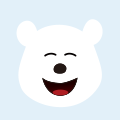
『江鸟中原』鸿蒙大作业——抽奖 原创
中原工学院鸿蒙期末大作业——转盘抽奖
一、摘要
1.通过画布组件Canvas,画出抽奖圆形转盘。
2.通过显式动画启动抽奖功能。
3.通过自定义弹窗弹出抽中的奖品。
二、环境搭建
1.软件要求
DevEco Studio版本:DevEco Studio 3.1 Release。
HarmonyOS SDK版本:API version 9。
2.硬件要求
设备类型:华为手机或运行在DevEco Studio上的华为手机设备模拟器。
HarmonyOS系统:3.1.0 Developer Release。
三、程序设计
1.构建主页面
效果图如下!
在绘制抽奖圆形转盘前,首先需要在CanvasPage.ets的aboutToAppear方法中获取屏幕的宽高。
let context = getContext(this);
aboutToAppear() {
// 获取屏幕的宽高
window.getLastWindow(context)
.then((windowClass: window.Window) => {
let windowProperties = windowClass.getWindowProperties();
this.screenWidth = px2vp(windowProperties.windowRect.width);
this.screenHeight = px2vp(windowProperties.windowRect.height);
})
.catch((error: Error) => {
Logger.error('Failed to obtain the window size. Cause: ' + JSON.stringify(error));
})
}
在CanvasPage.ets布局界面中添加Canvas组件,在onReady方法中进行绘制。
build() {
Stack({ alignContent: Alignment.Center }) {
Canvas(this.canvasContext)
.width(StyleConstants.FULL_PERCENT)
.height(StyleConstants.FULL_PERCENT)
.onReady(() => {
this.drawModel.draw(this.canvasContext, this.screenWidth, this.screenHeight);
})
.rotate({
x: 0,
y: 0,
z: 1,
angle: this.rotateDegree,
centerX: this.screenWidth / CommonConstants.TWO,
centerY: this.screenHeight / CommonConstants.TWO
})
Image($r('app.media.ic_center'))
.width(StyleConstants.CENTER_IMAGE_WIDTH)
.height(StyleConstants.CENTER_IMAGE_HEIGHT)
.enabled(this.enableFlag)
.onClick(() => {
this.enableFlag = !this.enableFlag;
this.startAnimator();
})
}
.width(StyleConstants.FULL_PERCENT)
.height(StyleConstants.FULL_PERCENT)
.backgroundImage($r('app.media.ic_background'), ImageRepeat.NoRepeat)
.backgroundImageSize({
width: StyleConstants.FULL_PERCENT,
height: StyleConstants.BACKGROUND_IMAGE_SIZE
})
}
在DrawModel.ets中,通过draw方法逐步进行自定义圆形抽奖转盘的绘制。
draw(canvasContext: CanvasRenderingContext2D, screenWidth: number, screenHeight: number) {
if (CheckEmptyUtils.isEmptyObj(canvasContext)) {
Logger.error('[DrawModel][draw] canvasContext is empty.');
return;
}
this.canvasContext= canvasContext;
this.screenWidth = screenWidth;
this.canvasContext.clearRect(0, 0, this.screenWidth, screenHeight);
// 将画布沿X、Y轴平移指定距离
this.canvasContext.translate(this.screenWidth / CommonConstants.TWO,
screenHeight / CommonConstants.TWO);
// 画外部圆盘的花瓣
this.drawFlower();
// 画外部圆盘、小圈圈
this.drawOutCircle();
// 画内部圆盘
this.drawInnerCircle();
// 画内部扇形抽奖区域
this.drawInnerArc();
// 画内部扇形区域文字
this.drawArcText();
// 画内部扇形区域奖品对应的图片
this.drawImage();
this.canvasContext.translate(-this.screenWidth / CommonConstants.TWO,
-screenHeight / CommonConstants.TWO);
}
2.外部圆盘
画外部圆盘的花瓣:通过调用rotate()方法,将画布旋转指定角度。再通过调用save()和restore()方法,使画布保存最新的绘制状态。根据想要绘制的花瓣个数,改变旋转角度,循环画出花瓣效果。
// 画外部圆盘的花瓣
drawFlower() {
let beginAngle = this.startAngle + this.avgAngle;
const pointY = this.screenWidth * CommonConstants.FLOWER_POINT_Y_RATIOS;
const radius = this.screenWidth * CommonConstants.FLOWER_RADIUS_RATIOS;
const innerRadius = this.screenWidth * CommonConstants.FLOWER_INNER_RATIOS;
for (let i = 0; i < CommonConstants.COUNT; i++) {
this.canvasContext?.save();
this.canvasContext?.rotate(beginAngle * Math.PI / CommonConstants.HALF_CIRCLE);
this.fillArc(new FillArcData(0, -pointY, radius, 0, Math.PI * CommonConstants.TWO),
ColorConstants.FLOWER_OUT_COLOR);
this.fillArc(new FillArcData(0, -pointY, innerRadius, 0, Math.PI * CommonConstants.TWO),
ColorConstants.FLOWER_INNER_COLOR);
beginAngle += this.avgAngle;
this.canvasContext?.restore();
}
}
// 画弧线方法
fillArc(fillArcData: FillArcData, fillColor: string) {
if (CheckEmptyUtils.isEmptyObj(fillArcData) || CheckEmptyUtils.isEmptyStr(fillColor)) {
Logger.error('[DrawModel][fillArc] fillArcData or fillColor is empty.');
return;
}
if (this.canvasContext !== undefined) {
this.canvasContext.beginPath();
this.canvasContext.fillStyle = fillColor;
this.canvasContext.arc(fillArcData.x, fillArcData.y, fillArcData.radius,
fillArcData.startAngle, fillArcData.endAngle);
this.canvasContext.fill();
}
drawOutCircle() {
// 画外部圆盘
this.fillArc(new FillArcData(0, 0, this.screenWidth * CommonConstants.OUT_CIRCLE_RATIOS, 0,
Math.PI * CommonConstants.TWO), ColorConstants.OUT_CIRCLE_COLOR);
let beginAngle = this.startAngle;
// 画小圆圈
for (let i = 0; i < CommonConstants.SMALL_CIRCLE_COUNT; i++) {
this.canvasContext?.save();
this.canvasContext?.rotate(beginAngle * Math.PI / CommonConstants.HALF_CIRCLE);
this.fillArc(new FillArcData(this.screenWidth * CommonConstants.SMALL_CIRCLE_RATIOS, 0,
CommonConstants.SMALL_CIRCLE_RADIUS, 0, Math.PI * CommonConstants.TWO),
ColorConstants.WHITE_COLOR);
beginAngle = beginAngle + CommonConstants.CIRCLE / CommonConstants.SMALL_CIRCLE_COUNT;
this.canvasContext?.restore();
}
}
3.画内部扇形抽奖区域
// 画内部圆盘
drawInnerCircle() {
this.fillArc(new FillArcData(0, 0, this.screenWidth * CommonConstants.INNER_CIRCLE_RATIOS, 0,
Math.PI * CommonConstants.TWO), ColorConstants.INNER_CIRCLE_COLOR);
this.fillArc(new FillArcData(0, 0, this.screenWidth * CommonConstants.INNER_WHITE_CIRCLE_RATIOS, 0,
Math.PI * CommonConstants.TWO), ColorConstants.WHITE_COLOR);
}
// 画内部扇形抽奖区域
drawInnerArc() {
// 颜色集合
let colors = [
ColorConstants.ARC_PINK_COLOR, ColorConstants.ARC_YELLOW_COLOR,
ColorConstants.ARC_GREEN_COLOR, ColorConstants.ARC_PINK_COLOR,
ColorConstants.ARC_YELLOW_COLOR, ColorConstants.ARC_GREEN_COLOR
];
let radius = this.screenWidth * CommonConstants.INNER_ARC_RATIOS;
for (let i = 0; i < CommonConstants.COUNT; i++) {
this.fillArc(new FillArcData(0, 0, radius, this.startAngle * Math.PI / CommonConstants.HALF_CIRCLE,
(this.startAngle + this.avgAngle) * Math.PI / CommonConstants.HALF_CIRCLE), colors[i]);
this.canvasContext?.lineTo(0, 0);
this.canvasContext?.fill();
this.startAngle += this.avgAngle;
}
}
// 画内部扇形区域文字
drawArcText() {
if (this.canvasContext !== undefined) {
this.canvasContext.textAlign = CommonConstants.TEXT_ALIGN;
this.canvasContext.textBaseline = CommonConstants.TEXT_BASE_LINE;
this.canvasContext.fillStyle = ColorConstants.TEXT_COLOR;
this.canvasContext.font = StyleConstants.ARC_TEXT_SIZE + CommonConstants.CANVAS_FONT;
}
// 需要绘制的文本数组集合
let textArrays = [
$r('app.string.text_smile'),
$r('app.string.text_hamburger'),
$r('app.string.text_cake'),
$r('app.string.text_smile'),
$r('app.string.text_beer'),
$r('app.string.text_watermelon')
];
let arcTextStartAngle = CommonConstants.ARC_START_ANGLE;
let arcTextEndAngle = CommonConstants.ARC_END_ANGLE;
for (let i = 0; i < CommonConstants.COUNT; i++) {
this.drawCircularText(this.getResourceString(textArrays[i]),
(this.startAngle + arcTextStartAngle) * Math.PI / CommonConstants.HALF_CIRCLE,
(this.startAngle + arcTextEndAngle) * Math.PI / CommonConstants.HALF_CIRCLE);
this.startAngle += this.avgAngle;
}
}
// 绘制圆弧文本
drawCircularText(textString: string, startAngle: number, endAngle: number) {
if (CheckEmptyUtils.isEmptyStr(textString)) {
Logger.error('[DrawModel][drawCircularText] textString is empty.');
return;
}
class CircleText {
x: number = 0;
y: number = 0;
radius: number = 0;
};
let circleText: CircleText = {
x: 0,
y: 0,
radius: this.screenWidth * CommonConstants.INNER_ARC_RATIOS
};
// 圆的半径
let radius = circleText.radius - circleText.radius / CommonConstants.COUNT;
// 每个字母占的弧度
let angleDecrement = (startAngle - endAngle) / (textString.length - 1);
let angle = startAngle;
let index = 0;
let character: string;
while (index < textString.length) {
character = textString.charAt(index);
this.canvasContext?.save();
this.canvasContext?.beginPath();
this.canvasContext?.translate(circleText.x + Math.cos(angle) * radius,
circleText.y - Math.sin(angle) * radius);
this.canvasContext?.rotate(Math.PI / CommonConstants.TWO - angle);
this.canvasContext?.fillText(character, 0, 0);
angle -= angleDecrement;
index++;
this.canvasContext?.restore();
}
// 画内部扇形区域文字对应的图片
drawImage() {
let beginAngle = this.startAngle;
let imageSrc = [
CommonConstants.WATERMELON_IMAGE_URL, CommonConstants.BEER_IMAGE_URL,
CommonConstants.SMILE_IMAGE_URL, CommonConstants.CAKE_IMAGE_URL,
CommonConstants.HAMBURG_IMAGE_URL, CommonConstants.SMILE_IMAGE_URL
];
for (let i = 0; i < CommonConstants.COUNT; i++) {
let image = new ImageBitmap(imageSrc[i]);
this.canvasContext?.save();
this.canvasContext?.rotate(beginAngle * Math.PI / CommonConstants.HALF_CIRCLE);
this.canvasContext?.drawImage(image, this.screenWidth * CommonConstants.IMAGE_DX_RATIOS,
this.screenWidth * CommonConstants.IMAGE_DY_RATIOS, CommonConstants.IMAGE_SIZE,
CommonConstants.IMAGE_SIZE);
beginAngle += this.avgAngle;
this.canvasContext?.restore();
}
}
4.实现抽奖功能
圆形转盘开始转动抽奖:给转盘指定一个随机的转动角度randomAngle,保证每次转动的角度是随机的,即每次抽到的奖品也是随机的。动画结束后,转盘停止转动,抽奖结束,弹出抽中的奖品信息。
dialogController: CustomDialogController = new CustomDialogController({
builder: PrizeDialog({
prizeData: $prizeData,
enableFlag: $enableFlag
}),
autoCancel: false
});
// 开始抽奖
startAnimator() {
let randomAngle = Math.round(Math.random() * CommonConstants.CIRCLE);
// 获取中奖信息
this.prizeData = this.drawModel.showPrizeData(randomAngle);
animateTo({
duration: CommonConstants.DURATION,
curve: Curve.Ease,
delay: 0,
iterations: 1,
playMode: PlayMode.Normal,
onFinish: () => {
this.rotateDegree = CommonConstants.ANGLE - randomAngle;
// 打开自定义弹窗,弹出抽奖信息
this.dialogController.open();
}
}, () => {
this.rotateDegree = CommonConstants.CIRCLE * CommonConstants.FIVE +
CommonConstants.ANGLE - randomAngle;
})
弹出抽中的奖品信息:抽奖结束后,弹出抽中的文本和图片信息,通过自定义弹窗实现。
@CustomDialog
export default struct PrizeDialog {
@Link prizeData: PrizeData;
@Link enableFlag: boolean;
private controller?: CustomDialogController;
build() {
Column() {
Image(this.prizeData.imageSrc !== undefined ? this.prizeData.imageSrc : '')
.width($r('app.float.dialog_image_size'))
.height($r('app.float.dialog_image_size'))
.margin({
top: $r('app.float.dialog_image_top'),
bottom: $r('app.float.dialog_image_bottom')
})
.transform(matrix4.identity().rotate({
x: 0,
y: 0,
z: 1,
angle: CommonConstants.TRANSFORM_ANGLE
}))
Text(this.prizeData.message)
.fontSize($r('app.float.dialog_font_size'))
.textAlign(TextAlign.Center)
.margin({ bottom: $r('app.float.dialog_message_bottom') })
Text($r('app.string.text_confirm'))
.fontColor($r('app.color.text_font_color'))
.fontWeight(StyleConstants.FONT_WEIGHT)
.fontSize($r('app.float.dialog_font_size'))
.textAlign(TextAlign.Center)
.onClick(() => {
this.controller?.close();
this.enableFlag = !this.enableFlag;
})
}
.backgroundColor($r('app.color.dialog_background'))
.width(StyleConstants.FULL_PERCENT)
.height($r('app.float.dialog_height'))
}
}
四、总结
虽然通过一个学期的学习对鸿蒙有了更多的认识,但是还是远远不够,尽管在借鉴多个demo下完成了期末大作业,想要将鸿蒙学习更进一步,还需要日后进一步的学习。最后,我相信在今后的工作和学习中,鸿蒙操作系统都将成为一个非常重要的工具和平台。我将继续深入学习和开发,探索更多的鸿蒙技术领域,努力成为一名专业的鸿蒙操作系统开发者,并在这个领域中取得更大的成就和贡献。
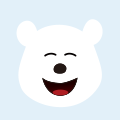