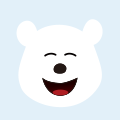
(七九)HarmonyOS Design 的自动化测试策略 原创
HarmonyOS Design 的自动化测试策略
在 HarmonyOS 应用开发的复杂生态中,自动化测试已成为保障应用质量、提升开发效率的关键环节。随着 HarmonyOS 应用功能日益丰富,用户对应用稳定性和性能要求不断提高,一套完善的自动化测试策略显得尤为重要。接下来,我们将深入探讨 HarmonyOS Design 中自动化测试的重要性,并结合代码示例详细阐述如何构建高效的测试框架。
自动化测试的重要性
提升测试效率
传统的手动测试在面对 HarmonyOS 应用复杂的功能和多样的设备适配时,耗时费力。例如,一款 HarmonyOS 的电商应用,包含商品浏览、购物车管理、支付等多个功能模块,手动测试每个功能在不同设备(如手机、平板、智能穿戴设备)上的表现,需要大量的时间和人力。而自动化测试可以在短时间内执行大量测试用例,快速覆盖各种场景。通过编写自动化测试脚本,如使用 Java 编写针对商品浏览功能的测试脚本:
import ohos.agp.components.Button;
import ohos.agp.components.Text;
import ohos.agp.components.element.ShapeElement;
import ohos.app.Context;
import ohos.test.UIEqualityComparator;
import ohos.test.UITestCase;
import ohos.test.UITestRunner;
import org.junit.runner.RunWith;
@RunWith(UITestRunner.class)
public class ProductBrowseTest extends UITestCase {
private Context context;
@Override
protected void setUp() throws Exception {
super.setUp();
context = getContext();
}
public void testProductBrowse() {
// 启动应用
startAbility("com.example.ecommerce/.MainAbility");
// 模拟点击商品列表中的某个商品
Button productItem = (Button) findComponentById("product_item_button");
productItem.click();
// 检查商品详情页面是否正确显示
Text productTitle = (Text) findComponentById("product_title_text");
assertNotNull(productTitle);
}
}
这样的脚本可以在不同设备上快速运行,大大提高了测试效率。
提高测试准确性
人工测试容易受到测试人员主观因素影响,出现遗漏或错误。自动化测试则严格按照预设的测试用例执行,确保每个测试点都被覆盖,结果更加准确可靠。例如,在测试 HarmonyOS 应用的界面布局在不同分辨率设备上的显示效果时,自动化测试可以通过获取界面元素的坐标、大小等属性,与预期值进行精确比对。使用UIEqualityComparator进行界面元素的比较:
// 假设已有预期的界面元素属性
ShapeElement expectedButtonBg = new ShapeElement();
expectedButtonBg.setRgbColor(0xff007BFF);
expectedButtonBg.setCornerRadius(8);
// 获取实际的按钮背景元素
Button actualButton = (Button) findComponentById("main_button");
ShapeElement actualButtonBg = (ShapeElement) actualButton.getBackgroundElement();
UIEqualityComparator comparator = new UIEqualityComparator();
boolean isEqual = comparator.equals(expectedButtonBg, actualButtonBg);
assertTrue(isEqual);
通过这种方式,避免了人工主观判断带来的误差,保证测试结果的准确性。
便于持续集成与持续交付(CI/CD)
在 HarmonyOS 应用的持续开发过程中,自动化测试是实现 CI/CD 的基础。每次代码提交后,自动化测试框架自动运行测试用例,及时发现代码变更带来的问题,确保新功能的添加或代码修改不会破坏原有功能。例如,在使用 Jenkins 搭建的 CI/CD 环境中,配置自动化测试任务:
- 安装 HarmonyOS 开发工具和测试框架相关依赖。
- 配置项目代码仓库地址,以便获取最新代码。
- 设定自动化测试脚本的执行路径,如上述的ProductBrowseTest脚本路径。
- 当代码有新的提交时,Jenkins 自动拉取代码,执行自动化测试。若测试通过,则继续后续的构建、部署流程;若测试失败,则及时通知开发者修复问题。
如何构建测试框架
选择合适的测试框架
HarmonyOS 提供了多种测试框架,如基于 Java 的ohos.test框架。该框架提供了丰富的 API 用于 UI 测试、功能测试等。在项目的build.gradle文件中添加测试依赖:
dependencies {
testImplementation 'com.huawei.ohos:ohos-test:1.0.0'
}
同时,根据项目需求,也可以结合第三方测试框架,如 Espresso for HarmonyOS,增强测试功能。例如,使用 Espresso for HarmonyOS 进行更复杂的 UI 交互测试:
import ohos.agp.components.Button;
import ohos.agp.components.Text;
import ohos.app.Context;
import ohos.test.UITestCase;
import ohos.test.UITestRunner;
import org.junit.runner.RunWith;
import static org.hamcrest.Matchers.allOf;
import static org.hamcrest.Matchers.instanceOf;
import static org.hamcrest.Matchers.is;
import static org.harmonyos.test.espresso.Espresso.onView;
import static org.harmonyos.test.espresso.action.ViewActions.click;
import static org.harmonyos.test.espresso.assertion.ViewAssertions.matches;
import static org.harmonyos.test.espresso.matcher.ViewMatchers.withId;
import static org.harmonyos.test.espresso.matcher.ViewMatchers.withText;
@RunWith(UITestRunner.class)
public class EspressoUITest extends UITestCase {
private Context context;
@Override
protected void setUp() throws Exception {
super.setUp();
context = getContext();
}
public void testLoginFunction() {
// 启动应用
startAbility("com.example.loginapp/.MainAbility");
// 输入用户名和密码
onView(withId("username_input")).perform(typeText("test_user"));
onView(withId("password_input")).perform(typeText("test_password"));
// 点击登录按钮
onView(withId("login_button")).perform(click());
// 验证登录成功后的页面显示
onView(withId("welcome_text")).check(matches(allOf(instanceOf(Text.class), withText("欢迎,test_user"))));
}
}
编写测试用例
根据 HarmonyOS 应用的功能模块和需求,编写全面的测试用例。以一个 HarmonyOS 的音乐播放应用为例,测试用例应包括:
- 功能测试:测试播放、暂停、下一曲、上一曲等基本功能。
public void testMusicPlayback() {
startAbility("com.example.musicapp/.MainAbility");
// 点击播放按钮
Button playButton = (Button) findComponentById("play_button");
playButton.click();
// 检查音乐是否正在播放,假设通过获取音乐服务状态判断
boolean isPlaying = getMusicServiceStatus();
assertTrue(isPlaying);
}
- 界面测试:测试界面布局、元素显示、颜色搭配等在不同设备上的效果。
public void testUILayout() {
startAbility("com.example.musicapp/.MainAbility");
// 检查播放列表按钮是否显示
Button playlistButton = (Button) findComponentById("playlist_button");
assertNotNull(playlistButton);
// 检查播放进度条的初始状态
ProgressBar progressBar = (ProgressBar) findComponentById("progress_bar");
assertEquals(0, progressBar.getProgress());
}
- 兼容性测试:测试应用在不同版本的 HarmonyOS 系统以及不同品牌、型号的设备上的运行情况。可以使用设备模拟器或真实设备进行测试,在测试脚本中添加设备相关的断言:
public void testDeviceCompatibility() {
String deviceModel = getDeviceModel();
if ("huawei_p40".equals(deviceModel)) {
// 针对华为P40设备的特定测试
startAbility("com.example.musicapp/.MainAbility");
// 检查某些功能在该设备上是否正常工作
assertTrue(specificFunctionOnHuaweiP40());
} else if ("huawei_matepad".equals(deviceModel)) {
// 针对华为MatePad设备的特定测试
startAbility("com.example.musicapp/.MainAbility");
// 检查界面布局在该设备上是否正确
assertTrue(correctLayoutOnMatePad());
}
}
管理测试数据
在自动化测试中,合理管理测试数据至关重要。测试数据应涵盖各种正常和异常情况。例如,在测试 HarmonyOS 应用的注册功能时,准备不同类型的测试数据:
// 正常注册数据
String validUsername = "test_user";
String validPassword = "Test@1234";
// 异常注册数据,如用户名已存在、密码不符合规则等
String existingUsername = "existing_user";
String invalidPassword = "weak";
在测试脚本中使用这些数据进行测试:
public void testRegistration() {
startAbility("com.example.app/.RegistrationAbility");
// 测试正常注册
onView(withId("username_input")).perform(typeText(validUsername));
onView(withId("password_input")).perform(typeText(validPassword));
onView(withId("register_button")).perform(click());
// 检查注册成功提示
onView(withId("success_toast")).check(matches(withText("注册成功")));
// 测试用户名已存在情况
onView(withId("username_input")).perform(typeText(existingUsername));
onView(withId("password_input")).perform(typeText(validPassword));
onView(withId("register_button")).perform(click());
// 检查错误提示
onView(withId("error_toast")).check(matches(withText("用户名已存在")));
}
通过以上对 HarmonyOS Design 中自动化测试重要性的分析以及测试框架构建方法的阐述,结合具体代码示例,开发者能够在应用开发过程中建立起完善的自动化测试体系。在实际应用中,不断优化测试框架,根据项目需求和应用特点持续改进测试策略,将为 HarmonyOS 应用的高质量交付提供有力保障。
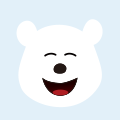