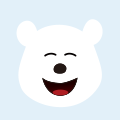
回复
反射操作注解
可以通过反射API: getAnnotations, getAnnotation获得相关的注解信息
Bean.class
package first.reflect;
@table("person")
public class Bean {
@Myfield(column = "id", type = "int", length = 10)
private int id;
@Myfield(column = "name", type = "string", length = 10)
private String name;
@Myfield(column = "age", type = "int", length = 10)
private int age;
public Bean() {
}
public Bean(int age, String name, int id) {
this.age = age;
this.name = name;
this.id = id;
}
@Myfun("getAge函数")
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
测试代码:
package first.reflect;
import java.lang.annotation.*;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
/**
* 反射获取注解
*/
@table("person")
public class test06 {
public static void main(String[] args) throws Exception {
Bean bean = new Bean();
Class c = bean.getClass();
//获取类的所有注解
Annotation annotation[] = c.getAnnotations();
for (Annotation a : annotation)
System.out.println(a);
//获得类的指定注解
table t = (table) c.getAnnotation(table.class);
System.out.println(t.value());
//获得类的属性的注解
Field f = c.getDeclaredField("name");
Myfield myfield = f.getAnnotation(Myfield.class);
System.out.println(myfield.column() + " " + myfield.type() + " " + myfield.length());
//获得类方法的注解
Method m = c.getDeclaredMethod("getAge");
Myfun myfun = m.getAnnotation(Myfun.class);
System.out.println(myfun.value());
}
}
@Target(value = ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@interface table {
String value();
}
@Target(value = ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
@interface Myfield {
String column();
String type();
int length();
}
@Target(value = ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
@interface Myfun {
String value();
}
反射操作泛型
Java采用泛型擦除的机制来引入泛型。Java中的泛型仅仅是给编译器javac使用的,确保数据的安全性和免去强制类型转换的麻烦。但是,一旦编译完成,所有的和泛型有关的类型全部擦除。
为了通过反射操作这些类型以迎合实际开发的需要,Java就新增了ParameterizedType,GenericArrayType,TypeVariable和WildcardType几种类型来代表不能被归一到Class类中的类型但是又和原始类型齐名的类型
package first.reflect;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.List;
import java.util.Map;
/**
* 通过反射读取泛型
*/
public class test05 {
public static void test1(Map<String, Bean> map, List<Bean> list) {
System.out.println("test1");
}
public static Map<Integer, Bean> test2() {
System.out.println("test2");
return null;
}
public static void main(String[] args) throws Exception {
//返回指定方法参数的泛型信息
Method method = test05.class.getDeclaredMethod("test1", Map.class, List.class);
Type[] types = method.getGenericParameterTypes();
for (Type t : types) {
System.out.println("#" + t);
if (t instanceof ParameterizedType) {
Type[] genericTypes = ((ParameterizedType) t).getActualTypeArguments();
for (Type generictype : genericTypes)
System.out.println("泛型类型" + generictype);
}
}
//返回指定方法返回值的泛型信息
Method method1 = test05.class.getDeclaredMethod("test2", null);
Type returnType = method1.getGenericReturnType();
if (returnType instanceof ParameterizedType) {
Type[] genericTypes1 = ((ParameterizedType) returnType).getActualTypeArguments();
for (Type generictype : genericTypes1)
System.out.println("返回值的泛型类型" + generictype);
}
}
}
作者:Baret H ~
来源:CSDN