owner or authType. |
12300003 | Account not exist. |
12300010 | Account service busy. |
12300113 | Authenticator service not found. |
12300114 | Authenticator service exception. |
示例:
getAuthToken9+
getAuthToken(name: string, owner: string, authType: string, callback: AsyncCallback<string>): void
获取指定应用帐号的特定鉴权类型的授权令牌。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
owner | string | 是 | 应用帐号所有者的包名。 |
authType | string | 是 | 鉴权类型。 |
callback | AsyncCallback<string> | 是 | 回调函数。当获取成功时,err为null,data为授权令牌值;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name, owner or authType. |
12300003 | Account not found. |
12300107 | AuthType not found. |
示例:
getAuthToken9+
getAuthToken(name: string, owner: string, authType: string): Promise<string>
获取指定应用帐号的特定鉴权类型的授权令牌。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
owner | string | 是 | 应用帐号所有者的包名。 |
authType | string | 是 | 鉴权类型。 |
返回值:
类型 | 说明 |
Promise<string> | Promise对象,返回授权令牌。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or owner or authType. |
12300003 | Account not found. |
12300107 | AuthType not found. |
示例:
setAuthToken9+
setAuthToken(name: string, authType: string, token: string, callback: AsyncCallback<void>): void
为指定应用帐号设置特定鉴权类型的授权令牌。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
authType | string | 是 | 鉴权类型。 |
token | string | 是 | 授权令牌。 |
callback | AsyncCallback<void> | 是 | 回调函数。当设置成功时,err为null;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or authType or token. |
12300003 | Account not found. |
12400004 | The number of token reaches the upper limit. |
示例:
setAuthToken9+
setAuthToken(name: string, authType: string, token: string): Promise<void>
为指定应用帐号设置特定鉴权类型的授权令牌。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
authType | string | 是 | 鉴权类型。 |
token | string | 是 | 授权令牌。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or authType or token. |
12300003 | Account not found. |
12400004 | The number of token reaches the upper limit. |
示例:
deleteAuthToken9+
deleteAuthToken(name: string, owner: string, authType: string, token: string, callback: AsyncCallback<void>): void
删除指定应用帐号的特定鉴权类型的授权令牌。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
owner | string | 是 | 应用帐号所有者的包名。 |
authType | string | 是 | 鉴权类型。 |
token | string | 是 | 授权令牌。 |
callback | AsyncCallback<void> | 是 | 回调函数。当删除成功时,err为null;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or owner or authType or token. |
12300003 | Account not found. |
12300107 | AuthType not found. |
示例:
deleteAuthToken9+
deleteAuthToken(name: string, owner: string, authType: string, token: string): Promise<void>
删除指定应用帐号的特定鉴权类型的授权令牌。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
owner | string | 是 | 应用帐号所有者的包名。 |
authType | string | 是 | 鉴权类型。 |
token | string | 是 | 授权令牌。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or owner or authType or token. |
12300003 | Account not found. |
12300107 | AuthType not found. |
示例:
setAuthTokenVisibility9+
setAuthTokenVisibility(name: string, authType: string, bundleName: string, isVisible: boolean, callback: AsyncCallback<void>): void
设置指定帐号的特定鉴权类型的授权令牌对指定应用的可见性。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
authType | string | 是 | 鉴权类型。 |
bundleName | string | 是 | 被设置可见性的应用包名。 |
isVisible | boolean | 是 | 是否可见。true表示可见,false表示不可见。 |
callback | AsyncCallback<void> | 是 | 回调函数。当设置成功时,err为null;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or authType or bundleName. |
12300003 | Account not found. |
12300107 | AuthType not found. |
12400001 | Application not found. |
12400005 | The size of authorization list reaches the upper limit. |
示例:
setAuthTokenVisibility9+
setAuthTokenVisibility(name: string, authType: string, bundleName: string, isVisible: boolean): Promise<void>
设置指定帐号的特定鉴权类型的授权令牌对指定应用的可见性。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
authType | string | 是 | 鉴权类型。 |
bundleName | string | 是 | 被设置可见性的应用包名。 |
isVisible | boolean | 是 | 是否可见。true表示可见,false表示不可见。 |
返回值:
类型 | 说明 |
Promise<void> | 无返回结果的Promise对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or authType or bundleName. |
12300003 | Account not found. |
12300107 | AuthType not found. |
12400001 | Application not found. |
12400005 | The size of authorization list reaches the upper limit. |
示例:
checkAuthTokenVisibility9+
checkAuthTokenVisibility(name: string, authType: string, bundleName: string, callback: AsyncCallback<boolean>): void
检查指定应用帐号的特定鉴权类型的授权令牌对指定应用的可见性。使用callback异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
authType | string | 是 | 鉴权类型。 |
bundleName | string | 是 | 检查可见性的应用包名。 |
callback | AsyncCallback<boolean> | 是 | 回调函数。当检查成功时,err为null,data为true表示可见,data为false表示不可见;否则为错误对象。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or authType or bundleName. |
12300003 | Account not found. |
12300107 | AuthType not found. |
12400001 | Application not found. |
示例:
checkAuthTokenVisibility9+
checkAuthTokenVisibility(name: string, authType: string, bundleName: string): Promise<boolean>
检查指定应用帐号的特定鉴权类型的授权令牌对指定应用的可见性。使用Promise异步回调。
系统能力: SystemCapability.Account.AppAccount
参数:
参数名 | 类型 | 必填 | 说明 |
name | string | 是 | 应用帐号的名称。 |
authType | string | 是 | 鉴权类型。 |
bundleName | string | 是 | 用于检查可见性的应用包名。 |
返回值:
类型 | 说明 |
Promise<boolean> | Promise对象。返回true表示授权令牌对指定应用的可见,返回false表示不可见。 |
错误码:
错误码ID | 错误信息 |
12300001 | System service exception. |
12300002 | Invalid name or authType or bundleName. |
12300003 | Account not found. |
12300107 | AuthType not found. |
12400001 | Application not found. |
示例:
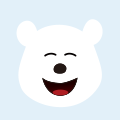