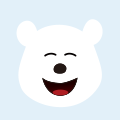
回复
Uniform Resource Location
,统一资源定位符mailto:clswcl@gmail.com
就是一种URN,知道这是个邮箱,却不知道该怎么查找定位
协议://主机名:端口/路径?查询#片段
[protocol]:[//host:port][/path][?query][#fragment]
//基于URL模式构造URL实例
public URL(String spec) throws MalformedURLException
//其中file相当于path、query和fragment三个部分组成
public URL(String protocol, String host, int port, String file) throws MalformedURLException
//根据类加载器获取URL
URL systemResource = ClassLoader.getSystemResource(String name)
Enumeration<URL> systemResources = ClassLoader.getSystemResources(String name)
URL resource = Main.class.getResource(String name)
Enumeration<URL> resources = Main.class.getClassLoader().getResources(String name)
public final InputStream openStream() throws java.io.IOException
public URLConnection openConnection() throws java.io.IOException
public final Object getContent() throws java.io.IOException
○ 通过Resource接口的子类获取资源
○ 通过ResourceLoader接口的子类获取资源
public interface Resource extends InputStreamSource {
//判断资源是否存在
boolean exists(); //
//返回当前资源对应的URL,不能解析则会抛出异常;如ByteArrayResource就不能解析为一个URL
URL getURL() throws IOException;
//返回当前资源对应的URI
URI getURI() throws IOException;
//返回当前资源对应的File
File getFile() throws IOException;
//返回对应的ReadableByteChannel
default ReadableByteChannel readableChannel() throws IOException
Resource resource = new FileSystemResource("D:/example.txt");
File file= new File("example.txt");
Resource resource2 = new FileSystemResource(file);
○ 基于ByteArrayInputStream和字节数组实现,应用场景类似ByteArrayInputStream,缓存byte[]资源
public class ClassPathResource extends AbstractFileResolvingResource {
//ClassPathResource.java 的三个属性
private final String path;
//使用Class或ClassLoader加载资源
private ClassLoader classLoader;
private Class<?> clazz;
---使用方式----
Resource resource = new ClassPathResource("test.txt");
resource.png
public interface ResourceLoader {
// 默认从类路径加载的资源 前缀: "classpath:",获取ClassPathResource
String CLASSPATH_URL_PREFIX = ResourceUtils.CLASSPATH_URL_PREFIX;
Resource getResource(String location);
public interface ResourcePatternResolver extends ResourceLoader {
// 默认加载所有路径(包括jar包)下面的文件,"classpath*:", 获取ClassPathResource
String CLASSPATH_ALL_URL_PREFIX = "classpath*:";
//Ant风格表达式 com/smart/**/*.xml
ResourcePatternResoler resolver = new PathMatchingResourcePatternResolver();
Resource resources[] = resolver.getResources("com/smart/**/*.xml");
// ApplicationContext ctx
//FileSystemResource资源
Resource template = ctx.getResource("file:///res.txt");
//UrlResource资源
Resource template = ctx.getResource("https://my.cn/res.txt");
前缀 | 示例 | 描述 |
classpath: | classpath:config.xml | 从类路径加载 |
file: | file:///res.txt | 从文件系统加载FileSystemResource |
http: | 加载UrlResource |
public class Properties extends Hashtable<Object,Object>{
.... //可根据Reader或者InputStream加载properties文件内容
public synchronized void load(Reader reader) throws IOException
public synchronized void load(InputStream inStream) throws IOException
//res.properties
username = root
password = password
-------代码示例-------------
InputStream input = ClassLoader.getSystemResourceAsStream("res.properties");
Properties prop = new Properties();
prop.load(inputStream); //根据inputStream载入资源
String username = prop.getProperty("username");
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-yaml</artifactId>
<version>2.9.5</version>
</dependency>
//res.yml 配置
name: chen
params:
url: http://www.my.com
----------代码示例---------------
InputStream input = ClassLoader.getSystemResourceAsStream("res.yml");
Yaml yml = new Yaml();
Map map = new Yaml().loadAs(input, LinkedHashMap.class);; //根据inputStream载入资源
String name = MapUtils.getString(map,"name"); // chen
//url: http://www.my.com
String url = MapUtils.getString((Map)map.get("params"),"url")
public class YSOAPConnection implements AutoCloseable {
private SOAPConnection connection;
public static YSOAPConnection open(SOAPConnectionFactory soapConnectionFactory) throws SOAPException {
YSOAPConnection ySoapConnection = new YSOAPConnection();
SOAPConnection connection = soapConnectionFactory.createConnection();
ySoapConnection.setConnection(connection);
return ySoapConnection;
}
public SOAPMessage call(SOAPMessage request, Object to) throws SOAPException {
return connection.call(request, to);
}
@Override
public void close() throws SOAPException {
if (connection != null) { connection.close(); }
}
}
//自动关闭的资源类使用示例
try (YSOAPConnection soapConnection=YSOAPConnection.open(soapConnectionFactory)){
SOAPMessage soapResponse = soapConnection.call(request, endpoint);
...//数据操作
} catch (Exception e) {
log.error(e.getMessage(), e);
...
}
public void close()
;对象需实现AutoCloseable接口
import lombok.Cleanup;
@Cleanup // @Cleanup的使用
YSOAPConnection soapConnection=YSOAPConnection.open(soapConnectionFactory)
//FileInputStream.java - JDK8
//jdk8的FileInputStream重写了finalize,保证对象回收前开启的资源被关闭
protected void finalize () throws IOException {
if (guard != null) {
guard.warnIfOpen();
}
if ((fd != null) && (fd != FileDescriptor.in)) {
close();
}
}
文章转载自公众号:潜行前行