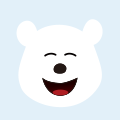
回复
【引言】
在本案例中,我们将展示如何使用鸿蒙NEXT框架开发一个简单的拼图游戏。该游戏允许用户选择一张图片,并将其分割成多个拼图块,用户可以通过点击交换拼图块的位置,最终完成拼图。
【环境准备】
电脑系统:windows 10
开发工具:DevEco Studio NEXT Beta1 Build Version: 5.0.3.806
工程版本:API 12
真机:Mate 60 Pro
语言:ArkTS、ArkUI
【项目结构】
本项目主要由以下几个部分组成:
图片选择:用户可以从设备中选择一张图片。
拼图生成:将选中的图片分割成多个拼图块。
拼图交换:用户可以通过点击拼图块进行交换。
拼图完成检测:检查用户是否完成拼图,并弹出提示。
【关键代码解析】
【结论】
通过本案例,我们展示了如何使用鸿蒙NEXT框架开发一个简单的拼图游戏。该项目涵盖了图片选择、拼图生成、拼图交换等基本功能,适合初学者学习和实践鸿蒙NEXT开发。希望本案例能够帮助您更好地理解鸿蒙NEXT的开发流程。
【完整代码】