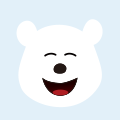
回复
在HarmonyOS应用开发中,数据持久化是一个非常重要的环节。关系型数据库(RDB)提供了一种结构化的数据存储方式,非常适合存储需要频繁查询和操作的复杂数据。本文将详细介绍如何在HarmonyOS应用中使用@ohos.data.relationalStore
模块进行数据库的增删改查操作。
首先确保你的DevEco Studio已配置好HarmonyOS开发环境,并在module.json5
文件中添加了必要的权限:
我们首先需要初始化数据库并创建表:
关键点说明:
getRdbStore()
方法用于获取或创建数据库SecurityLevel.S1
表示数据库安全级别executeSql()
用于执行SQL语句创建表我们使用ArkUI构建了一个简单的界面来展示操作按钮和数据表格:
本文详细介绍了HarmonyOS关系型数据库的CRUD操作,包括:
通过这个示例,你可以快速掌握HarmonyOS中关系型数据库的基本操作,为开发数据驱动的应用打下坚实基础。