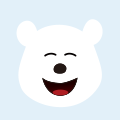
(三八)HarmonyOS Design 的安全性:数据加密与网络安全实践 原创
HarmonyOS Design 的安全性:数据加密与网络安全实践
引言
在数字化时代,安全性是操作系统设计中至关重要的一环。HarmonyOS 作为一款先进的分布式操作系统,高度重视安全性,为用户的数据和隐私保驾护航。本文将聚焦于 HarmonyOS Design 中的安全性,详细探讨数据加密与安全存储的方法,以及网络安全的最佳实践,并附上相关代码示例。
数据加密与安全存储
数据加密算法选择
HarmonyOS 支持多种加密算法,如 AES(高级加密标准)、RSA(非对称加密算法)等。开发者可以根据具体需求选择合适的加密算法。
AES 加密示例
AES 是一种对称加密算法,具有高效、安全的特点,常用于对大量数据的加密。以下是一个使用 AES 进行数据加密和解密的示例代码:
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.nio.charset.StandardCharsets; import java.util.Base64; public class AESEncryption { private static final String ALGORITHM = "AES"; private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding"; public static String encrypt(String plainText, String key) throws Exception { SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM); Cipher cipher = Cipher.getInstance(TRANSFORMATION); cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec); byte[] encryptedBytes = cipher.doFinal(plainText.getBytes(StandardCharsets.UTF_8)); return Base64.getEncoder().encodeToString(encryptedBytes); } public static String decrypt(String encryptedText, String key) throws Exception { SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM); Cipher cipher = Cipher.getInstance(TRANSFORMATION); cipher.init(Cipher.DECRYPT_MODE, secretKeySpec); byte[] decodedBytes = Base64.getDecoder().decode(encryptedText); byte[] decryptedBytes = cipher.doFinal(decodedBytes); return new String(decryptedBytes, StandardCharsets.UTF_8); } public static void main(String[] args) { try { String plainText = "HarmonyOS安全加密示例"; String key = "1234567890123456"; // AES密钥长度必须为16、24或32字节 String encryptedText = encrypt(plainText, key); String decryptedText = decrypt(encryptedText, key); System.out.println("原始文本: " + plainText); System.out.println("加密后文本: " + encryptedText); System.out.println("解密后文本: " + decryptedText); } catch (Exception e) { e.printStackTrace(); } } }
- 1.
RSA 加密示例
RSA 是一种非对称加密算法,常用于对少量数据(如密钥)的加密和数字签名。以下是一个使用 RSA 进行加密和解密的示例代码:
import javax.crypto.Cipher; import java.nio.charset.StandardCharsets; import java.security.*; import java.security.spec.PKCS8EncodedKeySpec; import java.security.spec.X509EncodedKeySpec; import java.util.Base64; public class RSAEncryption { private static final String ALGORITHM = "RSA"; public static KeyPair generateKeyPair() throws Exception { KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(ALGORITHM); keyPairGenerator.initialize(2048); return keyPairGenerator.generateKeyPair(); } public static String encrypt(String plainText, PublicKey publicKey) throws Exception { Cipher cipher = Cipher.getInstance(ALGORITHM); cipher.init(Cipher.ENCRYPT_MODE, publicKey); byte[] encryptedBytes = cipher.doFinal(plainText.getBytes(StandardCharsets.UTF_8)); return Base64.getEncoder().encodeToString(encryptedBytes); } public static String decrypt(String encryptedText, PrivateKey privateKey) throws Exception { Cipher cipher = Cipher.getInstance(ALGORITHM); cipher.init(Cipher.DECRYPT_MODE, privateKey); byte[] decodedBytes = Base64.getDecoder().decode(encryptedText); byte[] decryptedBytes = cipher.doFinal(decodedBytes); return new String(decryptedBytes, StandardCharsets.UTF_8); } public static void main(String[] args) { try { KeyPair keyPair = generateKeyPair(); PublicKey publicKey = keyPair.getPublic(); PrivateKey privateKey = keyPair.getPrivate(); String plainText = "HarmonyOS RSA加密示例"; String encryptedText = encrypt(plainText, publicKey); String decryptedText = decrypt(encryptedText, privateKey); System.out.println("原始文本: " + plainText); System.out.println("加密后文本: " + encryptedText); System.out.println("解密后文本: " + decryptedText); } catch (Exception e) { e.printStackTrace(); } } }
- 1.
安全存储
在 HarmonyOS 中,对于敏感数据的存储,应采用安全的方式,如使用加密文件系统或数据库加密。
加密文件存储示例
import ohos.data.rdb.RdbStore; import ohos.data.rdb.StoreConfig; import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.nio.charset.StandardCharsets; import java.util.Base64; public class SecureFileStorage { private static final String ALGORITHM = "AES"; private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding"; public static void encryptAndSaveFile(String plainText, String filePath, String key) throws Exception { SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM); Cipher cipher = Cipher.getInstance(TRANSFORMATION); cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec); byte[] encryptedBytes = cipher.doFinal(plainText.getBytes(StandardCharsets.UTF_8)); FileOutputStream fos = new FileOutputStream(new File(filePath)); fos.write(encryptedBytes); fos.close(); } public static String readAndDecryptFile(String filePath, String key) throws Exception { FileInputStream fis = new FileInputStream(new File(filePath)); byte[] encryptedBytes = new byte[fis.available()]; fis.read(encryptedBytes); fis.close(); SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(StandardCharsets.UTF_8), ALGORITHM); Cipher cipher = Cipher.getInstance(TRANSFORMATION); cipher.init(Cipher.DECRYPT_MODE, secretKeySpec); byte[] decryptedBytes = cipher.doFinal(encryptedBytes); return new String(decryptedBytes, StandardCharsets.UTF_8); } public static void main(String[] args) { try { String plainText = "HarmonyOS安全文件存储示例"; String filePath = "/data/secure_file.txt"; String key = "1234567890123456"; encryptAndSaveFile(plainText, filePath, key); String decryptedText = readAndDecryptFile(filePath, key); System.out.println("原始文本: " + plainText); System.out.println("解密后文本: " + decryptedText); } catch (Exception e) { e.printStackTrace(); } } }
- 1.
网络安全的最佳实践
使用 HTTPS 协议
在进行网络通信时,应优先使用 HTTPS 协议,它通过 SSL/TLS 加密技术对数据进行加密传输,防止数据在传输过程中被窃取或篡改。
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import javax.net.ssl.HttpsURLConnection; public class HttpsExample { public static void main(String[] args) { try { URL url = new URL("https://www.example.com"); HttpsURLConnection connection = (HttpsURLConnection) url.openConnection(); connection.setRequestMethod("GET"); BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String line; StringBuilder response = new StringBuilder(); while ((line = reader.readLine()) != null) { response.append(line); } reader.close(); System.out.println("响应内容: " + response.toString()); } catch (Exception e) { e.printStackTrace(); } } }
- 1.
输入验证与过滤
在接收用户输入或网络数据时,要进行严格的输入验证和过滤,防止 SQL 注入、XSS 攻击等安全漏洞。
示例代码
import java.util.regex.Pattern; public class InputValidation { private static final Pattern SAFE_INPUT_PATTERN = Pattern.compile("^[a-zA-Z0-9]+$"); public static boolean isValidInput(String input) { return SAFE_INPUT_PATTERN.matcher(input).matches(); } public static void main(String[] args) { String input = "abc123"; if (isValidInput(input)) { System.out.println("输入有效"); } else { System.out.println("输入无效"); } } }
- 1.
防止中间人攻击
使用证书验证机制,确保与合法的服务器进行通信。在使用 HTTPS 时,客户端会验证服务器的证书,防止中间人攻击。
import javax.net.ssl.*; import java.io.InputStream; import java.security.KeyStore; import java.security.cert.CertificateFactory; import java.security.cert.X509Certificate; public class SSLVerification { public static void main(String[] args) { try { // 加载服务器证书 InputStream certStream = SSLVerification.class.getResourceAsStream("/server.crt"); CertificateFactory certFactory = CertificateFactory.getInstance("X.509"); X509Certificate cert = (X509Certificate) certFactory.generateCertificate(certStream); // 创建TrustManager KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType()); keyStore.load(null, null); keyStore.setCertificateEntry("server", cert); TrustManagerFactory trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm()); trustManagerFactory.init(keyStore); TrustManager[] trustManagers = trustManagerFactory.getTrustManagers(); // 创建SSLContext SSLContext sslContext = SSLContext.getInstance("TLS"); sslContext.init(null, trustManagers, null); // 创建HttpsURLConnection URL url = new URL("https://www.example.com"); HttpsURLConnection connection = (HttpsURLConnection) url.openConnection(); connection.setSSLSocketFactory(sslContext.getSocketFactory()); // 进行网络请求 BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String line; StringBuilder response = new StringBuilder(); while ((line = reader.readLine()) != null) { response.append(line); } reader.close(); System.out.println("响应内容: " + response.toString()); } catch (Exception e) { e.printStackTrace(); } } }
- 1.
结论
HarmonyOS Design 通过提供丰富的加密算法和安全机制,为开发者构建安全的应用提供了有力支持。在数据加密与安全存储方面,合理选择加密算法和存储方式可以有效保护用户数据的安全。在网络安全方面,遵循最佳实践,如使用 HTTPS 协议、进行输入验证和防止中间人攻击等,可以抵御各种网络攻击,确保应用的安全性。开发者应充分利用这些安全特性,为用户打造更加安全可靠的 HarmonyOS 应用。
微信扫码分享
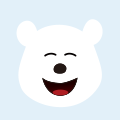