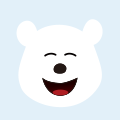
回复
在鸿蒙应用开发中,TextInput
组件用于接收用户输入,适用于文本、密码等多种输入类型。本文详细介绍鸿蒙 TextInput
组件的使用方法,包括输入限制、样式设置、事件监听及搜索框应用,帮助你灵活处理鸿蒙应用中的用户输入。
TextInput
是接收用户文本输入的基础组件,支持多种输入类型、占位符、自定义样式等设置。
创建文本输入框,设置占位符和背景颜色,并使用 @State
保存输入内容:
@Entry
@Component
struct TextInputDemo {
@State inputText: string = ''; // 定义状态变量保存输入内容
build() {
Column() {
TextInput({ placeholder: 'Enter text here' }) // 创建带占位符的输入框
.onChange((value) => this.inputText = value) // 监听输入变化,将内容保存到 inputText
.backgroundColor(Color.Pink) // 设置背景颜色为粉色
.fontColor(Color.Black) // 设置文本颜色为黑色
.fontSize(20) // 设置字体大小
}
.width('100%') // 设置列宽度为 100%
.height('100%') // 设置列高度为 100%
}
}
效果示例
TextInput
支持多种样式设置,方便定制字体、占位符、边框和对齐等视觉效果。
设置占位符的颜色和字体样式:
TextInput({ placeholder: 'Enter your username' }) // 设置占位符文本
.placeholderColor(Color.Gray) // 设置占位符颜色为灰色
.fontSize(18) // 设置字体大小为 18
.fontWeight(FontWeight.Normal) // 设置字体粗细为正常
通过 borderColor
和 borderRadius
设置边框颜色和圆角效果:
TextInput({ placeholder: 'Enter your password' }) // 设置占位符文本
.borderColor(Color.Blue) // 设置边框颜色为蓝色
.borderWidth(2) // 设置边框宽度为 2
.borderRadius(10) // 设置圆角半径为 10
使用 backgroundColor
设置输入框背景色:
TextInput({ placeholder: 'Type here...' }) // 设置占位符文本
.backgroundColor(Color.Gray) // 设置背景颜色为灰色
效果示例
使用 onChange
事件监听输入内容变化并保存到 @State
变量:
@Entry
@Component
struct InputListener {
@State userInput: string = ''; // 定义状态变量保存输入内容
build() {
Column() {
TextInput({ placeholder: 'Enter text here' }) // 创建带占位符的输入框
.onChange((value) => this.userInput = value) // 输入内容变化时,更新 userInput
.fontSize(18) // 设置字体大小为 18
Text(`You entered: ${this.userInput}`) // 显示输入的内容
.fontSize(16) // 设置显示文本的字体大小为 16
.margin({ top: 10 }) // 设置上边距为 10
}
}
}
使用 onFocus
和 onBlur
事件监听输入框的聚焦和失焦,适合执行特定操作:
TextInput({ placeholder: 'Type your message' }) // 创建带占位符的输入框
.onFocus(() => console.log('Input field focused')) // 输入框聚焦时,输出日志
.onBlur(() => console.log('Input field unfocused')) // 输入框失焦时,输出日志
在实际开发中,TextInput
常用于创建搜索框,可实时更新输入内容,并带有清空内容的功能。
实现一个带清除按钮的搜索框:
@Entry
@Component
struct SearchInput {
@State searchText: string = ''; // 定义状态变量保存搜索内容
build() {
Column() {
Row() {
TextInput({ placeholder: 'Search here...' }) // 设置搜索输入框占位符
.onChange((value) => this.searchText = value) // 输入变化时更新 searchText
.fontColor(Color.Black) // 设置字体颜色为黑色
.backgroundColor(Color.Gray) // 设置背景颜色为灰色
.fontSize(18) // 设置字体大小为 18
.width('80%') // 设置宽度为 80%
Button('Clear') // 创建清除按钮
.onClick(() => this.searchText = '') // 点击清除按钮时清空搜索内容
.width(60) // 设置按钮宽度为 60
.margin({ left: 10 }) // 设置左边距为 10
}
.margin({ bottom: 20 }) // 设置下边距为 20
if (this.searchText.length > 0) {
Text(`Searching for: ${this.searchText}`) // 显示当前输入的搜索内容
.fontSize(16) // 设置字体大小为 16
.fontColor(Color.Blue) // 设置字体颜色为蓝色
} else {
Text('Please enter a search term.') // 当没有输入时显示提示文本
.fontSize(16) // 设置字体大小为 16
.fontColor(Color.Gray) // 设置字体颜色为灰色
}
}
.padding(20) // 设置整体容器内边距为 20
}
}
searchText
用于存储用户输入内容。TextInput
输入框设置占位符,并通过 onChange
事件实时更新 searchText
。Button
清除按钮点击后会清空 searchText
,清空搜索框内容。使用 maxLength
限制输入字符数:
TextInput({ placeholder: 'Enter your username' }) // 设置占位符文本
.maxLength(10) // 限制最多输入 10 个字符
在输入框旁添加清除按钮,快速清空输入内容:
Row() {
TextInput({ placeholder: 'Type here...' }) // 设置占位符文本
.onChange((value) => this.text = value) // 绑定内容变化
Button('Clear') // 创建清除按钮
.onClick(() => this.text = '') // 点击清除按钮时清空内容
.width(50) // 设置按钮宽度为 50
.margin({ left: 10 }) // 设置左边距为 10
}
本文介绍了 TextInput
组件的基本用法、样式设置、事件监听,并展示了搜索框的实战应用,帮助开发者在鸿蒙应用中灵活处理用户输入。
下一篇将介绍 Switch 和 Checkbox 组件,实现切换和选择功能。