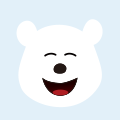
回复
【本文正在参与优质创作者激励】
老规矩还是将最终希望跑出来的效果放出来。如下:
结合上图的线程和代码可以看出来是内核线程跑着监控gpio输出充当中断的功能
@TOC
传统的Unix系统把一些重要的任务委托给周期性执行的进程,这些任务包括刷新磁盘高速缓存,交换出不用的页框,维护网络连接等等。事实上,以严格线性的方式执行这些任务的效率不高,如果把它们放在后台调度,不管是对它们的函数还是对终端用户进程都能得到较好的响应。因为一些系统进程只运行在内核态,所以现代操作系统把它们的函数委托给内核线程,内核线程不受不必要的用户态上下文的拖累。在Linux中,内核线程在以下几方面不同于普通进程:
#include <linux/module.h>
#include <linux/poll.h>
#include <linux/fs.h>
#include <linux/errno.h>
#include <linux/miscdevice.h>
#include <linux/kernel.h>
#include <linux/major.h>
#include <linux/mutex.h>
#include <linux/proc_fs.h>
#include <linux/seq_file.h>
#include <linux/stat.h>
#include <linux/init.h>
#include <linux/device.h>
#include <linux/tty.h>
#include <linux/kmod.h>
#include <linux/gfp.h>
#include <linux/gpio/consumer.h>
#include <linux/platform_device.h>
#include <linux/of_gpio.h>
#include <linux/of_irq.h>
#include <linux/interrupt.h>
#include <linux/irq.h>
#include <linux/slab.h>
#include <linux/fcntl.h>
#include <linux/timer.h>
#include <linux/workqueue.h>
#include <asm/current.h>
#include <linux/kthread.h>
struct task_struct *task;
static int major;
static struct class *sr501_class;
static struct gpio_desc *sr501_gpio;
static int sr501_data = 0;
static wait_queue_head_t sr501_wq;
struct task_struct *sr501_kthread;
/* 实现对应的read函数,填入file_operations结构体 */
static ssize_t sr501_drv_read (struct file *file, char __user *buf, size_t size, loff_t *offset)
{
int len = (size < 4)? size : 4;
wait_event_interruptible(sr501_wq, sr501_data);
printk("copy_to_user %s %s line %d\n", __FILE__, __FUNCTION__, __LINE__);
copy_to_user(buf, &sr501_data, len);
sr501_data = 0;
return len;
}
/* 定义file_operations结构体 */
static struct file_operations sr501_fops = {
.owner = THIS_MODULE,
.read = sr501_drv_read,
};
static int sr501_thread_func(void *data)
{
int val;
int pre = -1;
while(1)
{
val = gpiod_get_value(sr501_gpio);
if (pre != val)
{
printk("%s %s line %d val = %d\n", __FILE__, __FUNCTION__, __LINE__, val);
sr501_data = 0x80 | val;
wake_up(&sr501_wq);
pre = val;
}
set_current_state(TASK_INTERRUPTIBLE);
schedule_timeout(HZ);
if(kthread_should_stop()) {
set_current_state(TASK_RUNNING);
break;
}
}
return 0;
}
static int sr501_probe(struct platform_device *pdev)
{
/* 获得硬件信息 */
sr501_gpio = gpiod_get(&pdev->dev, NULL, 0);
gpiod_direction_input(sr501_gpio);
sr501_kthread = kthread_run(sr501_thread_func, NULL, "sr501d");
/* 注册file_operations */
major = register_chrdev(0, "sr501", &sr501_fops);
sr501_class = class_create(THIS_MODULE, "sr501_class");
if (IS_ERR(sr501_class)) {
printk("%s %s line %d\n", __FILE__, __FUNCTION__, __LINE__);
unregister_chrdev(major, "sr501");
return PTR_ERR(sr501_class);
}
/* device_create */
device_create(sr501_class, NULL, MKDEV(major, 0), NULL, "sr501");
return 0;
}
static int sr501_remove(struct platform_device *pdev)
{
device_destroy(sr501_class, MKDEV(major, 0));
class_destroy(sr501_class);
unregister_chrdev(major, "sr501");
return 0;
}
static const struct of_device_id qzk_sr501[] = {
{ .compatible = "qzk,sr501" },
{ },
};
/* 定义platform_driver */
static struct platform_driver sr501s_driver = {
.probe = sr501_probe,
.remove = sr501_remove,
.driver = {
.name = "qzk_sr501",
.of_match_table = qzk_sr501,
},
};
/* 在入口函数注册platform_driver */
static int __init sr501_init(void)
{
int err;
printk("%s %s line %d\n", __FILE__, __FUNCTION__, __LINE__);
init_waitqueue_head(&sr501_wq);
err = platform_driver_register(&sr501s_driver);
return err;
}
static void __exit sr501_exit(void)
{
printk("%s %s line %d\n", __FILE__, __FUNCTION__, __LINE__);
kthread_stop(sr501_kthread);
platform_driver_unregister(&sr501s_driver);
return;
}
module_init(sr501_init);
module_exit(sr501_exit);
MODULE_LICENSE("GPL");
与上一篇文章《HDF驱动框架探路(六):linux总线机制imx6ull驱动sr501红外传感器》中的第3节应用侧测试程序相同,点击进入
与上一篇文章《HDF驱动框架探路(六):linux总线机制imx6ull驱动sr501红外传感器》中的第2节修改设备树相同,点击进入
与上一篇文章《HDF驱动框架探路(六):linux总线机制imx6ull驱动sr501红外传感器》中的第4节编译,点击进入
代码存放路径为:codelab/sr501/bus_kthread/