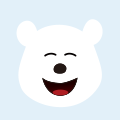
DES加密算法是怎么实现的?
前面阿粉说了关于 MD5 加密算法,还有 RSA 加密算法的实现,以及他们的前世今生,今天阿粉在来说一下这个关于 DES 加密算法,又是怎么实现的。
DES加密算法
DES 加密,是对称加密,之前阿粉也已经说了这个对称加密和非对称加密都是代表了什么意思,对称加密,顾名思义,加密和解密的运算全都是使用的同样的秘钥。
DES加密算法原始思想可以参照二战德国的恩格玛机,其基本思想大致相同。传统的密码加密都是由古代的循环移位思想而来,恩格玛机在这个基础之上进行了扩散模糊。但是本质原理都是一样的。现代DES在二进制级别做着同样的事:替代模糊,增加分析的难度。
DES加密原理
DES 使用一个 56 位的密钥以及附加的 8 位奇偶校验位,产生最大 64 位的分组大小。
这是一个迭代的分组密码,使用称为 Feistel 的技术,其中将加密的文本块分成两半。
使用子密钥对其中一半应用循环功能,然后将输出与另一半进行“异或”运算;接着交换这两半,这一过程会继续下去,但最后一个循环不交换。
DES 使用 16 个循环,使用异或,置换,代换,移位操作四种基本运算。
虽然现在 DES 加密已经被破解,但是如果保密级别不是很高的话,依然是可以使用的。
既然我们已经知道DES 加密的过程是从明文64位开始,然后到初始置换IP,之后生成子秘钥,然后在秘钥控制下进行16轮加密转换,再做一次交换左右32比特,最后进行逆初始置换IP,最后返回密文的64位。
就像下面的图:
具体的算法,阿粉暂时不说,直接开始我们的 Java 代码实现。
DES 加密算法Java实现
其实 DES 加密过程如果要是简化出来的话,无非就是那么几步。
第一步:明文根据IP置换,变成新的明文,得到一个乱序的64 bit 明文组。
将新得到的加密明文分成两个部分,Lo和Ro。
第二步:子秘钥生成,DES加密过程有16轮循环函数,其中需要用到16个密钥,所以要将这56 bit密钥扩展生成16个48 bit 的子密钥。
第三步:得到16个子密钥K
第四步:S盒代换数据
第五步:P盒代换,P为固定置换,将经过S盒变换得到的32 bit进行一个置换操作。至此,得到F函数的最终输出。
第六步:循环16次
第七步:IP的逆置换
最后输出64位的比特密文。
就这么简单,如果你要是理解了的话,那就没那么多问题了。
你学会了么?
本文转载自公众号:java极客技术
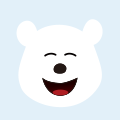