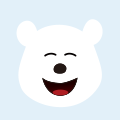
(三四)ArkTS 小程序开发实战 原创
一、引言
在移动应用生态中,小程序凭借其独特优势,成为众多企业和开发者触达用户的重要途径。ArkTS 作为一种新兴且功能强大的开发语言,为小程序开发带来了新的活力与高效体验。本文将全面深入地介绍如何利用 ArkTS 进行小程序开发实战,涵盖从开发概述到最终发布推广的全流程,并提供丰富具体的代码示例,助力开发者快速上手。
二、小程序开发概述
2.1 小程序的优势与市场需求
小程序具有无需安装、即点即用的特点,大大降低了用户使用应用的门槛。对于企业而言,开发小程序成本相对较低,能快速迭代更新,且可通过多种渠道触达用户,如社交平台分享、线下扫码等。在如今快节奏的生活中,用户对于便捷获取服务的需求日益增长,小程序正好满足了这一需求,无论是在电商购物、生活服务,还是资讯阅读等领域都得到了广泛应用,市场需求持续攀升。
2.2 ArkTS 开发小程序的流程
首先,需要安装好开发环境,如下载并安装支持 ArkTS 开发的小程序开发工具。创建新项目时,在开发工具中选择 ArkTS 作为开发语言,填写项目名称、描述等基本信息后,即可生成项目框架。项目框架中包含了小程序的基础配置文件、页面文件以及资源文件等。接着,根据项目需求进行页面设计、功能实现等开发工作,完成开发后进行本地调试,确保小程序在不同设备和场景下运行正常,最后提交审核并发布上线。
三、小程序界面设计与交互
3.1 小程序风格与布局
ArkTS 提供了丰富的组件和布局方式来构建小程序界面。例如,使用 Flex 布局来实现灵活的页面排版。假设我们要创建一个简单的商品展示页面:
@Entry
@Component
struct GoodsShowPage {
build() {
Flex({ direction: FlexDirection.Row, justifyContent: FlexAlign.SpaceAround }) {
ForEach([1, 2, 3], (index) => {
Card() {
Image($r('app.media.goods' + index))
.width(150).height(150).objectFit(ImageFit.Cover)
Text('Goods ' + index)
.fontSize(16).textAlign(TextAlign.Center)
}
.width(180).height(220).shadow({ color: '#ccc', radius: 5 })
}, (index) => index.toString())
}
.width('100%').padding(20)
}
}
在上述代码中,通过 Flex 布局将商品图片和名称进行整齐排列,FlexDirection.Row表示水平方向排列,FlexAlign.SpaceAround使元素之间均匀分布。Card组件用于为每个商品项添加一个带阴影的卡片效果,增强视觉层次感。
3.2 交互效果实现
交互效果能提升用户体验。比如实现一个按钮点击变色的交互效果:
@Entry
@Component
struct ButtonInteraction {
private isClicked: boolean = false;
build() {
Button('Click Me')
.backgroundColor(this.isClicked? '#FF5733' : '#3399FF')
.width(150).height(50).fontSize(16)
.onClick(() => {
this.isClicked =!this.isClicked;
})
}
}
当用户点击按钮时,isClicked状态发生改变,从而动态切换按钮的背景颜色,给用户直观的反馈。
四、小程序功能实现
4.1 数据获取与展示
通常小程序需要从后端服务器获取数据并展示。假设我们有一个获取用户列表数据的接口,使用@ohos.net.http模块来请求数据并展示:
import http from '@ohos.net.http';
@Entry
@Component
struct UserListPage {
private userList: Array<{ name: string, age: number }> = [];
async onInit() {
const request = http.createHttp();
request.requestUrl = 'https://example.com/api/userlist';
request.requestMethod = http.RequestMethod.GET;
const response = await request.request();
if (response.statusCode === 200) {
this.userList = JSON.parse(response.responseText);
} else {
console.error('Failed to get user list:', response.statusCode);
}
}
build() {
List() {
ForEach(this.userList, (user) => {
Row() {
Text(user.name).fontSize(16).width('50%')
Text(user.age.toString()).fontSize(16).width('50%')
}
.height(40).padding(10).borderBottom({ width: 1, color: '#ccc' })
}, (user) => user.name)
}
.width('100%')
}
}
在onInit生命周期函数中发起 HTTP 请求,获取数据后解析并存储在userList数组中,然后通过ForEach组件将用户数据展示在列表中。
4.2 表单提交与验证
以一个简单的登录表单为例,实现表单提交与验证功能:
@Entry
@Component
struct LoginForm {
private username: string = '';
private password: string = '';
private errorMessage: string = '';
validateForm() {
if (this.username === '') {
this.errorMessage = 'Username cannot be empty';
return false;
}
if (this.password === '') {
this.errorMessage = 'Password cannot be empty';
return false;
}
this.errorMessage = '';
return true;
}
async submitForm() {
if (this.validateForm()) {
// 模拟提交数据到服务器
const request = http.createHttp();
request.requestUrl = 'https://example.com/api/login';
request.requestMethod = http.RequestMethod.POST;
request.requestBody = { username: this.username, password: this.password };
const response = await request.request();
if (response.statusCode === 200) {
console.log('Login successful');
} else {
console.error('Login failed:', response.statusCode);
}
}
}
build() {
Column() {
TextField({ placeholder: 'Username' })
.value(this.username)
.onChange((value: string) => {
this.username = value;
})
TextField({ placeholder: 'Password', password: true })
.value(this.password)
.onChange((value: string) => {
this.password = value;
})
if (this.errorMessage!== '') {
Text(this.errorMessage).fontSize(14).textColor('#FF0000')
}
Button('Login')
.width(150).height(50).fontSize(16)
.onClick(() => {
this.submitForm();
})
}
.width('100%').padding(20)
}
}
在validateForm方法中对用户名和密码进行非空验证,若验证通过则在submitForm方法中发起登录请求,同时在页面上实时展示错误信息。
五、小程序发布与推广
完成小程序开发与测试后,在开发工具中进行代码打包,生成提交审核的文件。提交审核时,需填写小程序的基本信息、业务介绍等内容,确保符合平台的审核规则。审核通过后,小程序即可正式发布上线。
推广方面,可以利用社交媒体平台进行分享,如在微信、微博等平台发布小程序相关的介绍文章、海报等,吸引用户点击使用。还可以与线下商家合作,通过扫码优惠等活动引导用户使用小程序。此外,优化小程序的关键词,提高在小程序搜索中的排名,也是增加曝光度的有效方式。
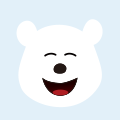