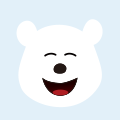
回复
鸿蒙开发板Hi3861驱动LCD1602A_4线并口模式_基于code-2.0
https://blog.csdn.net/txwtech/article/details/120690899
#include "_ansi.h"
#include <stdio.h>
#include "ohos_init.h"
#include "cmsis_os2.h"
#include "iot_gpio.h"
#include "hi_io.h"
#include "iot_pwm.h"
#include "hi_pwm.h"
#include "font_lcd1602.h"
/*
//
//by txwtech
2021.10
LCD1602--Hi3861 Board
2 VDD--3V3
3 V0 --GND
4 RS --GPIO6
5 RW --GND
6 E --GPIO8
11 D4 --GPIO9
12 D5 --GPIO10
13 D6 --GPIO11
14 D7 --GPIO12
15 A --3V3
16 K --GND
*/
#define lcd_rs HI_IO_NAME_GPIO_6 //,txianwu,
#define lcd_enable HI_IO_NAME_GPIO_8 //lcd
#define lcd_rs HI_IO_NAME_GPIO_10 //lcd
#define lcd_cs1 HI_IO_NAME_GPIO_12 ,
#define lcd_d4 HI_IO_NAME_GPIO_12 ,
#define lcd_d5 HI_IO_NAME_GPIO_12 ,
#define lcd_d6 HI_IO_NAME_GPIO_12 ,
#define lcd_d7 HI_IO_NAME_GPIO_12 ,
#define t_rs_pin HI_IO_NAME_GPIO_6 //,txianwu, RS
#define t_enable_pin HI_IO_NAME_GPIO_8 //lcd ,E
#define t_data_pins0 HI_IO_NAME_GPIO_9 , D4
#define t_data_pins1 HI_IO_NAME_GPIO_10 ,D5
#define t_data_pins2 HI_IO_NAME_GPIO_11 ,D6
#define t_data_pins3 HI_IO_NAME_GPIO_12 ,D7
//3v3--VDD
//GND--V0
//GND--RW
//3v3--A
//GND--K (lcd Pin16)
/*
*https://blog.csdn.net/weixin_45488643/article/details/108222308
*https://www.cnblogs.com/txwtech/p/11151064.html
*https://blog.csdn.net/txwtech/article/details/94970892
*http://www.360doc.com/content/18/0627/20/56844912_765915632.shtml
*/
void delay_ms(unsigned int ms)
{
osDelay((ms*5)/10);
}
size_t write1602(uint8_t value)
{
send1602(value, 1);
return 1; // assume sucess
}
// write either command or data, with automatic 4/8-bit selection
void send1602(uint8_t value, uint8_t mode)
{
//digitalWrite(_rs_pin, mode);
if(mode==0)
{
IoTGpioSetOutputVal(t_rs_pin, IOT_GPIO_VALUE0);
}
if(mode==1)
{
IoTGpioSetOutputVal(t_rs_pin, IOT_GPIO_VALUE1);
}
// if there is a RW pin indicated, set it low to Write
if (_rw_pin != 255) { //未使用RW--xxx
// digitalWrite(_rw_pin, LOW);
IoTGpioSetOutputVal(_rw_pin, IOT_GPIO_VALUE0);//xxx
}
if (_displayfunction & LCD_8BITMODE) {
write8bits(value);
}
else {
write4bits(value >> 4);
write4bits(value);
}
}
void command(uint8_t value)
{
// send(value, LOW);
send1602(value, 0);
}
void pulseEnable(void)
{
IoTGpioSetOutputVal(t_enable_pin, IOT_GPIO_VALUE1);
//delayMicroseconds(1); // enable pulse must be >450ns
delay_ms(15);
IoTGpioSetOutputVal(t_enable_pin, IOT_GPIO_VALUE0);
//digitalWrite(_enable_pin, LOW);
//delayMicroseconds(100); // commands need > 37us to settle
delay_ms(15);
}
void write4bits(uint8_t value)
{
for (int i = 0; i < 4; i++)
{
//digitalWrite(_data_pins[i], (value >> i) & 0x01);
switch (i)
{
case 0:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins0, (value >> i) & 0x01);
break;
case 1:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins1, (value >> i) & 0x01);
break;
case 2:/* constant-expression */
IoTGpioSetOutputVal(t_data_pins2, (value >> i) & 0x01);
/* code */
break;
case 3:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins3, (value >> i) & 0x01);
break;
default:
break;
}
int aa=(value>>i) & 0x01;
printf("line123, i=%d,write 4bits value:%d\n",i,aa);
delay_ms(5);
}
pulseEnable();
}
void write8bits(uint8_t value)
{
for (int i = 0; i < 8; i++)
{
switch (i)
{
case 0:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins0, (value >> i) & 0x01);
break;
case 1:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins1, (value >> i) & 0x01);
break;
case 2:/* constant-expression */
IoTGpioSetOutputVal(t_data_pins2, (value >> i) & 0x01);
/* code */
break;
case 3:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins3, (value >> i) & 0x01);
break;
default:
break;
}
int aa=(value>>i) & 0x01;
printf("line161, write 8bits value:%d\n",aa);
delay_ms(200);
}
pulseEnable();
}
void setRowOffsets(int row0, int row1, int row2, int row3)
{
_row_offsets[0] = row0;
_row_offsets[1] = row1;
_row_offsets[2] = row2;
_row_offsets[3] = row3;
}
void init(uint8_t fourbitmode, uint8_t rs, uint8_t rw, uint8_t enable,
uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7)
{
_rs_pin = rs; //
_rw_pin = rw;
_enable_pin = enable;
_data_pins[0] = d0;
_data_pins[1] = d1;
_data_pins[2] = d2;
_data_pins[3] = d3;
_data_pins[4] = d4;
_data_pins[5] = d5;
_data_pins[6] = d6;
_data_pins[7] = d7;
if (fourbitmode)
_displayfunction = LCD_4BITMODE | LCD_1LINE | LCD_5x8DOTS;
else
_displayfunction = LCD_8BITMODE | LCD_1LINE | LCD_5x8DOTS;
begin(16, 1,LCD_5x8DOTS);
}
void begin(uint8_t cols, uint8_t lines, uint8_t dotsize)
{
if (lines > 1) {
_displayfunction |= LCD_2LINE;
}
_numlines = lines;
setRowOffsets(0x00, 0x40, 0x00 + cols, 0x40 + cols);
// for some 1 line displays you can select a 10 pixel high font
if ((dotsize != LCD_5x8DOTS) && (lines == 1)) {
_displayfunction |= LCD_5x10DOTS;
}
//pinMode(_rs_pin, OUTPUT);
//IoTGpioSetOutputVal(t_rs_pin,);
// we can save 1 pin by not using RW. Indicate by passing 255 instead of pin#
if (_rw_pin != 255) {
//pinMode(_rw_pin, OUTPUT);
}
// Do these once, instead of every time a character is drawn for speed reasons.
for (int i = 0; i<((_displayfunction & LCD_8BITMODE) ? 8 : 4); ++i)
{
//pinMode(_data_pins[i], OUTPUT);
}
// SEE PAGE 45/46 FOR INITIALIZATION SPECIFICATION!
// according to datasheet, we need at least 40ms after power rises above 2.7V
// before sending commands. Arduino can turn on way before 4.5V so we'll wait 50
//delayMicroseconds(50000);
delay_ms(1);
// Now we pull both RS and R/W low to begin commands
//digitalWrite(_rs_pin, LOW);
IoTGpioSetOutputVal(t_rs_pin,IOT_GPIO_VALUE0);
//digitalWrite(_enable_pin, LOW);
IoTGpioSetOutputVal(t_enable_pin,IOT_GPIO_VALUE0);
if (_rw_pin != 255) {//rw未使用
//digitalWrite(_rw_pin, LOW);///rw未使用
}
//put the LCD into 4 bit or 8 bit mode
if (!(_displayfunction & LCD_8BITMODE)) {
// this is according to the hitachi HD44780 datasheet
// figure 24, pg 46
// we start in 8bit mode, try to set 4 bit mode
write4bits(0x03);
//delayMicroseconds(4500); // wait min 4.1ms
delay_ms(15);
// second try
write4bits(0x03);
//delayMicroseconds(4500); // wait min 4.1ms
delay_ms(15);
// third go!
write4bits(0x03);
//delayMicroseconds(150);
delay_ms(15);
// finally, set to 4-bit interface
write4bits(0x02);
printf("line272,LCD_8BITMODE\n");
}
else {
// this is according to the hitachi HD44780 datasheet
// page 45 figure 23
// Send function set command sequence
command(LCD_FUNCTIONSET | _displayfunction);
// delayMicroseconds(4500); // wait more than 4.1ms
delay_ms(15);
// second try
command(LCD_FUNCTIONSET | _displayfunction);
// delayMicroseconds(150);
delay_ms(15);
// third go
command(LCD_FUNCTIONSET | _displayfunction);
}
// finally, set # lines, font size, etc.
command(LCD_FUNCTIONSET | _displayfunction);
// turn the display on with no cursor or blinking default
_displaycontrol = LCD_DISPLAYON | LCD_CURSOROFF | LCD_BLINKOFF;
display();
// clear it off
clear();
// Initialize to default text direction (for romance languages)
_displaymode = LCD_ENTRYLEFT | LCD_ENTRYSHIFTDECREMENT;
// set the entry mode
command(LCD_ENTRYMODESET | _displaymode);
printf("line306,none LCD_8BITMODE\n");
}
// Turn the display on/off (quickly)
void noDisplay()
{
_displaycontrol &= ~LCD_DISPLAYON;
command(LCD_DISPLAYCONTROL | _displaycontrol);
}
void display()
{
_displaycontrol |= LCD_DISPLAYON;
command(LCD_DISPLAYCONTROL | _displaycontrol);
}
/********** high level commands, for the user! */
void clear()
{
command(LCD_CLEARDISPLAY); // clear display, set cursor position to zero
//delayMicroseconds(2000); // this command takes a long time!
delay_ms(10);
}
void send_to_lcd (char value, int rs)
{
//HAL_GPIO_WritePin(RS_GPIO_Port, RS_Pin, rs); // rs = 1 for data, rs=0 for command
if(1==rs)
IoTGpioSetOutputVal(t_rs_pin,IOT_GPIO_VALUE1);
if(0==rs)
IoTGpioSetOutputVal(t_rs_pin,IOT_GPIO_VALUE0);
/* write the data to the respective pin */
/* HAL_GPIO_WritePin(D7_GPIO_Port, D7_Pin, ((data>>3)&0x01));
HAL_GPIO_WritePin(D6_GPIO_Port, D6_Pin, ((data>>2)&0x01));
HAL_GPIO_WritePin(D5_GPIO_Port, D5_Pin, ((data>>1)&0x01));
HAL_GPIO_WritePin(D4_GPIO_Port, D4_Pin, ((data>>0)&0x01)); */
for (int i = 3; i >= 0; i--)
{
switch (i)
{
case 0:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins0, (value >> i) & 0x01);
break;
case 1:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins1, (value >> i) & 0x01);
break;
case 2:/* constant-expression */
IoTGpioSetOutputVal(t_data_pins2, (value >> i) & 0x01);
/* code */
break;
case 3:/* constant-expression */
/* code */
IoTGpioSetOutputVal(t_data_pins3, (value >> i) & 0x01);
break;
}
}
/* Toggle EN PIN to send the data
* if the HCLK > 100 MHz, use the 20 us delay
* if the LCD still doesn't work, increase the delay to 50, 80 or 100..
*/
//HAL_GPIO_WritePin(EN_GPIO_Port, EN_Pin, 1);
//delay (20);
//HAL_GPIO_WritePin(EN_GPIO_Port, EN_Pin, 0);
//delay (20);
IoTGpioSetOutputVal(t_enable_pin,IOT_GPIO_VALUE1);//延迟时间减小导致乱码
delay_ms(2);
IoTGpioSetOutputVal(t_enable_pin,IOT_GPIO_VALUE0);
delay_ms(1);
}
void lcd_send_cmd (char cmd)
{
char datatosend;
/* send upper nibble first */
datatosend = ((cmd>>4)&0x0f);
send_to_lcd(datatosend,0); // RS must be 0 while sending command
/* send Lower Nibble */
datatosend = ((cmd)&0x0f);
send_to_lcd(datatosend, 0);
}
void lcd_send_data (char data)
{
char datatosend;
/* send higher nibble */
datatosend = ((data>>4)&0x0f);
send_to_lcd(datatosend, 1); // rs =1 for sending data
/* send Lower nibble */
datatosend = ((data)&0x0f);
send_to_lcd(datatosend, 1);
}
void lcd_clear (void)
{
lcd_send_cmd(0x01);
delay_ms(2);
}
void lcd_put_cur(int row, int col)
{
switch (row)
{
case 0:
col |= 0x80;
break;
case 1:
col |= 0xC0;
break;
}
lcd_send_cmd (col);
}
void lcd_init (void)
{
// 4 bit initialisation
delay_ms(50); // wait for >40ms
lcd_send_cmd (0x30);
delay_ms(5); // wait for >4.1ms
lcd_send_cmd (0x30);
delay_ms(1); // wait for >100us
lcd_send_cmd (0x30);
delay_ms(10);
lcd_send_cmd (0x20); // 4bit mode
delay_ms(10);
// dislay initialisation
lcd_send_cmd (0x28); // Function set --> DL=0 (4 bit mode), N = 1 (2 line display) F = 0 (5x8 characters)
delay_ms(1);
lcd_send_cmd (0x08); //Display on/off control --> D=0,C=0, B=0 ---> display off
delay_ms(1);
lcd_send_cmd (0x01); // clear display
delay_ms(1);
delay_ms(1);
lcd_send_cmd (0x06); //Entry mode set --> I/D = 1 (increment cursor) & S = 0 (no shift)
delay_ms(1);
lcd_send_cmd (0x0C); //Display on/off control --> D = 1, C and B = 0. (Cursor and blink, last two bits)
}
void lcd_send_string (char *str)
{
while (*str) lcd_send_data (*str++);
}
static void lcd1602task(void *arg)
{
(void) arg;
IoTGpioInit(t_rs_pin);
IoTGpioInit(t_enable_pin);
IoTGpioInit(t_data_pins0); //9
IoTGpioInit(t_data_pins1); //10
IoTGpioInit(t_data_pins2); //11
IoTGpioInit(t_data_pins3);
hi_io_set_func(t_rs_pin,HI_IO_FUNC_GPIO_6_GPIO);
IoTGpioSetDir(t_rs_pin,IOT_GPIO_DIR_OUT);
IoTGpioSetDir(t_enable_pin,IOT_GPIO_DIR_OUT);
IoTGpioSetDir(t_data_pins0,IOT_GPIO_DIR_OUT); //9
IoTGpioSetDir(t_data_pins1,IOT_GPIO_DIR_OUT); //10
IoTGpioSetDir(t_data_pins2,IOT_GPIO_DIR_OUT); //11
IoTGpioSetDir(t_data_pins3,IOT_GPIO_DIR_OUT);
init(1,lcd_rs,255,lcd_enable,lcd_d4,lcd_d5,lcd_d6,lcd_d7,0,0,0,0);
begin(16,2,LCD_5x8DOTS);
printf("init is finished\n");
//write1602("lcd1602 display");
//display();
delay_ms(5);
lcd_clear();
while(1)
{
// lcd_init ();
lcd_put_cur(0, 0);
lcd_send_string("Hello ");
lcd_send_string("TXWTECH ");
// lcd_send_string("From");
lcd_put_cur(1, 0);
lcd_send_string("V:tianwu 2021.10");
delay_ms(300);
printf("lcd_send_data_test\n");
lcd_clear();
}
while (0)
{
/* IoTGpioSetOutputVal(_enable_pin,1);
IoTGpioSetOutputVal(_rs_pin,1);
IoTGpioSetOutputVal(_data_pins[0],1); */
printf("lcd1602\n");
//IoTGpioSetOutputVal(lcd_d4,IOT_GPIO_VALUE1); //9
// IoTGpioSetOutputVal(lcd_d5,IOT_GPIO_VALUE1); //10
// IoTGpioSetOutputVal(t_data_pins3,IOT_GPIO_VALUE1); //11
display();
delay_ms(1000);
noDisplay();
delay_ms(200);
write1602("v:txianwu");
display();
delay_ms(1000);
noDisplay();
delay_ms(200);
}
}
static void GpioEntry(void)
{
osThreadAttr_t attr={0};
attr.name="lcd1602task";
attr.stack_size=4096;
attr.priority=osPriorityNormal;
if(osThreadNew(lcd1602task,NULL,&attr)==NULL)
{
printf("[GpioEntry] create lcd1602task failed!\n");
}
}
SYS_RUN(lcd1602task);
头文件:
#ifndef font_lcd1602_h
#define font_lcd1602_h
// commands
#define LCD_CLEARDISPLAY 0x01
#define LCD_RETURNHOME 0x02
#define LCD_ENTRYMODESET 0x04
#define LCD_DISPLAYCONTROL 0x08
#define LCD_CURSORSHIFT 0x10
#define LCD_FUNCTIONSET 0x20
#define LCD_SETCGRAMADDR 0x40
#define LCD_SETDDRAMADDR 0x80
// flags for display entry mode
#define LCD_ENTRYRIGHT 0x00
#define LCD_ENTRYLEFT 0x02
#define LCD_ENTRYSHIFTINCREMENT 0x01
#define LCD_ENTRYSHIFTDECREMENT 0x00
// flags for display on/off control
#define LCD_DISPLAYON 0x04
#define LCD_DISPLAYOFF 0x00
#define LCD_CURSORON 0x02
#define LCD_CURSOROFF 0x00
#define LCD_BLINKON 0x01
#define LCD_BLINKOFF 0x00
// flags for display/cursor shift
#define LCD_DISPLAYMOVE 0x08
#define LCD_CURSORMOVE 0x00
#define LCD_MOVERIGHT 0x04
#define LCD_MOVELEFT 0x00
// flags for function set
#define LCD_8BITMODE 0x10
#define LCD_4BITMODE 0x00
#define LCD_2LINE 0x08
#define LCD_1LINE 0x00
#define LCD_5x10DOTS 0x04
#define LCD_5x8DOTS 0x00
typedef unsigned char uint8_t;
//LiquidCrystal(uint8_t rs, uint8_t enable,uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3); //arduino使用这个
void init(uint8_t fourbitmode, uint8_t rs, uint8_t rw, uint8_t enable,
uint8_t d0, uint8_t d1, uint8_t d2, uint8_t d3,
uint8_t d4, uint8_t d5, uint8_t d6, uint8_t d7);
//void begin(uint8_t cols, uint8_t rows, uint8_t charsize = LCD_5x8DOTS);
void begin(uint8_t cols, uint8_t rows, uint8_t charsize);
void clear();
void home();
void noDisplay();
void display();
void noBlink();
void blink();
void noCursor();
void cursor();
void scrollDisplayLeft();
void scrollDisplayRight();
void leftToRight();
void rightToLeft();
void autoscroll();
void noAutoscroll();
void setRowOffsets(int row1, int row2, int row3, int row4);
void createChar(uint8_t, uint8_t[]);
void setCursor(uint8_t, uint8_t);
size_t write1602(uint8_t);
void command(uint8_t);
//using Print::write;
void send1602(uint8_t, uint8_t);
void write4bits(uint8_t);
void write8bits(uint8_t);
void pulseEnable();
void delay_ms(unsigned int ms);
/* #define lcd_rs HI_IO_NAME_GPIO_6 //,txianwu,
#define lcd_enable HI_IO_NAME_GPIO_8 //lcd
#define lcd_rs HI_IO_NAME_GPIO_10 //lcd
#define lcd_cs1 HI_IO_NAME_GPIO_12 ,
#define lcd_d4 HI_IO_NAME_GPIO_12 ,
#define lcd_d5 HI_IO_NAME_GPIO_12 ,
#define lcd_d6 HI_IO_NAME_GPIO_12 ,
#define lcd_d7 HI_IO_NAME_GPIO_12 , */
uint8_t _rs_pin; // LOW: command. HIGH: character.GPIO_6
uint8_t _rw_pin; // LOW: write to LCD. HIGH: read from LCD.--GND
uint8_t _enable_pin; // activated by a HIGH pulse.GPIO_8
uint8_t _data_pins[8]; //d4,d5,d6,d7,by txianwu
uint8_t _displayfunction;
uint8_t _displaycontrol;
uint8_t _displaymode;
uint8_t _initialized;
uint8_t _numlines;
uint8_t _row_offsets[4];
void lcd_init (void); // initialize lcd
void lcd_send_cmd (char cmd); // send command to the lcd
void lcd_send_data (char data); // send data to the lcd
void lcd_send_string (char *str); // send string to the lcd
void lcd_put_cur(int row, int col); // put cursor at the entered position row (0 or 1), col (0-15);
void lcd_clear (void);
int row=0;
int col=0;
#endif