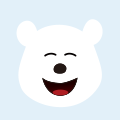
回复
这是一个Flutter插件平台接口项目,用于统一管理屏幕亮度的相关操作。它定义了与平台无关的接口,具体实现由各个平台(Android、iOS、macOS、Windows等)完成。
在ScreenBrightnessPlatform
类中定义了以下主要接口:
系统亮度相关
system
: 获取系统亮度(0.0-1.0)setSystemScreenBrightness()
: 设置系统亮度onSystemScreenBrightnessChanged
: 监听系统亮度变化应用亮度相关
application
: 获取应用亮度setApplicationScreenBrightness()
: 设置应用亮度resetApplicationScreenBrightness()
: 重置应用亮度onApplicationScreenBrightnessChanged
: 监听应用亮度变化hasApplicationScreenBrightnessChanged
: 判断应用亮度是否被修改其他功能
isAutoReset
: 是否自动重置亮度setAutoReset()
: 设置自动重置isAnimate
: 是否启用动画setAnimate()
: 设置动画canChangeSystemBrightness
: 是否可以修改系统亮度在鸿蒙侧实现时,需要关注:
MethodChannel通信
pluginMethodChannel
与Flutter端通信method_name.dart
中plugin_channel.dart
中亮度范围
RangeError
异常处理
PlatformException
生命周期管理
autoReset
功能在ScreenBrightnessPlugin.ets
中,你可以:
ScreenBrightnessPlatform
接口Window
API获取和设置亮度可以参考screen_brightness_platform_interface_test.dart
中的测试用例,确保实现的功能符合预期。
需要我详细解释某个部分或提供具体实现示例吗?
核心的亮度控制功能。
核心功能
@ohos.window
模块进行亮度控制错误处理
MethodChannel通信
MethodChannel
与Flutter端进行通信窗口管理
getLastWindow()
获取当前窗口