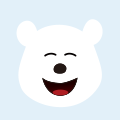
Arkts之拖拽操作-------Bond! 原创
概述
拖拽框架提供了一种通过鼠标或手势触屏的方式传递数据,即从一个组件位置拖出数据,并拖入到另一个组件位置上进行响应,拖出一方提供数据,拖入一方接收和处理数据。该操作可以让用户方便地移动、复制或删除指定内容。
拖拽操作:在某个能够响应拖出的组件上长按并滑动触发的拖拽行为,当用户释放时,拖拽操作结束;
拖拽背景(背板):用户所拖动数据的形象化表示,开发者可通过onDragStart的CustomerBuilder或DragItemInfo设置,也可以通过dragPreview通用属性设置;
拖拽内容:拖动的数据,使用UDMF统一API UnifiedData 进行封装;
拖出对象:触发拖拽操作并提供数据的组件;
拖入目标:可接收并处理拖动数据的组件;
拖拽点:鼠标或手指等与屏幕的接触位置,是否进入组件范围的判定是以接触点是否进入范围进行判断。
用到功能详解
onDragStart 支持拖出的组件产生拖出动作时触发。
该回调可以感知拖拽行为的发起,开发者可通过在 onDragStart 方法中设置拖拽所传递的数据以及自定义拖拽背板图。推荐开发者使用pixelmap的方式返回背板图,不推荐使用customBuilder的方式,会有额外的性能开销。
onDrop 当用户在组件范围内释放时触发,需在此回调中通过DragEvent中的setResult方法设置拖拽结果,否则在拖出方组件的onDragEnd方法中通过getResult方法只能拿到默认的处理结果DragResult.DRAG_FAILED。
该回调也是开发者干预系统默认拖入处理行为的地方,系统会优先执行开发者的onDrop回调,通过在回调中执行setResult方法来告知系统该如何处理所拖拽的数据;
- 设置 DragResult.DRAG_SUCCESSFUL,数据完全由开发者自己处理,系统不进行处理;
- 设置DragResult.DRAG_FAILED,数据不再由系统继续处理;
- 设置DragResult.DRAG_CANCELED,系统也不需要进行数据处理;
- 设置DragResult.DROP_ENABLED或DragResult.DROP_DISABLED会被忽略,同设置DragResult.DRAG_FAILED。
更多详细内容参阅官方文档: 拖拽事件
效果展示
具体代码实现
import { unifiedDataChannel, uniformTypeDescriptor } from '@kit.ArkData';
import { promptAction } from '@kit.ArkUI';
import { BusinessError } from '@kit.BasicServicesKit';
import { image } from '@kit.ImageKit';
interface Data {
name:string;
image:Resource;
color:Color;
}
@Entry
@Component
struct Index {
@State selectDayBtn: number = -1;
@State Data:Array<Data> = [
{name:"1",image:$r('app.media.beijing'),color:Color.Red},
{name:"2",image:$r('app.media.background'),color:Color.Blue},
{name:"3",image:$r('app.media.app_icon'),color:Color.Green},
{name:"4",image:$r('app.media.startIcon'),color:Color.Yellow}
]
build() {
Column() {
ForEach(this.Data, (item: Data, index: number) => {
Row() {
Image(this.Data[index].image)
.width(100)
.height(100)
Text(this.Data[index].name)
.fontSize(24)
.width(100)
.height(100)
.backgroundColor(this.Data[index].color)
}
.width('100%')
.onDragStart((event: DragEvent) => {
this.selectDayBtn = index; // 记录开始拖拽的索引
console.log(`开始拖拽: ${index}`);
})
.parallelGesture(LongPressGesture().onAction(() => {
// promptAction.showToast({ duration: 100, message: '拖动' });
}))
.onDrop((event: DragEvent) => {
if (this.selectDayBtn !== -1 && this.selectDayBtn !== index) {
// 交换数据
const temp = this.Data[this.selectDayBtn];
this.Data[this.selectDayBtn] = this.Data[index];
this.Data[index] = temp;
console.log(`交换位置: ${this.selectDayBtn} 和 ${index}`);
} else {
console.log('拖拽索引无效或未改变');
}
this.selectDayBtn = -1; // 重置拖拽索引
})
})
}
}
}
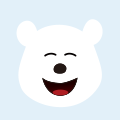