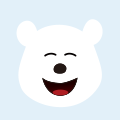
回复
1.申请ohos.permission.PUBLISH_AGENT_REMINDER权限。
2.使能通知开关。获得用户授权后,才能使用代理提醒功能。
3.导入模块。
import reminderAgentManager from '@ohos.reminderAgentManager';
import notificationManager from '@ohos.notificationManager';
4.定义目标提醒代理。开发者根据实际需要,选择定义如下类型的提醒。
定义倒计时实例。
let targetReminderAgent: reminderAgentManager.ReminderRequestTimer = {
reminderType: reminderAgentManager.ReminderType.REMINDER_TYPE_TIMER, // 提醒类型为倒计时类型
triggerTimeInSeconds: 10,
actionButton: [// 设置弹出的提醒通知信息上显示的按钮类型和标题
{
title: 'close',
type: reminderAgentManager.ActionButtonType.ACTION_BUTTON_TYPE_CLOSE
}
],
wantAgent: { // 点击提醒通知后跳转的目标UIAbility信息
pkgName: 'com.example.myapplication',
abilityName: 'EntryAbility'
},
maxScreenWantAgent: { // 全屏显示提醒到达时自动拉起的目标UIAbility信息
pkgName: 'com.example.myapplication',
abilityName: 'EntryAbility'
},
title: 'this is title', // 指明提醒标题
content: 'this is content', // 指明提醒内容
expiredContent: 'this reminder has expired', // 指明提醒过期后需要显示的内容
notificationId: 100, // 指明提醒使用的通知的ID号,相同ID号的提醒会覆盖
slotType: notificationManager.SlotType.SOCIAL_COMMUNICATION // 指明提醒的Slot类型
}
定义日历实例。
let targetReminderAgent: reminderAgentManager.ReminderRequestCalendar = {
reminderType: reminderAgentManager.ReminderType.REMINDER_TYPE_CALENDAR, // 提醒类型为日历类型
dateTime: { // 指明提醒的目标时间
year: 2023,
month: 1,
day: 1,
hour: 11,
minute: 14,
second: 30
},
repeatMonths: [1], // 指明重复提醒的月份
repeatDays: [1], // 指明重复提醒的日期
actionButton: [// 设置弹出的提醒通知信息上显示的按钮类型和标题
{
title: 'close',
type: reminderAgentManager.ActionButtonType.ACTION_BUTTON_TYPE_CLOSE
},
{
title: 'snooze',
type: reminderAgentManager.ActionButtonType.ACTION_BUTTON_TYPE_SNOOZE
},
],
wantAgent: { // 点击提醒通知后跳转的目标UIAbility信息
pkgName: 'com.example.myapplication',
abilityName: 'EntryAbility'
},
maxScreenWantAgent: { // 全屏显示提醒到达时自动拉起的目标UIAbility信息
pkgName: 'com.example.myapplication',
abilityName: 'EntryAbility'
},
ringDuration: 5, // 指明响铃时长(单位:秒)
snoozeTimes: 2, // 指明延迟提醒次数
timeInterval: 5, // 执行延迟提醒间隔(单位:秒)
title: 'this is title', // 指明提醒标题
content: 'this is content', // 指明提醒内容
expiredContent: 'this reminder has expired', // 指明提醒过期后需要显示的内容
snoozeContent: 'remind later', // 指明延迟提醒时需要显示的内容
notificationId: 100, // 指明提醒使用的通知的ID号,相同ID号的提醒会覆盖
slotType: notificationManager.SlotType.SOCIAL_COMMUNICATION // 指明提醒的Slot类型
}
定义闹钟实例。
let targetReminderAgent: reminderAgentManager.ReminderRequestAlarm = {
reminderType: reminderAgentManager.ReminderType.REMINDER_TYPE_ALARM, // 提醒类型为闹钟类型
hour: 23, // 指明提醒的目标时刻
minute: 9, // 指明提醒的目标分钟
daysOfWeek: [2], // 指明每周哪几天需要重复提醒
actionButton: [ // 设置弹出的提醒通知信息上显示的按钮类型和标题
{
title: 'close',
type: reminderAgentManager.ActionButtonType.ACTION_BUTTON_TYPE_CLOSE
},
{
title: 'snooze',
type: reminderAgentManager.ActionButtonType.ACTION_BUTTON_TYPE_SNOOZE
},
],
wantAgent: { // 点击提醒通知后跳转的目标UIAbility信息
pkgName: 'com.example.myapplication',
abilityName: 'EntryAbility'
},
maxScreenWantAgent: { // 全屏显示提醒到达时自动拉起的目标UIAbility信息
pkgName: 'com.example.myapplication',
abilityName: 'EntryAbility'
},
ringDuration: 5, // 指明响铃时长(单位:秒)
snoozeTimes: 2, // 指明延迟提醒次数
timeInterval: 5, // 执行延迟提醒间隔(单位:秒)
title: 'this is title', // 指明提醒标题
content: 'this is content', // 指明提醒内容
expiredContent: 'this reminder has expired', // 指明提醒过期后需要显示的内容
snoozeContent: 'remind later', // 指明延迟提醒时需要显示的内容
notificationId: 99, // 指明提醒使用的通知的ID号,相同ID号的提醒会覆盖
slotType: notificationManager.SlotType.SOCIAL_COMMUNICATION // 指明提醒的Slot类型
}
5.发布相应的提醒代理。代理发布后,应用即可使用后台代理提醒功能。
reminderAgentManager.publishReminder(targetReminderAgent).then(res => {
console.info('Succeeded in publishing reminder. ');
let reminderId: number = res; // 发布的提醒ID
}).catch(err => {
console.error(`Failed to publish reminder. Code: ${err.code}, message: ${err.message}`);
})
6.根据需要删除提醒任务。
// reminderId的值从发布提醒代理成功之后的回调中获得
reminderAgentManager.cancelReminder(reminderId).then(() => {
console.info('Succeeded in canceling reminder.');
}).catch(err => {
console.error(`Failed to cancel reminder. Code: ${err.code}, message: ${err.message}`);
});
本文根据HarmonyOS官方开发文档学习整理