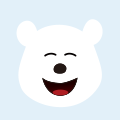
鸿蒙NEXT开发案例:扫雷
【引言】
本文将介绍如何使用鸿蒙NEXT框架开发一个简单的扫雷游戏。通过本案例,您将学习到如何利用鸿蒙NEXT的组件化特性、状态管理以及用户交互设计来构建一个完整的游戏应用。
【环境准备】
电脑系统:windows 10
开发工具:DevEco Studio NEXT Beta1 Build Version: 5.0.3.806
工程版本:API 12
真机:Mate 60 Pro
语言:ArkTS、ArkUI
【项目结构】
本项目主要由两个类组成:Cell类表示游戏中的每一个方块,MineSweeper类则是整个扫雷游戏的核心组件。
Cell类
Cell类用于表示游戏面板上的每一个方块,包含以下属性:
row和column:方块的行列位置。
hasMine:标记该方块是否有地雷。
neighborMines:记录周围地雷的数量。
isFlag:标记该方块是否被标记为地雷。
value:方块的显示值。
MineSweeper类
MineSweeper类是游戏的主要逻辑,包含以下关键方法:
初始化游戏:initializeGame方法重置游戏状态,生成新的游戏面板。
生成游戏面板:generateBoard方法创建一个10x10的方块数组,并随机放置地雷。
计算周围地雷数量:calculateNumbers方法为每个方块计算周围的地雷数量。
揭示方块:revealCell方法处理方块的揭示逻辑,包括递归揭示周围方块。
胜利与失败判断:isVictory和showGameOverDialog方法用于判断游戏的胜利或失败,并显示相应的对话框。
【用户界面】
游戏的用户界面通过build方法构建,使用了鸿蒙NEXT的布局组件。主要包括:
重新开始按钮:点击后重新初始化游戏。
游戏面板:使用ForEach遍历每个方块,显示其值,并处理用户的点击和长按事件。
【交互设计】
游戏支持单击揭示方块和长按标记地雷的操作,用户体验友好。通过状态管理,游戏能够实时更新方块的显示状态和游戏进程。
【完整代码】
import { promptAction } from '@kit.ArkUI'; // 导入用于显示对话框的工具
// 定义方块类
@ObservedV2 // 使用@ObservedV2装饰器使得类的状态可以被自动追踪
class Cell {
row: number; // 方块所在的行
column: number; // 方块所在的列
hasMine: boolean = false; // 是否有地雷,默认没有
neighborMines: number = 0; // 周围地雷数量,默认为0
@Trace isFlag: boolean = false; // 是否被标记为地雷,默认没有标记
@Trace value: string; // 方块值,显示数字或“雷”
// 构造函数,初始化方块的位置和值
constructor(row: number, column: number) {
this.row = row;
this.column = column;
this.value = ''; // 初始为空字符串
}
}
// 定义扫雷游戏组件
@Entry // 使用@Entry装饰器声明这是一个入口组件
@Component // 使用@Component装饰器声明这是一个组件
struct MineSweeper {
@State private gameBoard: Cell[][] = []; // 游戏面板数据
@State private mineCount: number = 10; // 地雷总数
@State private revealedCells: Set<string> = new Set(); // 已经揭示的方块集合
@State private flaggedCells: Set<string> = new Set(); // 标记为地雷的方块集合
@State private cellSize: number = 60; // 方块大小
@State private cellMargin: number = 2; // 方块之间的边距
private startTime: number = Date.now(); // 游戏开始时间
@State private isGameOver: boolean = false; // 游戏结束标志
// 当组件即将显示时调用此方法
aboutToAppear(): void {
this.initializeGame(); // 初始化游戏
}
// 初始化游戏
private initializeGame() {
this.isGameOver = false; // 设置游戏未结束
this.startTime = Date.now(); // 重置游戏开始时间
this.revealedCells.clear(); // 清空已揭示的方块集合
this.flaggedCells.clear(); // 清空标记为地雷的方块集合
this.generateBoard(); // 生成新的游戏面板
}
// 生成游戏面板
private generateBoard() {
this.gameBoard = []; // 清空游戏面板
for (let i = 0; i < 10; i++) { // 循环创建10行
this.gameBoard.push([]); // 每行初始化为空数组
for (let j = 0; j < 10; j++) { // 循环创建10列
this.gameBoard[i].push(new Cell(i, j)); // 为每个位置创建一个新方块
}
}
this.placeMines(); // 随机放置地雷
this.calculateNumbers(); // 计算每个方块周围的地雷数量
}
// 随机放置地雷
private placeMines() {
let placed = 0; // 已放置的地雷数量
while (placed < this.mineCount) { // 当已放置的地雷少于设定数量时继续放置
let x = Math.floor(Math.random() * 10); // 随机选择一行
let y = Math.floor(Math.random() * 10); // 随机选择一列
if (!this.gameBoard[x][y].hasMine) { // 如果该位置还没有地雷
this.gameBoard[x][y].hasMine = true; // 在该位置放置地雷
placed++; // 地雷计数加1
}
}
}
// 计算每个方块周围的地雷数量
private calculateNumbers() {
for (let i = 0; i < 10; i++) { // 遍历每一行
for (let j = 0; j < 10; j++) { // 遍历每一列
if (!this.gameBoard[i][j].hasMine) { // 如果当前方块不是地雷
this.gameBoard[i][j].neighborMines = this.countNeighborMines(i, j); // 计算并设置周围地雷数量
this.gameBoard[i][j].value = this.gameBoard[i][j].neighborMines.toString(); // 将数量转换为字符串
} else {
this.gameBoard[i][j].value = '雷'; // 如果是地雷,则设置值为"雷"
}
}
}
}
// 计算给定坐标周围地雷的数量
private countNeighborMines(row: number, col: number): number {
let count = 0; // 周围地雷计数
for (let dx = -1; dx <= 1; dx++) { // 遍历上下左右及对角线方向
for (let dy = -1; dy <= 1; dy++) {
if (dx === 0 && dy === 0) { // 跳过当前位置
continue;
}
let newRow = row + dx, newCol = col + dy; // 新的行列坐标
if (newRow >= 0 && newRow < 10 && newCol >= 0 && newCol < 10 && this.gameBoard[newRow][newCol].hasMine) { // 如果新位置有效且是地雷
count++; // 地雷计数加1
}
}
}
return count; // 返回地雷计数
}
// 揭示方块
private revealCell(row: number, col: number) {
if (this.isGameOver || this.revealedCells.has(`${row},${col}`)) { // 如果游戏结束或者该方块已经被揭示
return; // 不执行任何操作
}
const key = `${row},${col}`; // 创建方块唯一标识
this.revealedCells.add(key); // 添加到已揭示的方块集合
if (this.gameBoard[row][col].hasMine) { // 如果揭示的方块是地雷
this.showGameOverDialog(); // 显示游戏结束对话框
} else {
if (this.gameBoard[row][col].neighborMines === 0) { // 如果揭示的方块周围没有地雷
for (let dx = -1; dx <= 1; dx++) { // 自动揭示周围所有方块
for (let dy = -1; dy <= 1; dy++) {
if (dx === 0 && dy === 0) { // 跳过当前位置
continue;
}
let newRow = row + dx, newCol = col + dy;
if (newRow >= 0 && newRow < 10 && newCol >= 0 && newCol < 10) { // 如果新位置有效
this.revealCell(newRow, newCol); // 递归揭示相邻方块
}
}
}
}
}
if (this.isVictory()) { // 如果游戏胜利
this.showVictoryDialog(); // 显示胜利对话框
}
}
// 显示游戏结束对话框
private showGameOverDialog() {
this.isGameOver = true; // 设置游戏结束
promptAction.showDialog({ // 显示对话框
title: '游戏结束: 游戏失败!', // 对话框标题
buttons: [{ text: '重新开始', color: '#ffa500' }] // 对话框按钮
}).then(() => { // 点击按钮后执行
this.initializeGame(); // 重新开始游戏
});
}
// 显示胜利对话框
private showVictoryDialog() {
this.isGameOver = true; // 设置游戏结束
promptAction.showDialog({ // 显示对话框
title: '恭喜你,游戏胜利!', // 对话框标题
message: `用时:${((Date.now() - this.startTime) / 1000).toFixed(3)}秒`, // 对话框消息
buttons: [{ text: '重新开始', color: '#ffa500' }] // 对话框按钮
}).then(() => { // 点击按钮后执行
this.initializeGame(); // 重新开始游戏
});
}
// 判断游戏是否胜利
private isVictory(): boolean {
let revealedNonMineCount = 0; // 非地雷方块的揭示数量
for (let i = 0; i < this.gameBoard.length; i++) { // 遍历每一行
for (let j = 0; j < this.gameBoard[i].length; j++) { // 遍历每一列
if (this.revealedCells.has(`${i},${j}`)) { // 如果方块已被揭示
revealedNonMineCount++; // 非地雷方块的揭示数量加1
}
}
}
return revealedNonMineCount == 90; // 如果非地雷方块全部揭示,则游戏胜利
}
// 决定是否显示方块值
private isShowValue(cell: Cell): string {
if (this.isGameOver) { // 如果游戏结束
return cell.value === '0' ? '' : cell.value; // 显示方块值,除非是0
} else {
if (this.revealedCells.has(`${cell.row},${cell.column}`)) { // 如果方块已被揭示
return cell.value === '0' ? '' : cell.value; // 显示方块值,除非是0
} else {
return ''; // 否则不显示值
}
}
}
// 构建用户界面
build() {
Column({ space: 10 }) { // 创建垂直布局,元素之间间距10
Button('重新开始').onClick(() => this.initializeGame()); // 创建“重新开始”按钮,点击时重新开始游戏
Flex({ wrap: FlexWrap.Wrap }) { // 创建可换行的水平布局
ForEach(this.gameBoard, (row: Cell[], rowIndex: number) => { // 遍历每一行
ForEach(row, (cell: Cell, colIndex: number) => { // 遍历每一列
Stack() { // 创建层叠布局
Text(this.isShowValue(cell)) // 显示方块上的数字或“雷”
.width(`${this.cellSize}lpx`) // 设置宽度
.height(`${this.cellSize}lpx`) // 设置高度
.margin(`${this.cellMargin}lpx`) // 设置边距
.fontSize(`${this.cellSize / 2}lpx`) // 设置字体大小
.textAlign(TextAlign.Center) // 文本居中
.backgroundColor(this.revealedCells.has(`${rowIndex},${colIndex}`) ? // 设置背景颜色
(this.isShowValue(cell) === '雷' ? Color.Red : Color.White) : Color.Gray)
.fontColor(!this.revealedCells.has(`${rowIndex},${colIndex}`) || this.isShowValue(cell) === '雷' ? // 设置字体颜色
Color.White : Color.Black)
.borderRadius(5) // 设置圆角
.parallelGesture(GestureGroup(GestureMode.Exclusive, // 设置手势
TapGesture({ count: 1, fingers: 1 }) // 单击揭示方块
.onAction(() => this.revealCell(rowIndex, colIndex)),
LongPressGesture({ repeat: true }) // 长按标记地雷
.onAction(() => cell.isFlag = true)
));
Text(`${!this.revealedCells.has(`${rowIndex},${colIndex}`) ? '旗' : ''}`) // 显示标记旗帜
.width(`${this.cellSize}lpx`) // 设置宽度
.height(`${this.cellSize}lpx`) // 设置高度
.margin(`${this.cellMargin}lpx`) // 设置边距
.fontSize(`${this.cellSize / 2}lpx`) // 设置字体大小
.textAlign(TextAlign.Center) // 文本居中
.fontColor(Color.White) // 设置字体颜色
.visibility(cell.isFlag && !this.isGameOver ? Visibility.Visible : Visibility.None) // 设置可见性
.onClick(() => { // 点击取消标记
cell.isFlag = false;
})
}
});
});
}
.width(`${(this.cellSize + this.cellMargin * 2) * 10}lpx`); // 设置游戏面板宽度
}
.backgroundColor(Color.Orange) // 设置背景颜色
.width('100%') // 设置宽度为100%
.height('100%'); // 设置高度为100%
}
}
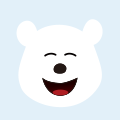