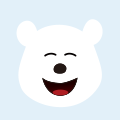
(五一)物流应用的性能优化:操作流程与开发优化技巧 原创
物流应用的性能优化:操作流程与开发优化技巧
引言
在物流行业数字化转型的浪潮中,物流应用已成为提升物流效率、优化服务质量的关键工具。从订单管理、仓储调配到运输跟踪,物流应用涵盖了物流业务的各个环节。然而,随着业务规模的不断扩大和用户需求的日益复杂,物流应用面临着性能提升的挑战。优化物流应用的操作流程和开发过程中的性能,对于提高物流企业的竞争力和用户满意度至关重要。本文将深入探讨如何优化物流应用的操作流程以及分享实际开发中的优化技巧,并结合相关代码示例,为物流应用开发者提供实践指导。
一、优化物流应用的操作流程
(一)订单处理流程优化
- 自动化订单录入:传统的手动订单录入方式不仅效率低下,还容易出现错误。引入自动化订单录入系统,通过与客户系统、电商平台等对接,实现订单信息的自动抓取和录入。例如,在与电商平台对接时,利用电商平台提供的 API 接口获取订单数据。以 Python 和电商平台(假设为某知名电商平台)的 API 对接为例:
import requests
import json
# 电商平台API地址
url = 'https://api.ecommerceplatform.com/orders'
# 身份认证信息
headers = {
'Authorization': 'Bearer your_token',
'Content-Type': 'application/json'
}
# 获取订单数据
response = requests.get(url, headers=headers)
if response.status_code == 200:
orders = json.loads(response.text)
for order in orders:
# 处理订单数据,如录入物流应用数据库
process_order(order)
else:
print('获取订单失败,状态码:', response.status_code)
- 智能订单分配:根据订单的重量、体积、目的地、紧急程度等因素,利用算法自动分配最合适的运输资源和配送路线。在实际实现中,可以使用 Dijkstra 算法来计算最优配送路线。以下是一个简化的 Python 实现示例,假设图结构以邻接矩阵表示:
import heapq
def dijkstra(graph, start):
distances = {node: float('inf') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
dist, current = heapq.heappop(pq)
if dist > distances[current]:
continue
for neighbor, weight in enumerate(graph[current]):
if weight > 0:
new_dist = dist + weight
if new_dist < distances[neighbor]:
distances[neighbor] = new_dist
heapq.heappush(pq, (new_dist, neighbor))
return distances
(二)库存管理流程优化
- 实时库存监控与预警:通过物联网技术和传感器,实时监控库存水平。当库存低于设定的安全阈值时,自动触发预警机制,通知相关人员进行补货。在库存管理系统中,可以使用 SQL 查询实时获取库存数据,并结合编程语言实现预警功能。以 Java 和 MySQL 数据库为例:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class InventoryMonitor {
private static final String DB_URL = "jdbc:mysql://localhost:3306/logistics_db";
private static final String DB_USER = "your_username";
private static final String DB_PASSWORD = "your_password";
public static void main(String[] args) {
try (Connection connection = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT product_id, quantity FROM inventory")) {
while (resultSet.next()) {
int productId = resultSet.getInt("product_id");
int quantity = resultSet.getInt("quantity");
int safetyThreshold = getSafetyThreshold(productId); // 假设该方法获取产品的安全阈值
if (quantity < safetyThreshold) {
sendAlert(productId, quantity); // 发送预警通知
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
private static int getSafetyThreshold(int productId) {
// 从数据库或其他配置文件中获取安全阈值
return 10; // 示例值
}
private static void sendAlert(int productId, int quantity) {
// 实现发送预警通知的逻辑,如邮件、短信等
System.out.println("产品 " + productId + " 的库存仅剩 " + quantity + ",低于安全阈值,请补货!");
}
}
- 库存优化算法:运用库存优化算法,如经济订货批量(EOQ)模型,确定最优的订货量和订货时间,以降低库存成本。EOQ 模型的计算公式为:EOQ=2DSH−−−−√EOQ=2DSH,其中 D 为年需求量,S 为每次订货成本,H 为单位产品的年持有成本。在 Python 中实现 EOQ 模型:
import math
def calculate_eoq(demand, setup_cost, holding_cost):
return math.sqrt((2 * demand * setup_cost) / holding_cost)
(三)运输跟踪流程优化
- 多源数据整合:整合来自 GPS 设备、车辆传感器、运输合作伙伴系统等多源数据,实现对货物运输状态的实时跟踪。在后端开发中,使用消息队列(如 Kafka)来处理和整合这些数据。以下是一个简单的 Kafka 生产者示例,用于发送运输设备的 GPS 数据:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerConfig;
import org.apache.kafka.clients.producer.ProducerRecord;
import java.util.Properties;
public class GPSDataProducer {
public static void main(String[] args) {
Properties properties = new Properties();
properties.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
properties.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringSerializer");
properties.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(properties);
String topic = "gps_data_topic";
String gpsData = "latitude: 34.0522, longitude: -118.2437";
ProducerRecord<String, String> record = new ProducerRecord<>(topic, gpsData);
producer.send(record);
producer.close();
}
}
- 可视化运输跟踪界面:为用户提供直观的可视化运输跟踪界面,以地图、图表等形式展示货物的运输轨迹和预计到达时间。在前端开发中,使用 JavaScript 和地图 API(如百度地图 API)实现运输轨迹可视化:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>运输跟踪</title>
<script src="https://api.map.baidu.com/api?v=3.0&ak=your_ak"></script>
</head>
<body>
<div id="map" style="width: 800px; height: 600px;"></div>
<script>
var map = new BMap.Map("map");
map.centerAndZoom(new BMap.Point(116.404, 39.915), 11);
// 假设gpsPoints是包含经纬度的数组
var gpsPoints = [
{latitude: 34.0522, longitude: -118.2437},
{latitude: 34.1234, longitude: -118.1122}
];
var polyline = new BMap.Polyline(gpsPoints.map(point => new BMap.Point(point.longitude, point.latitude)), {strokeColor: "red", strokeWeight: 2, strokeOpacity: 0.5});
map.addOverlay(polyline);
</script>
</body>
</html>
二、实际开发中的优化技巧
(一)代码优化
- 算法优化:在物流应用中,涉及到许多复杂的算法,如路径规划、库存优化等。选择高效的算法可以显著提升应用性能。例如,在路径规划中,A算法相比 Dijkstra 算法在启发式搜索下能更快地找到最优路径。以下是一个简单的 A算法 Python 实现框架:
import heapq
def heuristic(a, b):
# 启发式函数,例如曼哈顿距离
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def astar(graph, start, goal):
open_set = []
heapq.heappush(open_set, (0, start))
came_from = {}
g_score = {node: float('inf') for node in graph}
g_score[start] = 0
f_score = {node: float('inf') for node in graph}
f_score[start] = heuristic(start, goal)
while open_set:
_, current = heapq.heappop(open_set)
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.reverse()
return path
for neighbor in graph[current]:
tentative_g_score = g_score[current] + graph[current][neighbor]
if tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = tentative_g_score + heuristic(neighbor, goal)
if neighbor not in [i[1] for i in open_set]:
heapq.heappush(open_set, (f_score[neighbor], neighbor))
return None
- 减少代码冗余:审查代码,去除重复的代码片段,将通用功能封装成独立的函数或模块。例如,在物流应用中,订单处理、库存管理等模块可能都需要进行数据验证,将数据验证功能封装成一个独立的函数,在不同模块中调用:
def validate_data(data):
# 数据验证逻辑,如检查数据格式、必填字段等
if not isinstance(data, dict):
return False
required_fields = ['field1', 'field2', 'field3']
for field in required_fields:
if field not in data:
return False
return True
(二)数据库优化
- 索引优化:合理创建索引可以加快数据库查询速度。在物流应用中,经常需要根据订单号、运单号、产品 ID 等字段进行查询。为这些频繁查询的字段创建索引,能够显著提升查询效率。例如,在 MySQL 数据库中,使用CREATE INDEX语句创建索引:
CREATE INDEX idx_order_number ON orders(order_number);
CREATE INDEX idx_waybill_number ON waybills(waybill_number);
CREATE INDEX idx_product_id ON inventory(product_id);
- 数据库分区:对于数据量较大的表,如订单历史表、运输记录表等,采用数据库分区技术。按照时间、地区等维度进行分区,减少单次查询的数据量,提高查询性能。在 MySQL 中,使用PARTITION BY RANGE语句进行按时间分区:
CREATE TABLE orders (
order_id INT,
order_date DATE,
customer_id INT,
amount DECIMAL(10, 2)
)
PARTITION BY RANGE (YEAR(order_date)) (
PARTITION p2020 VALUES LESS THAN (2021),
PARTITION p2021 VALUES LESS THAN (2022),
PARTITION p2022 VALUES LESS THAN (2023),
PARTITION p2023 VALUES LESS THAN (2024)
);
(三)系统架构优化
- 微服务架构:将物流应用拆分为多个独立的微服务,如订单服务、库存服务、运输服务等。每个微服务专注于特定的业务功能,独立部署和扩展。这样可以提高系统的灵活性和可维护性,当某个服务出现性能问题时,不会影响其他服务的正常运行。在 Spring Cloud 框架中,可以使用@SpringBootApplication和@RestController注解创建一个简单的订单微服务:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class OrderServiceApplication {
public static void main(String[] args) {
SpringApplication.run(OrderServiceApplication.class, args);
}
@GetMapping("/orders/{orderId}")
public String getOrder(@PathVariable int orderId) {
// 从数据库或其他数据源获取订单信息
return "订单ID: " + orderId + " 的详细信息";
}
}
- 缓存机制:在物流应用中,一些数据的变化频率较低,如地区编码表、运输价格表等。可以将这些数据缓存在内存中,减少对数据库的查询次数。常用的缓存工具如 Redis,在 Java 应用中,使用 Jedis 库操作 Redis 缓存:
import redis.clients.jedis.Jedis;
public class RedisCacheExample {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost", 6379);
// 从缓存中获取地区编码表
String regionCodes = jedis.get("region_codes");
if (regionCodes == null) {
// 如果缓存中没有,从数据库查询
regionCodes = getRegionCodesFromDatabase();
// 将查询到的数据存入缓存
jedis.set("region_codes", regionCodes);
}
jedis.close();
}
private static String getRegionCodesFromDatabase() {
// 模拟从数据库获取地区编码表数据
return "地区编码数据";
}
}
三、总结
优化物流应用的操作流程和开发性能是提升物流企业竞争力的关键举措。通过优化订单处理、库存管理和运输跟踪等操作流程,以及在实际开发中运用代码优化、数据库优化和系统架构优化等技巧,能够显著提高物流应用的效率和用户体验。在物流行业不断发展的背景下,持续关注和应用这些优化方法,将为物流企业提供更强大的数字化支持,推动物流行业的高效发展。希望本文能为物流应用开发者在性能优化方面提供有益的参考和实践指导。
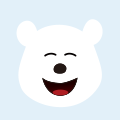