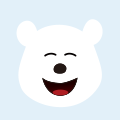
第十四课:HarmonyOS Next第三方库集成指南 原创
HarmonyOS Next第三方库集成指南:从原理到企业级实践
一、第三方库生态全景解析
1. 官方包管理工具(ohpm)
# 常用命令示例
ohpm install @ohos/lodash --save # 安装生产依赖
ohpm update @ohos/axios # 指定库更新
ohpm list --depth=1 # 查看一级依赖树
2. 库类型支持矩阵
库类型 | 适配要求 | 典型代表 |
纯JS库 | 无Native依赖 | lodash、moment |
混合库 | 提供ArkUI/NAPI组件 | react-native-harmony |
原生库 | 提供.so/.hap资源 | openCV-harmony |
AI模型库 | 符合HMOS Model格式 | tensorflow-lite-harmony |
二、六大核心集成方案
1. 网络请求库(axios-harmony)
// oh-package.json5配置
"dependencies": {
"@ohos/axios": "^2.0.5"
}
// 发起GET请求
import axios from '@ohos/axios';
axios.get('/user', {
params: { ID: 12345 },
timeout: 3000
}).then((response) => {
console.log(response.data);
});
2. UI动效库(Lottie-Harmony)
// 加载JSON动画资源
import { LottieAnimation } from '@ohos/lottie-harmony';
@Entry
@Component
struct AnimationPage {
build() {
LottieAnimation({
src: $rawfile('loading.json'),
loop: true,
autoPlay: true
}).width(200).height(200)
}
}
3. 状态管理库(Redux-Harmony)
// 创建Store实例
import { createStore } from '@ohos/redux-harmony';
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT': return state + 1;
default: return state;
}
}
const store = createStore(counterReducer);
// 组件中调用
@Observed
class CounterComp {
@ObjectLink count: number;
build() {
Text(`Count: ${this.count}`)
.onClick(() => store.dispatch({ type: 'INCREMENT' }))
}
}
三、企业级集成规范
1. 安全校验策略
// oh-package.json5安全配置
"security": {
"allowUnverified": false, // 禁止未验签库
"whitelist": ["@harmony/.*"],// 白名单正则
"checksumVerify": true // 强制哈希校验
}
2. 依赖优化方案
# 分析依赖树
ohpm analyze --size
# 输出结果示例
Package Size License
@ohos/lodash 1.2MB MIT
@ohos/axios 890KB Apache-2.0
3. 多环境配置管理
// config.ts动态配置
const configs = {
dev: {
apiBase: 'http://dev.example.com',
libs: { debug: true }
},
prod: {
apiBase: 'https://api.example.com',
libs: { debug: false }
}
}
export default configs[process.env.NODE_ENV];
四、高阶集成技巧
1. C/C++库混合编译
# CMakeLists.txt配置
cmake_minimum_required(VERSION 3.12)
project(native-lib)
add_library(
native-lib
SHARED
src/main/cpp/native-lib.cpp )
target_include_directories(
native-lib PRIVATE
${CMAKE_CURRENT_SOURCE_DIR}/include)
# ArkTS侧调用
import native from 'libnative.so';
native.callNativeMethod();
2. AI模型库集成
// 加载TFLite模型
import { TFLite } from '@ohos/tensorflow-lite';
const interpreter = await TFLite.create({
model: $rawfile('mobilenet_v2.tflite'),
options: {
threads: 4,
useGPU: true
}
});
const output = interpreter.run(inputTensor);
3. 分布式能力集成
// 跨设备调用库方法
import { DeviceManager } from '@ohos.distributedHardware';
const devices = DeviceManager.getTrustedDeviceList();
devices.forEach(device => {
device.callRemoteMethod('sharedLibMethod', { param: 'value' });
});
五、常见问题解决方案
Q1:依赖冲突如何解决?
# 查看依赖树定位冲突
ohpm list --dependency-conflict
# 强制指定版本(以lodash为例)
ohpm install @ohos/lodash@4.17.21 --force
Q2:Native库内存泄漏检测
// 启用内存监控
profiler.startMemoryMonitor({
samplingInterval: 1000,
callback: (report) => {
if (report.nativeHeap > 100MB) {
alert('Native内存异常');
}
}
});
Q3:旧版库兼容性处理
// 使用polyfill适配
if (!Array.prototype.flatMap) {
Array.prototype.flatMap = function(callback) {
return this.map(callback).flat();
};
}
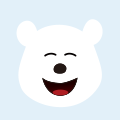