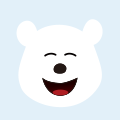
玩转SpringBoot—自动装配解决Bean的复杂配置
作者 | 宇木木兮
来源 |今日头条
学习目标
- 理解自动装配的核心原理
- 能手写一个EnableAutoConfiguration注解
- 理解SPI机制的原理
第1章 集成Redis
1.引入依赖包
2.配置参数
3.controller
通过上面的案例,我们就能看出来,RedisTemplate这个类的bean对象,我们并没有通过XML的方式也没有通过注解的方式注入到IoC容器中去,但是我们就是可以通过@Autowired注解自动从容器里面拿到相应的Bean对象,再去进行属性注入。
那这是怎么做到的呢?接下来我们来分析一下自动装配的原理,等我们弄明白了原理,自然而然你们就懂了RedisTemplate的bean对象怎么来的。
第2章 自动装配原理
1.SpringBootApplication注解是入口
在其中比较重要的有三个注解,分别是:
- @SpringBootConfiguration:继承了Configuration,表示当前是注解类
- @EnableAutoConfiguration: 开启springboot的注解功能,springboot的四大神器之一,其借助@import的帮助
- @ComponentScan(excludeFilters = { // 扫描路径设置(具体使用待确认)
2.1 ComponentScan
ComponentScan的功能其实就是自动扫描并加载符合条件的组件(比如@Component和@Repository等)或者bean定义;并将这些bean定义加载到IoC容器中.
我们可以通过basePackages等属性来细粒度的定制@ComponentScan自动扫描的范围,如果不指定,则默认Spring框架实现会从声明@ComponentScan所在类的package进行扫描。
注:所以SpringBoot的启动类最好是放在root package下,因为默认不指定basePackages
2.2 EnableAutoConfiguration
此注解顾名思义是可以自动配置,所以应该是springboot中最为重要的注解。
其中最重要的两个注解:
- @AutoConfigurationPackage
- @Import(AutoConfigurationImportSelector.class)
当然还有其中比较重要的一个类就是:
AutoConfigurationImportSelector.class
2.2.1 AutoConfigurationPackage
通过@Import(
注册当前启动类的根package;
注册
org.springframework.boot.autoconfigure.AutoConfigurationPackages的BeanDefinition。
AutoConfigurationPackage注解的作用是将添加该注解的类所在的package作为自动配置package 进行管理。
可以通过 AutoConfigurationPackages 工具类获取自动配置package列表。当通过注解@SpringBootApplication标注启动类时,已经为启动类添加了@AutoConfigurationPackage注解。路径为 @SpringBootApplication -> @EnableAutoConfiguration -> @AutoConfigurationPackage。也就是说当SpringBoot应用启动时默认会将启动类所在的package作为自动配置的package。
如我们创建了一个sbia-demo的应用,下面包含一个启动模块demo-bootstrap,启动类时Bootstrap,它添加了@SpringBootApplication注解,我们通过测试用例可以看到自动配置package为com.tm.sbia.demo.boot。 2.2.2 AutoConfigurationImportSelector
可以从图中看出
AutoConfigurationImportSelector实现了 DeferredImportSelector 从 ImportSelector继承的方法:selectImports。
第9行List configurations =
getCandidateConfigurations(annotationMetadata,`attributes);其实是去加载各个组件jar下的 public static final String FACTORIES_RESOURCE_LOCATION = "META-INF/spring.factories";外部文件。
如果获取到类信息,spring可以通过类加载器将类加载到jvm中,现在我们已经通过spring-boot的starter依赖方式依赖了我们需要的组件,那么这些组件的类信息在select方法中就可以被获取到。
其返回一个自动配置类的类名列表,方法调用了loadFactoryNames方法,查看该方法
自动配置器会跟根据传入的factoryClass.getName()到项目系统路径下所有的spring.factories文件中找到相应的key,从而加载里面的类。
这个外部文件,有很多自动配置的类。如下:其中,最关键的要属@Import(
AutoConfigurationImportSelector.class),借助AutoConfigurationImportSelector,@EnableAutoConfiguration可以帮助SpringBoot应用将所有符合条件(spring.factories)的bean定义(如Java Config@Configuration配置)都加载到当前SpringBoot创建并使用的IoC容器。
2.3 SpringFactoriesLoader
其实SpringFactoriesLoader的底层原理就是借鉴于JDK的SPI机制,所以,在将SpringFactoriesLoader之前,我们现在发散一下SPI机制。
2.3.1 SPI
SPI ,全称为 Service Provider Interface,是一种服务发现机制。它通过在ClassPath路径下的META-INF/services文件夹查找文件,自动加载文件里所定义的类。这一机制为很多框架扩展提供了可能,比如在Dubbo、JDBC中都使用到了SPI机制。我们先通过一个很简单的例子来看下它是怎么用的。
2.3.1.1 例子
首先,我们需要定义一个接口,SPIService
然后,定义两个实现类,没别的意思,只输入一句话。
最后呢,要在ClassPath路径下配置添加一个文件。文件名字是接口的全限定类名,内容是实现类的全限定类名,多个实现类用换行符分隔。
文件路径如下:内容就是实现类的全限定类名:
2.3.1.2 测试
然后我们就可以通过ServiceLoader.load或者Service.providers方法拿到实现类的实例。其中,Service.providers包位于sun.misc.Service,而ServiceLoader.load包位于java.util.ServiceLoader。
两种方式的输出结果是一致的:
2.3.1.3 源码分析
我们看到一个位于sun.misc包,一个位于java.util包,sun包下的源码看不到。我们就以ServiceLoader.load为例,通过源码看看它里面到底怎么做的。
1.ServiceLoader
首先,我们先来了解下ServiceLoader,看看它的类结构。
2.Load
load方法创建了一些属性,重要的是实例化了内部类,LazyIterator。最后返回ServiceLoader的实例。
3.查找实现类
查找实现类和创建实现类的过程,都在LazyIterator完成。当我们调用iterator.hasNext和iterator.next方法的时候,实际上调用的都是LazyIterator的相应方法。
所以,我们重点关注lookupIterator.hasNext()方法,它最终会调用到hasNextService。
4.创建实例
当然,调用next方法的时候,实际调用到的是,
lookupIterator.nextService。它通过反射的方式,创建实现类的实例并返回。
看到这儿,我想已经很清楚了。获取到类的实例,我们自然就可以对它为所欲为了!
2.3.1.4 JDBC中的应用
我们开头说,SPI机制为很多框架的扩展提供了可能,其实JDBC就应用到了这一机制。回忆一下JDBC获取数据库连接的过程。在早期版本中,需要先设置数据库驱动的连接,再通过
DriverManager.getConnection获取一个Connection。
在较新版本中(具体哪个版本,笔者没有验证),设置数据库驱动连接,这一步骤就不再需要,那么它是怎么分辨是哪种数据库的呢?答案就在SPI。
1.加载
我们把目光回到DriverManager类,它在静态代码块里面做了一件比较重要的事。很明显,它已经通过SPI机制, 把数据库驱动连接初始化了。
具体过程还得看loadInitialDrivers,它在里面查找的是Driver接口的服务类,所以它的文件路径就是:
META-INF/services/java.sql.Driver。
那么,这个文件哪里有呢?我们来看MySQL的jar包,就是这个文件,文件内容为:
com.mysql.cj.jdbc.Driver2.创建实例
上一步已经找到了MySQL中的com.mysql.jdbc.Driver全限定类名,当调用next方法时,就会创建这个类的实例。它就完成了一件事,向DriverManager注册自身的实例。
3.创建Connection
在
DriverManager.getConnection()方法就是创建连接的地方,它通过循环已注册的数据库驱动程序,调用其connect方法,获取连接并返回。
4.再扩展
既然我们知道JDBC是这样创建数据库连接的,我们能不能再扩展一下呢?如果我们自己也创建一个java.sql.Driver文件,自定义实现类MyDriver,那么,在获取连接的前后就可以动态修改一些信息。
还是先在项目ClassPath下创建文件,文件内容为自定义驱动类
com.viewscenes.netsupervisor.spi.MyDriver
我们的MyDriver实现类,继承自MySQL中的NonRegisteringDriver,还要实现java.sql.Driver接口。这样,在调用connect方法的时候,就会调用到此类,但实际创建的过程还靠MySQL完成。
2.3.2 回到SpringFactoriesLoader
借助于Spring框架原有的一个工具类:SpringFactoriesLoader的支持,@EnableAutoConfiguration可以智能的自动配置功效才得以大功告成!
SpringFactoriesLoader属于Spring框架私有的一种扩展方案,其主要功能就是从指定的配置文件META-INF/spring.factories加载配置,加载工厂类。
SpringFactoriesLoader为Spring工厂加载器,该对象提供了loadFactoryNames方法,入参为factoryClass和classLoader即需要传入工厂类名称和对应的类加载器,方法会根据指定的classLoader,加载该类加器搜索路径下的指定文件,即spring.factories文件;
传入的工厂类为接口,而文件中对应的类则是接口的实现类,或最终作为实现类。
配合@EnableAutoConfiguration使用的话,它更多是提供一种配置查找的功能支持,即根据@EnableAutoConfiguration的完整类名
org.springframework.boot.autoconfigure.EnableAutoConfiguration作为查找的Key,获取对应的一组@Configuration类上图就是从SpringBoot的autoconfigure依赖包中的META-INF/spring.factories配置文件中摘录的一段内容,可以很好地说明问题。
(重点)所以,@EnableAutoConfiguration自动配置的魔法其实就变成了:
从classpath中搜寻所有的META-INF/spring.factories配置文件,并将其中
org.springframework.boot.autoconfigure.EnableAutoConfiguration对应的配置项通过反射(Java Refletion)实例化为对应的标注了@Configuration的JavaConfig形式的IoC容器配置类,然后汇总为一个并加载到IoC容器。
第3章 补充内容
- @Target 注解可以用在哪。TYPE表示类型,如类、接口、枚举@Target(ElementType.TYPE) //接口、类、枚举@Target(ElementType.FIELD) //字段、枚举的常量
- @Target(ElementType.METHOD) //方法@Target(ElementType.PARAMETER) //方法参数@Target(ElementType.CONSTRUCTOR) //构造函数
- @Target(ElementType.LOCAL_VARIABLE)//局部变量@Target(ElementType.ANNOTATION_TYPE)//注解@Target(ElementType.PACKAGE) ///包
- @Retention 注解的保留位置。只有RUNTIME类型可以在运行时通过反射获取其值@Retention(RetentionPolicy.SOURCE) //注解仅存在于源码中,在class字节码文件中不包含@Retention(RetentionPolicy.CLASS) // 默认的保留策略,注解会在class字节码文件中存在,但运行时无法获得,@Retention(RetentionPolicy.RUNTIME) // 注解会在class字节码文件中存在,在运行时可以通过反射获取到
- @Documented 该注解在生成javadoc文档时是否保留
- @Inherited 被注解的元素,是否具有继承性,如子类可以继承父类的注解而不必显式的写下来。
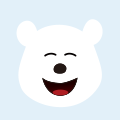