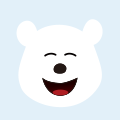
回复
公司列表页面是用户查看公司信息的重要功能模块。以下是一个完整的公司列表页面实现,包括公司信息的展示和点击跳转到公司详情页的功能。
CompanyList
的公司列表组件。@State
装饰器定义了companyModel
,用于存储公司列表数据。companyurl
,用于存储获取公司信息的接口URL。aboutToAppear
生命周期函数中,调用httpRequestGet
方法获取公司列表数据,并根据返回结果更新companyModel
或显示提示信息。Column
和Row
布局组件,构建公司列表页面的UI。List
和ForEach
组件,遍历companyModel
,为每个公司生成一个列表项。router.pushUrl
跳转到公司详情页,并传递公司ID作为参数。通过上述代码,我们实现了一个完整的公司列表页面,包括公司信息的展示和点击跳转到公司详情页的功能。用户可以通过公司列表页面查看各个公司的基本信息,并通过点击进入公司详情页获取更多详细信息。