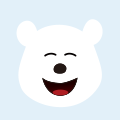
回复
支持横向、纵向双向滑动的列表控件。实现思路是:
1、在封装的控件内实现首行布局,第一列为固定列,不可滑动,其它列通过Scroll组件包裹,实现横向滑动。
2、数据列通过List组件包裹,实现纵向滑动。数据通过LazyForEach实现懒加载,行布局第一列为固定列,不可滑动,其它列通过Scroll组件包裹,实现横向滑动。为了APP可以自定义数据列样式,因此通过定义Builder参数,由调用者动态传入,在调用方实现数据列的布局。
技术细节
1、定义表头单元格布局
2、定义表头行
3、构造数据列表
双向滑动列表的实现,需要使用复杂的手势处理。感兴趣的朋友可以参考@sunshine/multilist · git_zhaoyang/MultiList - 码云 - 开源中国