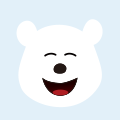
第十二课:HarmonyOS Next多设备适配与响应式开发终极指南 原创
HarmonyOS Next多设备适配与响应式开发终极指南
一、设备矩阵适配策略
1. 设备类型分级规范
// build-profile.json5配置
"targetDevices": {
"mandatory": ["phone", "tablet"], // 强制适配设备
"optional": ["tv", "watch"], // 可选适配设备
"exclude": ["car"] // 排除设备类型
}
2. 分辨率动态分级方案
// 基于屏幕逻辑宽度(vp)的响应式断点
const Breakpoints = {
SMALL: 320, // 智能手表
MEDIUM: 600, // 折叠屏手机(展开)
LARGE: 840 // 平板设备
};
@Builder function AdaptiveLayout() {
if ($r('sw') < Breakpoints.SMALL) {
Column() { /* 手表布局 */ }
} else if ($r('sw') >= Breakpoints.LARGE) {
Grid() { /* 平板布局 */ }
} else {
Row() { /* 手机布局 */ }
}
}
3. 输入方式智能适配
// 混合输入事件处理
@Component
struct InputAdaptor {
@State touchActive: boolean = false;
build() {
Button('操作按钮')
.onTouch((event) => {
this.touchActive = true;
})
.onKeyEvent((event) => {
if (event.keyCode === 10009) { // 车机旋钮确认键
this.handleConfirm();
}
})
}
}
二、响应式布局三大核心方案
1. 栅格系统(Grid System)
// 12列栅格布局模板
@Entry
@Component
struct GridDemo {
build() {
GridRow({ columns: 12, gutter: 8 }) {
GridCol({ span: { xs: 12, sm: 6, md: 4 } }) { /* 响应式列宽 */ }
GridCol({ offset: 2, span: { xs: 12, sm: 4 } }) { /* 动态偏移 */ }
}
.breakpoints({
xs: 320, // < 600vp
sm: 600, // ≥600vp
md: 840 // ≥840vp
})
}
}
2. 容器查询布局
// 根据父容器尺寸动态调整
@Component
struct ContainerQueryDemo {
@BuilderParam adaptLayout: BuilderParam;
build() {
Column() {
this.adaptLayout()
}
.size({ width: '100%', height: '100%' })
.onAreaChange((oldValue, newValue) => {
if (newValue.width < 300) {
this.adaptLayout = this.buildCompactView;
} else {
this.adaptLayout = this.buildExpandedView;
}
})
}
}
3. 原子化自适应样式
// 创建自适应样式变量
@Styles function adaptivePadding() {
.padding({
top: $r('sw') >= 840 ? 24 : 12,
bottom: $r('sw') >= 840 ? 24 : 12,
left: $r('sw') >= 600 ? 16 : 8,
right: $r('sw') >= 600 ? 16 : 8
})
}
// 应用样式
Text('内容区块')
.adaptivePadding()
.fontSize($r('sw') >= 840 ? 20 : 14)
三、开发效率提升方案
1. 多设备协同调试
# 启动设备矩阵模拟器
hdc emulator start --devices phone,watch,car
2. 布局热重载配置
// tsconfig.json
{
"compilerOptions": {
"enableHotReload": true,
"hotReloadWhitelist": ["./src/layout/**/*"]
}
}
3. 设计稿智能转换
/*
1. 导入Figma/Sketch设计稿
2. 识别布局结构和约束关系
3. 输出ArkUI自适应组件
*/
四、企业级适配最佳实践
1. 设备能力分级策略
能力等级 | 设备类型 | 功能要求 |
Tier 1 | 旗舰手机/平板 | 完整功能+3D效果 |
Tier 2 | 中端设备/车机 | 核心功能+基础动效 |
Tier 3 | 智能穿戴/旧款设备 | 精简功能+静态布局 |
2. 性能优化指标
// 布局渲染性能检测
Profiler.trackLayout(() => {
/* 布局代码块 */
}, {
reportType: 'detail',
threshold: 16.6 // 60FPS标准帧时间(ms)
})
3. 多语言布局反向适配
// 处理从右向左布局(RTL)
Flex({ direction: FlexDirection.Row }) {
if (I18n.isRTL()) {
ChildComponent().margin({ right: 16 })
} else {
ChildComponent().margin({ left: 16 })
}
}
五、常见问题解决方案
Q1:折叠屏展开/折叠状态检测
// 屏幕状态监听
screen.getDefaultDisplay().on('foldStatusChange', (status) => {
if (status === screen.FoldStatus.FOLD_STATUS_EXPANDED) {
this.currentLayoutMode = LayoutMode.EXPANDED;
}
});
Q2:车机高对比度模式适配
// 颜色方案自动切换
@State colorScheme: ColorScheme = ColorScheme.LIGHT;aboutToAppear() {
Accessibility.subscribeColorSchemeChange((scheme) => {
this.colorScheme = scheme;
});
}
Q3:手表圆形屏幕裁切处理
// 圆形界面适配方案
Canvas.create({
type: 'watch',
shape: CanvasShape.CIRCLE
})
.component({
layout: (ctx) => {
// 自动处理内容裁切区域
}
})
前沿技术展望:
- AI预测布局:基于设备使用习惯预加载界面模块
- 3D空间自适应:适配AR眼镜等空间计算设备
- 神经渲染技术:实时生成分辨率无关的矢量图形
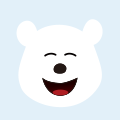