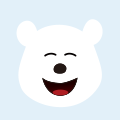
笔记:事件分发机制(二):ViewGroup的事件分发
前言
做一下笔记。从源码角度深入分析和理解一下ViewGroup的事件分发。
ViewGroup
ViewGroup是View 的子类,一般作为容器,盛放其他View和ViewGroup。是Android布局控件的直接或间接父类,像LinearLayout、FrameLayout、RelativeLayout等都属于ViewGroup的子类。ViewGroup与View相比,多了可以包含子View和定义布局参数的功能。ViewGroup的继承关系如下:
从ViewGroup的子类中可以找到平常经常用到的布局控件。
ViewGroup的事件分发流程
demo
简单了解了一下ViewGroup,接下来用demo探索ViewGroup的事件分发流程。
首先,自定义一个布局命名为MyLayout继承自LinearLayout,如下所示:
public class MyLayout extends LinearLayout {
public MyLayout(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
}
然后打开布局文件 activity_view_group.xml ,用MyLayout作为根布局,设置属性,添加控件。如下:
<?xml version="1.0" encoding="utf-8"?>
<com.wzhy.dispatcheventdemo.MyLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button1"
/>
<Button
android:id="@+id/button2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button2"
/>
</com.wzhy.dispatcheventdemo.MyLayout>
在MyLayout布局中添加两个按钮,接着在Activity中为MyLayout和两个按钮添加监听事件:
mLayout.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
Log.i("TAG", " MyLayout: onTouch === action: " + event.getAction());
return false;
}
});
mButton1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.i("TAG", "Button1: onClick Button1");
}
});
mButton2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Log.i("TAG", "Button2: onClick Button2");
}
});
设置好监听事件,运行一下:
分别点击Button1、Button2和空白区域,打印结果如下:
根据log打印可以看出,当点击按钮时,MyLayout注册的触摸监听(OnTouchEvent)的onTouch方法并没有执行,只有点击空白区域时才会执行onTouch方法。此时,可以先理解为Button的onClick方法将事件消费掉了,因此事件不再向下传递。
那么事件的传递流程到底是怎样的呢?难道是事件先经过子View再到ViewGroup吗?欲知答案,继续跟进…
源码分析
onInterceptTouchEvent事件拦截
在事件分发机制中,ViewGroup有一个View不具备的方法–onInterceptTouchEvent
/**
* Implement this method to intercept all touch screen motion events. This
* allows you to watch events as they are dispatched to your children, and
* take ownership of the current gesture at any point.
*
* <p>Using this function takes some care, as it has a fairly complicated
* interaction with {@link View#onTouchEvent(MotionEvent)
* View.onTouchEvent(MotionEvent)}, and using it requires implementing
* that method as well as this one in the correct way. Events will be
* received in the following order:
*
* <ol>
* <li> You will receive the down event here.
* <li> The down event will be handled either by a child of this view
* group, or given to your own onTouchEvent() method to handle; this means
* you should implement onTouchEvent() to return true, so you will
* continue to see the rest of the gesture (instead of looking for
* a parent view to handle it). Also, by returning true from
* onTouchEvent(), you will not receive any following
* events in onInterceptTouchEvent() and all touch processing must
* happen in onTouchEvent() like normal.
* <li> For as long as you return false from this function, each following
* event (up to and including the final up) will be delivered first here
* and then to the target's onTouchEvent().
* <li> If you return true from here, you will not receive any
* following events: the target view will receive the same event but
* with the action {@link MotionEvent#ACTION_CANCEL}, and all further
* events will be delivered to your onTouchEvent() method and no longer
* appear here.
* </ol>
*
* @param ev The motion event being dispatched down the hierarchy.
* @return Return true to steal motion events from the children and have
* them dispatched to this ViewGroup through onTouchEvent().
* The current target will receive an ACTION_CANCEL event, and no further
* messages will be delivered here.
*/
public boolean onInterceptTouchEvent(MotionEvent ev) {
if (ev.isFromSource(InputDevice.SOURCE_MOUSE)
&& ev.getAction() == MotionEvent.ACTION_DOWN
&& ev.isButtonPressed(MotionEvent.BUTTON_PRIMARY)
&& isOnScrollbarThumb(ev.getX(), ev.getY())) {
return true;
}
return false;
}
源码注释很多,代码很简单,返回值是boolean类型,既然如此,可以重写这个方法,返回一个true试试。
public class MyLayout extends LinearLayout {
public MyLayout(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
@Override
public boolean onInterceptTouchEvent(MotionEvent ev) {
super.onInterceptTouchEvent(ev);
return true;
}
}
再次运行,分别点击Button1、Button2和空白区域,log打印如下:
发现不管在哪里点击,只会触发MyLayout的touch事件,按钮的点击事件被屏蔽了!这是为什么?如果touch事件先传递到子控件后传递到ViewGroup,那么MyLayout怎么可能屏蔽掉Button的点击事件呢?明明之前点击MyLayout中的Button,只触发了按钮的点击事件,没有触发MyLayout的touch事件。
只能从源码中找答案,才能解决心中的疑惑。事先说明,Android中的touch事件的传递,绝对是先传递到ViewGroup,后传递到View的。
ViewGroup的事件分发dispatchTouchEvent
记得在上一篇(笔记:事件分发机制(一):View的事件分发)中分析过,当触摸一个控件,都会先调用该控件的dispatchTouchEvent()方法。说法没错,但并不完整。实际情况是当你触摸某个控件,首先调用该控件所在布局的dispatchTouchEvent()方法,然后在布局的dispatchTouchEvent()方法中找到相应的被触摸控件,然后在调用该空间的dispatchTouchEvent()方法。所以,当我们点击了MyLayout的按钮,首先会调用MyLayout的dispatchTouchEvent()方法,这时会发现MyLayout和它的父类LinearLayout中没有这个方法,继续往上找在ViewGroup中发现了dispatchTouchEvent()方法。
既然在ViewGroup找到了dispatchTouchEvent()方法,那么就看一下这里的dispatchTouchEvent()方法:
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
if (mInputEventConsistencyVerifier != null) {
mInputEventConsistencyVerifier.onTouchEvent(ev, 1);
}
// If the event targets the accessibility focused view and this is it, start
// normal event dispatch. Maybe a descendant is what will handle the click.
if (ev.isTargetAccessibilityFocus() && isAccessibilityFocusedViewOrHost()) {
ev.setTargetAccessibilityFocus(false);
}
boolean handled = false;
if (onFilterTouchEventForSecurity(ev)) {
final int action = ev.getAction();
final int actionMasked = action & MotionEvent.ACTION_MASK;
// Handle an initial down. 处理一个初始化的按下动作
if (actionMasked == MotionEvent.ACTION_DOWN) {
// Throw away all previous state when starting a new touch gesture.
// The framework may have dropped the up or cancel event for the previous gesture
// due to an app switch, ANR, or some other state change.
//当启动一个新的触摸手势,摒弃所有之前的状态。
//由于App切换、ANR(应用无响应),或一些其他状态改变,框架可能会放弃先前手势的up或cancel事件。
cancelAndClearTouchTargets(ev);//取消和清除TouchTarget
resetTouchState();//重置触摸状态,会将mFirstTouchTarget置空,FLAG_DISALLOW_INTERCEPT重置
}
// Check for interception.//检查拦截
final boolean intercepted;
//如果是按下或者mFirstTouchTarget不为空(初始按下时,会寻找触摸目标),当事件由ViewGroup的子元素
//成功处理时,mFirstTouchTarget会被赋值并指向子元素,也就是
//ViewGroup不拦截事件并将事件交由子元素处理时mFirstTouchTarget!=null
if (actionMasked == MotionEvent.ACTION_DOWN
|| mFirstTouchTarget != null) {
//FLAG_DISALLOW_INTERCEPT,可通过子View通过requestDisallowInterceptTouchEvent方法设置
final boolean disallowIntercept = (mGroupFlags & FLAG_DISALLOW_INTERCEPT) != 0;
if (!disallowIntercept) {//如果允许拦截
intercepted = onInterceptTouchEvent(ev);//调用ViewGroup自己的事件拦截
ev.setAction(action); // restore action in case it was changed
} else {
intercepted = false;
}
} else {
// There are no touch targets and this action is not an initial down
// so this view group continues to intercept touches.
//没有触摸目标(mFirstTouchTarget == null)且当前动作(action)不是初始按下动作
//那么当前ViewGroup继续拦截触摸事件。也就是点击位置的ViewGroup内没有找到目标View,
//事件由这个ViewGroup拦截处理。比如,点击ViewGroup内空白处。
intercepted = true;
}
//如果已拦截或已经有一个View正在处理手势,正常事件分发。
// If intercepted, start normal event dispatch. Also if there is already
// a view that is handling the gesture, do normal event dispatch.
if (intercepted || mFirstTouchTarget != null) {
ev.setTargetAccessibilityFocus(false);
}
// Check for cancelation.//检查是否取消。
final boolean canceled = resetCancelNextUpFlag(this)
|| actionMasked == MotionEvent.ACTION_CANCEL;
// Update list of touch targets for pointer down, if needed.
//如果需要,更新手指按下后一些列touch目标。
final boolean split = (mGroupFlags & FLAG_SPLIT_MOTION_EVENTS) != 0;
//新的touch目标
TouchTarget newTouchTarget = null;
boolean alreadyDispatchedToNewTouchTarget = false;
//动作(手势)没有取消,且事件没有被当前ViewGroup拦截,
//事件会向下分发,交由它的子类处理。
if (!canceled && !intercepted) {
// If the event is targeting accessiiblity focus we give it to the
// view that has accessibility focus and if it does not handle it
// we clear the flag and dispatch the event to all children as usual.
// We are looking up the accessibility focused host to avoid keeping
// state since these events are very rare.
View childWithAccessibilityFocus = ev.isTargetAccessibilityFocus()
? findChildWithAccessibilityFocus() : null;
if (actionMasked == MotionEvent.ACTION_DOWN
|| (split && actionMasked == MotionEvent.ACTION_POINTER_DOWN)
|| actionMasked == MotionEvent.ACTION_HOVER_MOVE) {
final int actionIndex = ev.getActionIndex(); // always 0 for down
final int idBitsToAssign = split ? 1 << ev.getPointerId(actionIndex)
: TouchTarget.ALL_POINTER_IDS;
// Clean up earlier touch targets for this pointer id in case they
// have become out of sync.
//清除当前触摸点之前的触摸目标以防止不同步。
removePointersFromTouchTargets(idBitsToAssign);
//遍历ViewGroup所有子元素,然后判断子元素是否能够接收到事件。
final int childrenCount = mChildrenCount;
if (newTouchTarget == null && childrenCount != 0) {
final float x = ev.getX(actionIndex);
final float y = ev.getY(actionIndex);
// Find a child that can receive the event. 找到一个能够接收事件的子控件。
// Scan children from front to back. 从前往后扫描ViewGroup的子控件。
final ArrayList<View> preorderedList = buildTouchDispatchChildList();
final boolean customOrder = preorderedList == null
&& isChildrenDrawingOrderEnabled();
final View[] children = mChildren;
//遍历ViewGroup的所有子View。
for (int i = childrenCount - 1; i >= 0; i--) {
final int childIndex = getAndVerifyPreorderedIndex(
childrenCount, i, customOrder);
final View child = getAndVerifyPreorderedView(
preorderedList, children, childIndex);
// If there is a view that has accessibility focus we want it
// to get the event first and if not handled we will perform a
// normal dispatch. We may do a double iteration but this is
// safer given the timeframe.
if (childWithAccessibilityFocus != null) {
if (childWithAccessibilityFocus != child) {
continue;
}
childWithAccessibilityFocus = null;
i = childrenCount - 1;
}
//重要:触摸点位置是否在子View的范围内,或者子View是否可见或在播放动画;
//如果均不符合,则continue, 表示子View不符合条件,开始遍历下一个子View
if (!canViewReceivePointerEvents(child)
|| !isTransformedTouchPointInView(x, y, child, null)) {
ev.setTargetAccessibilityFocus(false);
continue;
}
newTouchTarget = getTouchTarget(child);
if (newTouchTarget != null) {
// Child is already receiving touch within its bounds.
// Give it the new pointer in addition to the ones it is handling.
newTouchTarget.pointerIdBits |= idBitsToAssign;
break;
}
resetCancelNextUpFlag(child);
//分发转换过的触摸事件,获取最后按下的子View的index。
if (dispatchTransformedTouchEvent(ev, false, child, idBitsToAssign)) {
// Child wants to receive touch within its bounds.
mLastTouchDownTime = ev.getDownTime();
if (preorderedList != null) {
// childIndex points into presorted list, find original index
for (int j = 0; j < childrenCount; j++) {
if (children[childIndex] == mChildren[j]) {
mLastTouchDownIndex = j;
break;
}
}
} else {
mLastTouchDownIndex = childIndex;
}
mLastTouchDownX = ev.getX();
mLastTouchDownY = ev.getY();
//为子View添加一个TouchTarget,并把这个TouchTarget赋值给mFirstTouchTarget
newTouchTarget = addTouchTarget(child, idBitsToAssign);
alreadyDispatchedToNewTouchTarget = true;
break;
}
// The accessibility focus didn't handle the event, so clear
// the flag and do a normal dispatch to all children.
ev.setTargetAccessibilityFocus(false);
}
if (preorderedList != null) preorderedList.clear();
}
if (newTouchTarget == null && mFirstTouchTarget != null) {
// Did not find a child to receive the event.
// Assign the pointer to the least recently added target.
newTouchTarget = mFirstTouchTarget;
while (newTouchTarget.next != null) {
newTouchTarget = newTouchTarget.next;
}
newTouchTarget.pointerIdBits |= idBitsToAssign;
}
}
}
//如果找不到子View来处理事件,最后交由ViewGroup来处理
// Dispatch to touch targets.
if (mFirstTouchTarget == null) {
//没有触摸的目标,所以把当前ViewGroup当做普通View(,去处理触摸事件)。
// No touch targets so treat this as an ordinary view.
handled = dispatchTransformedTouchEvent(ev, canceled, null,
TouchTarget.ALL_POINTER_IDS);
} else {
//mFirstTouchTarget不为空,表示已找到一个子View来消耗事件
// Dispatch to touch targets, excluding the new touch target if we already
// dispatched to it. Cancel touch targets if necessary.
//分发事件给触摸目标,不包括已经分发过额这个新触摸目标。
TouchTarget predecessor = null;
TouchTarget target = mFirstTouchTarget;
while (target != null) {
final TouchTarget next = target.next;
//如果已分发事件给新的触摸目标,且当前目标就是新的触摸目标,
//表示事件已经被这个触摸目标消费掉,不再给它分发事件,继续下一个触摸目标
if (alreadyDispatchedToNewTouchTarget && target == newTouchTarget) {
handled = true;
} else {
final boolean cancelChild = resetCancelNextUpFlag(target.child)
|| intercepted;
//如果当前目标没有被分发事件,为它分发事件
if (dispatchTransformedTouchEvent(ev, cancelChild,
target.child, target.pointerIdBits)) {
handled = true;
}
if (cancelChild) {
if (predecessor == null) {
mFirstTouchTarget = next;
} else {
predecessor.next = next;
}
target.recycle();
target = next;
continue;
}
}
predecessor = target;
target = next;
}
}
// Update list of touch targets for pointer up or cancel, if needed.
//如果需要,更新触摸点抬起(up)和取消(cancel)的触摸目标集合
if (canceled
|| actionMasked == MotionEvent.ACTION_UP
|| actionMasked == MotionEvent.ACTION_HOVER_MOVE) {
resetTouchState();
} else if (split && actionMasked == MotionEvent.ACTION_POINTER_UP) {
final int actionIndex = ev.getActionIndex();
final int idBitsToRemove = 1 << ev.getPointerId(actionIndex);
removePointersFromTouchTargets(idBitsToRemove);
}
}
if (!handled && mInputEventConsistencyVerifier != null) {
mInputEventConsistencyVerifier.onUnhandledEvent(ev, 1);
}
return handled;
}
从上面的源码可知,当ViewGroup不作事件拦截,会遍历ViewGroup的所有子元素,然后判断子元素是否能够接收到事件。是否能够接收到事件主要由两点判断:子元素是否在播放动画和点击事件是否落在子元素的边界范围内。如果某个子元素满足这两个条件,那么事件就会传递给这个子控件来处理。可以看到,dispatchTransformedTouchEvent实际上调用的是子元素的dispatchEvent方法,其内部有一段代码:
if(child == null) {
handled = super.dispatchTouchEvent(event);
} else {
...
handled = child.dispatchTouchEvent(transformedEvent);
}
如果找到了能够接收事件的子View,就会由这个View分发处理事件,否则将当前ViewGroup作为View(ViewGroup的super是View)分发处理该事件。
如果子元素的dispatchTouchEvent返回true,我们就暂时不用考虑事件是怎样在子View中分发的。那么mFirstTouchTarget就会被赋值同时跳出for循环,如下所示:
newTouchTarget = addTouchTarget(child, idBitsToAssign);
alreadyDispatchedToNewTouchTarget = true;
break;
这几行代码完成了mFirstTouchTarget的赋值并终止对子元素的遍历。如果子元素的dispatchTouchEvent返回false,ViewGroup会继续遍历,把事件分发给下一个符合条件的子元素(如果有的话)。
其实mFirstTouchTarget的赋值是在addTouchTarget方法中,从下面addTouchTarget方法的源码中可以看出,mFirstTouchTarget其实是一种单链表结构。mFirstTouchTarget是否被赋值,将直接影响到ViewGroup对事件的拦截策略,如果mFirstTouchTarget为null,那么ViewGroup就默认拦截接下来的同一序列中(down-up、down-move…-up等)的所有触摸事件。
/**
* Adds a touch target for specified child to the beginning of the list.
* Assumes the target child is not already present.
*/
private TouchTarget addTouchTarget(@NonNull View child, int pointerIdBits) {
final TouchTarget target = TouchTarget.obtain(child, pointerIdBits);
target.next = mFirstTouchTarget;
mFirstTouchTarget = target;
return target;
}
如果遍历了所有子元素后,事件没有被合理地处理。这包含两种情况:一,ViewGroup没有子元素;二,子元素处理了事件,但在dispatchTouchEvent中返回了false,这一般是因为子元素在onTouchEvent中返回了false。在这两种情况下,ViewGroup会自己处理点击事件。如下:
// Dispatch to touch targets.
if (mFirstTouchTarget == null) {
// No touch targets so treat this as an ordinary view.
handled = dispatchTransformedTouchEvent(ev, canceled, null,
TouchTarget.ALL_POINTER_IDS);
} else {
......
}
注意dispatchTransformedTouchEvent的第三个参数child为null,从dispatchTransformedTouchEvent方法的分析可知,它会调用super.dispatchTouchEvent(event);,而这里的super是一个View(ViewGroup的super是View),也就是ViewGroup作为View去处理事件,这就转到了View的事件分发。
下面是dispatchTransformedTouchEvent的源码:
//把一个动作事件转换到一个特定子View的坐标空间中,过滤掉不相干的
//触摸点ids,并且在必要时覆盖它的动作(事件)。如果子控件为空,
//动作事件(MotionEvent)将会分发给当前ViewGroup.
/**
* Transforms a motion event into the coordinate space of a particular child view,
* filters out irrelevant pointer ids, and overrides its action if necessary.
* If child is null, assumes the MotionEvent will be sent to this ViewGroup instead.
*/
private boolean dispatchTransformedTouchEvent(MotionEvent event, boolean cancel,
View child, int desiredPointerIdBits) {
final boolean handled;
// Canceling motions is a special case. We don't need to perform any transformations
// or filtering. The important part is the action, not the contents.
//取消动作时一个特殊情况。我们不需要执行任何转换或者过滤。
//它重要的部分是动作(action),不是内容。
final int oldAction = event.getAction();//获取动作
if (cancel || oldAction == MotionEvent.ACTION_CANCEL) {
event.setAction(MotionEvent.ACTION_CANCEL);
if (child == null) {
handled = super.dispatchTouchEvent(event);
} else {
handled = child.dispatchTouchEvent(event);
}
event.setAction(oldAction);
return handled;
}
//计算要分发的触摸点数量。过滤掉不相干的触摸点id。
// Calculate the number of pointers to deliver.
final int oldPointerIdBits = event.getPointerIdBits();
final int newPointerIdBits = oldPointerIdBits & desiredPointerIdBits;
// If for some reason we ended up in an inconsistent state where it looks like we
// might produce a motion event with no pointers in it, then drop the event.
//如果由于一些原因,我们最后处于一个不合逻辑的状态,就好像我们作出一个没有触摸点的动作事件,那么停止事件。
if (newPointerIdBits == 0) {
return false;
}
//动作事件复用
// If the number of pointers is the same and we don't need to perform any fancy
// irreversible transformations, then we can reuse the motion event for this
// dispatch as long as we are careful to revert any changes we make.
// Otherwise we need to make a copy.
//如果两个触摸点的数值一样,并且我们不需要执行任何不可逆的变换,
//那么我们可以复用动作事件(motion event)来进行分发,只要
//我们注意恢复我们做出的改变。
final MotionEvent transformedEvent;
if (newPointerIdBits == oldPointerIdBits) {
if (child == null || child.hasIdentityMatrix()) {
if (child == null) {
handled = super.dispatchTouchEvent(event);
} else {
final float offsetX = mScrollX - child.mLeft;
final float offsetY = mScrollY - child.mTop;
event.offsetLocation(offsetX, offsetY);
handled = child.dispatchTouchEvent(event);
event.offsetLocation(-offsetX, -offsetY);
}
return handled;
}
transformedEvent = MotionEvent.obtain(event);
} else {
transformedEvent = event.split(newPointerIdBits);
}
//执行任何必要的转换和事件分发。
// Perform any necessary transformations and dispatch.
if (child == null) {
//如果没找到接收事件的子类,就由ViewGroup(ViewGroup的super是View)自己作为View分发处理事件。
handled = super.dispatchTouchEvent(transformedEvent);
} else {如果有接收事件的子View,坐标空间转换后,事件由子View分发处理。
final float offsetX = mScrollX - child.mLeft;
final float offsetY = mScrollY - child.mTop;
transformedEvent.offsetLocation(offsetX, offsetY);
if (! child.hasIdentityMatrix()) {
transformedEvent.transform(child.getInverseMatrix());
}
handled = child.dispatchTouchEvent(transformedEvent);
}
// Done.
transformedEvent.recycle();
return handled;
}
Activity对事件的分发过程
点击事件用MotionEvent来表示,当一个点击操作发生时,事件首先传递给当前Activity,由Activity的dispatchTouchEvent来进行事件分发,具体工作是由Activity内部的Window来完成的。Window会将事件传递给DecorView,DecorView一般是当前界面的底层容器(也就是setContentView所设置View的父容器),通过activity.getWindow().getDecorView()可以获得。先从Activity的dispatchTouchEvent开始分析:
public boolean dispatchTouchEvent(MotionEvent ev) {
if (ev.getAction() == MotionEvent.ACTION_DOWN) {
onUserInteraction();
}
if (getWindow().superDispatchTouchEvent(ev)) {
return true;
}
return onTouchEvent(ev);
}
分析一下源码,发现事件首先被交给Activity所附属的Window进行分发,如果返回true,整个事件分发过程结束,如果返回false,意味着事件没有控件处理,那么Activity的onTouchEvent就会被调用。
接下来看Window是如何将事件传递给ViewGroup的。通过源码我们知道,Window是一个抽象类,而Window的superDispatchTouchEvent是个抽象方法,因此必须找到Window的实现类才行。
Window的实现类到底是谁?其实是PhoneWindow,这一点从Window的源码可以知道,在Window源码的类注释中有这么一段话:
Abstract base class for a top-level window look and behavior policy. An
instance of this class should be used as the top-level view added to the
window manager. It provides standard UI policies such as a background, title
area, default key processing, etc.
<p>The only existing implementation of this abstract class is
android.view.PhoneWindow, which you should instantiate when needing a
Window.
大概意思是:Window类可以控制顶层View的外观和行为策略。它的唯一实现是android.policy.PhoneWindow,当你想要实例化这个Window类时,你并不 知道它的细节,因为这个类会被重构,只有一个工厂方法可以使用。
由于Window的唯一实现是PhoneWindow,那么就看一下PhoneWindow是怎样处理事件的。也就是看一下superDispatchTouchEvent方法:
@Override
public boolean superDispatchTouchEvent(MotionEvent event) {
return mDecor.superDispatchTouchEvent(event);
}
到这里就很清楚了,PhoneWindow将事件传递给了DecorView,这个DecorView是什么呢?看下面:
// This is the top-level view of the window, containing the window decor.
private DecorView mDecor;
@Override
public final View getDecorView() {
if (mDecor == null || mForceDecorInstall) {
installDecor();
}
return mDecor;
}
我们可以通过getWindow().getDecorView().findViewById(R.id.content).getChildAt(0)获取Activity通过所设置的View。这个mDecor就是getWindow().getDecorView()返回的View,而我们通过setContentView设置的View是它的一个子View。现在事件传递到了DecorView这里,由于DecorView继承自FrameLayout,且是父View,所以最终事件会传递给setContentView设置View。从这里开始,事件就传递到顶级View了,也就是Activity中通过setContentView设置View,另外顶级View也叫根View,顶级View一般都是ViewGroup。
这样就与上面ViewGroup的事件分发连接上了。
借用何俊林大神(逆流的鱼yuiop)的一张图片,来展示ViewGroup的事件分发流程。
ViewGroup事件分发试验
code
与博客最开始一样,自定义一个MyLayout,继承LinearLayout。重写三个方法:onInterceptTouchEvent、onTouchEvent、dispatchTouchEvent,代码如下:
public class MyLayout extends LinearLayout {
public MyLayout(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
}
@Override
public boolean onInterceptTouchEvent(MotionEvent ev) {
Log.i("TAG", "MyLayout: onInterceptTouchEvent === " + getActionString(ev.getAction()));
return super.onInterceptTouchEvent(ev);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
Log.i("TAG", "MyLayout: onTouchEvent === " + getActionString(event.getAction()));
return super.onTouchEvent(event);
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
Log.i("TAG", "MyLayout: dispatchTouchEvent === " + getActionString(ev.getAction()));
return super.dispatchTouchEvent(ev);
}
private String getActionString(int action){
switch (action) {
case MotionEvent.ACTION_DOWN:
return "ACTION_DOWN";
case MotionEvent.ACTION_MOVE:
return "ACTION_MOVE";
case MotionEvent.ACTION_UP:
return "ACTION_UP";
case MotionEvent.ACTION_CANCEL:
return "ACTION_CANCEL";
default: return "ACTION_OTHER";
}
}
}
然后,自定义一个MButton,继承Button,重写两个方法onTouchEvent、dispatchTouchEvent,代码如下:
public class MButton extends android.support.v7.widget.AppCompatButton {
public MButton(Context context) {
super(context);
}
public MButton(Context context, AttributeSet attrs) {
super(context, attrs);
}
public MButton(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
@Override
public boolean dispatchTouchEvent(MotionEvent event) {
Log.i("TAG", "MButton: dispatchTouchEvent === " + getActionString(event.getAction()));
return super.dispatchTouchEvent(event);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
Log.i("TAG", "MButton: onTouchEvent === " + getActionString(event.getAction()));
return super.onTouchEvent(event);
}
private String getActionString(int action){
switch (action) {
case MotionEvent.ACTION_DOWN:
return "ACTION_DOWN";
case MotionEvent.ACTION_MOVE:
return "ACTION_MOVE";
case MotionEvent.ACTION_UP:
return "ACTION_UP";
case MotionEvent.ACTION_CANCEL:
return "ACTION_CANCEL";
default: return "ACTION_OTHER";
}
}
}
在布局文件activity_view_group.xml中引用MyLayout和MButton,如下所示:
<?xml version="1.0" encoding="utf-8"?>
<com.wzhy.dispatcheventdemo.MyLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/my_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.wzhy.dispatcheventdemo.MButton
android:id="@+id/m_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="MButton"
/>
</com.wzhy.dispatcheventdemo.MyLayout>
最后,在ActivityViewGroup中为MyLayout和MButton设置onTouchListener,重写Activity的onTouchEvent、dispatchTouchEvent。代码如下:
public class ActivityViewGroup extends AppCompatActivity {
private MyLayout mLayout;
private MButton mButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_view_group);
mLayout = (MyLayout) findViewById(R.id.my_layout);
mButton = (MButton) findViewById(R.id.m_button);
mLayout.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
Log.i("TAG", "MyLayout: onTouch === " + getActionString(event.getAction()));
return false;
}
});
mButton.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
Log.i("TAG", "MButton: onTouch === " + getActionString(event.getAction()));
return false;
}
});
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
String actionString = getActionString(ev.getAction());
Log.i("TAG", "Activity: dispatchTouchEvent === " + actionString);
return super.dispatchTouchEvent(ev);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
String actionString = getActionString(event.getAction());
Log.i("TAG", "Activity: onTouchEvent === " + actionString);
return super.onTouchEvent(event);
}
private String getActionString(int action){
switch (action) {
case MotionEvent.ACTION_DOWN:
return "ACTION_DOWN";
case MotionEvent.ACTION_MOVE:
return "ACTION_MOVE";
case MotionEvent.ACTION_UP:
return "ACTION_UP";
case MotionEvent.ACTION_CANCEL:
return "ACTION_CANCEL";
default: return "ACTION_OTHER";
}
}
}
代码完成后,运行!接下来进行试验。
试验
手指点击一下手机屏幕中的按钮
log打印如下:
运用之前的分析结果,可解释为:
手指按下
ActivityViewGroup
· 接收到DOWN事件
· 调用dispatchTouchEvent分发事件(①Activity: dispatchTouchEvent)
- - - - - - - - - - - - - - - -
Activity会将事件传递给顶层View——DecorView ,DecorView 继承了FrameLayout。DecorView默认不拦截事件,会继续向下分发事件,最后事件进入我们的根布局MyLayout
- - - - - - - - - - - - - - - -
MyLayout
· 接收到DOWN事件
· 调用dispatchTouchEvent进行事件分发(②MyLayout: dispatchTouchEvent)
· 调用onInterceptTouchEvent,默认不拦截事件(③MyLayout: onInterceptTouchEvent)
· 向下遍历查找触摸点所在位置可接收事件的子View,找到了MButton,将事件传递给MButton
- - - - - - - - - - - - - - - -
MyButton
· 接收到DOWN事件
· 调用dispatchTouchEvent进行事件分发(④MButton: dispatchTouchEvent)
· 先进入触摸监听的重写方法onTouch(⑤MButton: onTouch),返回false
· 后进入onTouchEvent最终处理事件(⑥MButton: onTouchEvent)
手指抬起(UP)
与DOWN事件一样,从外向内传递事件,最终由被点击的MButton的onTouchEvent将事件处理。
手指点击一下空白处
按照我们之前的分析,点击空白处,MyLayout没有子View去接收事件,事件就由MyLayout(作为View)处理事件。到底是不是呢?看一下log打印验证一下:
从上面log打印第4行看,这里走了MyLayout设置的onTouchListener监听的方法onTouch,这个方法返回默认值false,接着走了MyLayout的onTouchEvent, 根据上一篇笔记:事件分发机制(一):View的事件分发,我们知道,当一个控件不可点击时,在View的onTouchEvent中不会处理action,会返回false。onTouchEvent返回false,那么MyLayout(此时作为View)的dispatchTouchEvent也会返回false,由View的dispatchTouchEvent中的代码
//noinspection SimplifiableIfStatement
ListenerInfo li = mListenerInfo;
if (li != null && li.mOnTouchListener != null
&& (mViewFlags & ENABLED_MASK) == ENABLED
&& li.mOnTouchListener.onTouch(this, event)) {
result = true;
}
if (!result && onTouchEvent(event)) {
result = true;
}
可知,onTouchEvent(event)返回false,result就不会赋true值(见上面代码最后三行),那么dispatchTouchEvent就返回false,那么MyLayout的dispatchTouchEvent就返回false,也就是说MyLayout也处理不了这个点击事件。
那么最后就会传递给(其实先交给了DecorView,逻辑与MyLayout应该相同,最后传递给Activity处理)Activity的onTouchEvent处理,最终Activity作为处理事件的对象,之后的一序列动作(down之后的move和up)都将由Activity的onTouchEvent处理。
如果我们给MyLayout的布局中①设置android:clickable="true",或②在onTouch中返回true,事件就会交由MyLayout处理。运行后拿到log打印验证一下:
- ①设置android:clickable="true" (或 在onTouchEvent中返回true)
- ②在onTouch中返回true
ViewGroup拦截事件
首先将上面的设置恢复。
从上面的log打印发现,事件由Activity的dispatchTouchEvent接收,并分发到MyLayout的dispatchTouchEvent,在MyLayout开始分发后先调用了onInterceptTouchEvent,然后再继续事件分发。那么,我们就再次尝试一下事件拦截,看一看log打印结果。
将MyLayout的onInterceptTouchEvent返回值改为true,如下
@Override
public boolean onInterceptTouchEvent(MotionEvent ev) {
Log.i("TAG", "MyLayout: onInterceptTouchEvent === " + getActionString(ev.getAction()));
super.onInterceptTouchEvent(ev);
return true;
}
运行后,分别点击按钮和空白区域,发现log打印一样
可以解释为:
·> 事件传递到Activity的dispatchTouchEvent;
·> 分发给MyLayout的dispatchTouchEvent;
·> 先经过MyLayout拦截onInterceptTouchEvent,返回true,事件被拦截;
·> MyLayout作为View来处理事件,事件先经过onTouch,返回false,
·> 后传递到MyLayout的onTouchEvent;
·> 由于MyLayout默认不可点击,MyLayout的onTouchEvent无法处理事件,会返回false,事件没有被MyLayout处理,dispatchTouchEvent返回false;
·> 最终,事件由Activity在它的onTouchEvent中处理,后续的move、up等一序列事件都在Activity的onTouchEvent中处理。
MyLayout有事件拦截的时候,点击空白区域的流程和点击按钮的流程是一样的。
我们想让MyLayout处理事件,那就需要让MyLayout是clickable,或让onTouchEvent处理过事件返回true,或由MyLayout的onTouch处理过事件后返回true。如果直接由dispatchTouchEvent处理事件后返回true,那么事件拦截接方法onInterceptTouchEvent也不会走了。
我们来看一下log打印验证 一下!
-①设置android:clickable="true" (或 在onTouchEvent中返回true)
- ②在onTouch中返回true
- ③直接在dispatchTouchEvent处理事件后返回true
注意: 直接在MyLayout(ViewGroup)的onTouch中返回true,或在onTouchEvent中返回true,或让它clickable,MyLayout是不能获得事件并执行的;除非事件被MyLayout拦截(onInterceptTouchEvent返回true),或在点击处没找到能够处理事件的子View(包括点击空白处),MyLayout才作为View去处理事件,先经过onTouch(如果返回true,表示事件被onTouch处理,不再经过onTouchEvent),再经过onTouchEvent。
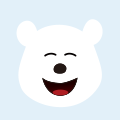