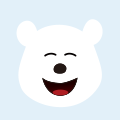
回复
4. 网格 Flutter Web 在线示例
grid.png
class GridExample extends StatelessWidget {
const GridExample({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
List<Color> colors = [
Colors.redAccent,
Colors.greenAccent,
Colors.blueAccent,
Colors.orangeAccent,
Colors.yellow,
Colors.pink,
Colors.lightBlueAccent
];
return Scaffold(
body: ConstraintLayout(
children: [
...constraintGrid(
id: ConstraintId('grid'),
left: parent.left,
top: parent.top,
itemCount: 50,
columnCount: 8,
itemWidth: 50,
itemHeight: 50,
itemBuilder: (index) {
return Container(
color: colors[index % colors.length],
);
},
itemMarginBuilder: (index) {
return const EdgeInsets.only(
left: 10,
top: 10,
);
})
],
),
);
}
}
5. 瀑布流 Flutter Web 在线示例
staggered_grid.gif
class StaggeredGridExample extends StatelessWidget {
const StaggeredGridExample({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
List<Color> colors = [
Colors.redAccent,
Colors.greenAccent,
Colors.blueAccent,
Colors.orangeAccent,
Colors.yellow,
Colors.pink,
Colors.lightBlueAccent
];
const double smallestSize = 40;
const int columnCount = 8;
Random random = Random();
return Scaffold(
body: ConstraintLayout(
children: [
TextButton(
onPressed: () {
(context as Element).markNeedsBuild();
},
child: const Text(
'Upset',
style: TextStyle(
fontSize: 32,
height: 1.5,
),
),
).applyConstraint(
left: ConstraintId('horizontalList').right,
top: ConstraintId('horizontalList').top,
),
...constraintGrid(
id: ConstraintId('horizontalList'),
left: parent.left,
top: parent.top,
margin: const EdgeInsets.only(
left: 100,
),
itemCount: 50,
columnCount: columnCount,
itemBuilder: (index) {
return Container(
color: colors[index % colors.length],
alignment: Alignment.center,
child: Text('$index'),
);
},
itemSizeBuilder: (index) {
if (index == 0) {
return const Size(
smallestSize * columnCount + 35, smallestSize);
}
if (index == 6) {
return const Size(smallestSize * 2 + 5, smallestSize);
}
if (index == 7) {
return const Size(smallestSize * 6 + 25, smallestSize);
}
if (index == 19) {
return const Size(smallestSize * 2 + 5, smallestSize);
}
if (index == 29) {
return const Size(smallestSize * 3 + 10, smallestSize);
}
return Size(
smallestSize, (2 + random.nextInt(4)) * smallestSize);
},
itemSpanBuilder: (index) {
if (index == 0) {
return columnCount;
}
if (index == 6) {
return 2;
}
if (index == 7) {
return 6;
}
if (index == 19) {
return 2;
}
if (index == 29) {
return 3;
}
return 1;
},
itemMarginBuilder: (index) {
return const EdgeInsets.only(
left: 5,
top: 5,
);
})
],
),
);
}
}
6. 圆形定位 Flutter Web 在线示例
circle_position.gif
class CirclePositionExampleState extends State<CirclePositionExample> {
late Timer timer;
late int hour;
late int minute;
late int second;
double centerTranslateX = 0;
double centerTranslateY = 0;
@override
void initState() {
super.initState();
calculateClockAngle();
timer = Timer.periodic(const Duration(seconds: 1), (_) {
calculateClockAngle();
});
}
void calculateClockAngle() {
setState(() {
DateTime now = DateTime.now();
hour = now.hour;
minute = now.minute;
second = now.second;
});
}
@override
void dispose() {
super.dispose();
timer.cancel();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: ConstraintLayout(
children: [
GestureDetector(
child: Container(
decoration: const BoxDecoration(
color: Colors.red,
borderRadius: BorderRadius.all(
Radius.circular(1000),
),
),
),
onPanUpdate: (details) {
setState(() {
centerTranslateX += details.delta.dx;
centerTranslateY += details.delta.dy;
});
},
).applyConstraint(
width: 20,
height: 20,
centerTo: parent,
zIndex: 100,
translate: Offset(centerTranslateX, centerTranslateY),
translateConstraint: true,
),
for (int i = 0; i < 12; i++)
Text(
'${i + 1}',
style: const TextStyle(
fontWeight: FontWeight.bold,
fontSize: 25,
),
).applyConstraint(
centerTo: rId(0),
translate: circleTranslate(
radius: 205,
angle: (i + 1) * 30,
),
),
for (int i = 0; i < 60; i++)
if (i % 5 != 0)
Transform.rotate(
angle: pi + pi * (i * 6 / 180),
child: Container(
color: Colors.grey,
margin: const EdgeInsets.only(
top: 405,
),
),
).applyConstraint(
width: 1,
height: 415,
centerTo: rId(0),
),
Transform.rotate(
angle: pi + pi * (hour * 30 / 180),
alignment: Alignment.topCenter,
child: Container(
color: Colors.green,
),
).applyConstraint(
width: 5,
height: 80,
centerTo: rId(0),
translate: const Offset(0, 0.5),
percentageTranslate: true,
),
Transform.rotate(
angle: pi + pi * (minute * 6 / 180),
alignment: Alignment.topCenter,
child: Container(
color: Colors.pink,
),
).applyConstraint(
width: 5,
height: 120,
centerTo: rId(0),
translate: const Offset(0, 0.5),
percentageTranslate: true,
),
Transform.rotate(
angle: pi + pi * (second * 6 / 180),
alignment: Alignment.topCenter,
child: Container(
color: Colors.blue,
),
).applyConstraint(
width: 5,
height: 180,
centerTo: rId(0),
translate: const Offset(0, 0.5),
percentageTranslate: true,
),
Text(
'$hour:$minute:$second',
style: const TextStyle(
fontSize: 40,
),
).applyConstraint(
outTopCenterTo: rId(0),
margin: const EdgeInsets.only(
bottom: 250,
),
)
],
),
);
}
}
文章转自公众号:FlutterFirst