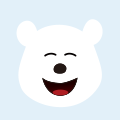
HarmonyOS API:通用属性
版本:v3.1 Beta
Popup控制
更新时间: 2023-02-17 09:19
给组件绑定popup弹窗,并设置弹窗内容,交互逻辑和显示状态。
说明
从API Version 7开始支持。后续版本如有新增内容,则采用上角标单独标记该内容的起始版本。
接口
名称 | 参数类型 | 描述 |
bindPopup | show: boolean, popup: PopupOptions | CustomPopupOptions8+ | 给组件绑定Popup弹窗,设置参数show为true弹出弹框。 show: 弹窗显示状态,默认值为false,隐藏弹窗。 popup: 配置当前弹窗提示的参数。 |
PopupOptions类型说明
名称 | 类型 | 必填 | 描述 |
message | string | 是 | 弹窗信息内容。 |
placementOnTop | boolean | 否 | 是否在组件上方显示,默认值为false。 |
primaryButton | { value: string, action: () => void } | 否 | 第一个按钮。 value: 弹窗里主按钮的文本。 action: 点击主按钮的回调函数。 |
secondaryButton | { value: string, action: () => void } | 否 | 第二个按钮。 value: 弹窗里辅助按钮的文本。 action: 点击辅助按钮的回调函数。 |
onStateChange | (event: { isVisible: boolean }) => void | 否 | 弹窗状态变化事件回调,参数isVisible为弹窗当前的显示状态。 |
arrowOffset9+ | Length | 否 | popup箭头在弹窗处的偏移。箭头在气泡上下方时,默认居左;箭头在气泡左右侧时,默认居上。 |
showInSubWindow9+ | boolean | 否 | 是否在子窗口显示气泡,默认值为false。 |
CustomPopupOptions8+类型说明
名称 | 类型 | 必填 | 描述 |
builder | 是 | 提示气泡内容的构造器。 | |
placement | 否 | 气泡组件优先显示的位置,当前位置显示不下时,会自动调整位置。 默认值:Placement.Bottom | |
maskColor | Color | string | Resource | number | 否 | 提示气泡遮障层的颜色。 |
popupColor | Color | string | Resource | number | 否 | 提示气泡的颜色。 |
enableArrow | boolean | 否 | 是否显示箭头。 从API Version 9开始,如果箭头所在方位侧的气泡长度不足以显示下箭头,则会默认不显示箭头。比如:placement设置为Left,但气泡高度小于箭头的宽度(32vp),则实际不会显示箭头。 默认值:true |
autoCancel | boolean | 否 | 页面有操作时,是否自动关闭气泡。 默认值:true |
onStateChange | (event: { isVisible: boolean }) => void | 否 | 弹窗状态变化事件回调,参数为弹窗当前的显示状态。 |
arrowOffset9+ | Length | 否 | popup箭头在弹窗处的偏移。箭头在气泡上下方时,默认居左;箭头在气泡左右侧时,默认居上。 |
showInSubWindow9+ | boolean | 否 | 是否在子窗口显示气泡,默认值为false。 |
示例
// xxx.ets
@Entry
@Component
struct PopupExample {
@State handlePopup: boolean = false
@State customPopup: boolean = false
// popup构造器定义弹框内容
@Builder popupBuilder() {
Row({ space: 2 }) {
Image($r("app.media.image")).width(24).height(24).margin({ left: -5 })
Text('Custom Popup').fontSize(10)
}.width(100).height(50).padding(5)
}
build() {
Flex({ direction: FlexDirection.Column }) {
// PopupOptions 类型设置弹框内容
Button('PopupOptions')
.onClick(() => {
this.handlePopup = !this.handlePopup
})
.bindPopup(this.handlePopup, {
message: 'This is a popup with PopupOptions',
placementOnTop: true,
showInSubWindow:false,
primaryButton: {
value: 'confirm',
action: () => {
this.handlePopup = !this.handlePopup
console.info('confirm Button click')
}
},
// 第二个按钮
secondaryButton: {
value: 'cancel',
action: () => {
this.handlePopup = !this.handlePopup
console.info('cancel Button click')
}
},
onStateChange: (e) => {
console.info(JSON.stringify(e.isVisible))
if (!e.isVisible) {
this.handlePopup = false
}
}
})
.position({ x: 100, y: 50 })
// CustomPopupOptions 类型设置弹框内容
Button('CustomPopupOptions')
.onClick(() => {
this.customPopup = !this.customPopup
})
.bindPopup(this.customPopup, {
builder: this.popupBuilder,
placement: Placement.Top,
maskColor: 0x33000000,
popupColor: Color.Yellow,
enableArrow: true,
showInSubWindow: false,
onStateChange: (e) => {
if (!e.isVisible) {
this.customPopup = false
}
}
})
.position({ x: 80, y: 200 })
}.width('100%').padding({ top: 5 })
}
}
Menu控制
更新时间: 2023-02-17 09:19
为组件绑定弹出式菜单,弹出式菜单以垂直列表形式显示菜单项,可通过长按、点击或鼠标右键触发。
说明
从API Version 7开始支持。后续版本如有新增内容,则采用上角标单独标记该内容的起始版本。
属性
名称 | 参数类型 | 描述 |
bindMenu | Array<MenuItem> | CustomBuilder | 给组件绑定菜单,点击后弹出菜单。弹出菜单项支持文本和自定义两种功能。 |
bindContextMenu8+ | content: CustomBuilder, responseType: ResponseType | 给组件绑定菜单,触发方式为长按或者右键点击,弹出菜单项需要自定义。 |
MenuItem
名称 | 类型 | 描述 |
value | string | 菜单项文本。 |
action | () => void | 点击菜单项的事件回调。 |
示例
示例1
普通菜单
// xxx.ets
@Entry
@Component
struct MenuExample {
build() {
Column() {
Text('click for Menu')
}
.width('100%')
.margin({ top: 5 })
.bindMenu([
{
value: 'Menu1',
action: () => {
console.info('handle Menu1 select')
}
},
{
value: 'Menu2',
action: () => {
console.info('handle Menu2 select')
}
},
])
}
}
示例2
自定义内容菜单。
该示例能力在HarmonyOS 3.1 Beta1暂不支持。
@Entry
@Component
struct MenuExample {
@State listData: number[] = [0, 0, 0]
@Builder MenuBuilder() {
Flex({ direction: FlexDirection.Column, justifyContent: FlexAlign.Center, alignItems: ItemAlign.Center }) {
ForEach(this.listData, (item, index) => {
Column() {
Row() {
Image($r("app.media.icon")).width(20).height(20).margin({ right: 5 })
Text(`Menu${index + 1}`).fontSize(20)
}
.width('100%')
.height(30)
.justifyContent(FlexAlign.Center)
.align(Alignment.Center)
.onClick(() => {
console.info(`Menu${index + 1} Clicked!`)
})
if (index != this.listData.length - 1) {
Divider().height(10).width('80%').color('#ccc')
}
}.padding(5).height(40)
})
}.width(100)
}
build() {
Column() {
Text('click for menu')
.fontSize(20)
.margin({ top: 20 })
.bindMenu(this.MenuBuilder)
}
.height('100%')
.width('100%')
.backgroundColor('#f0f0f0')
}
}
示例3
菜单(右键触发显示)
// xxx.ets
@Entry
@Component
struct ContextMenuExample {
@Builder MenuBuilder() {
Flex({ direction: FlexDirection.Column, justifyContent: FlexAlign.Center, alignItems: ItemAlign.Center }) {
Text('Test menu item 1')
.fontSize(20)
.width(100)
.height(50)
.textAlign(TextAlign.Center)
Divider().height(10)
Text('Test menu item 2')
.fontSize(20)
.width(100)
.height(50)
.textAlign(TextAlign.Center)
}.width(100)
}
build() {
Column() {
Text('rightclick for menu')
}
.width('100%')
.margin({ top: 5 })
.bindContextMenu(this.MenuBuilder, ResponseType.RightClick)
}
}
点击控制
更新时间: 2023-02-17 09:19
设置组件是否可以响应点击事件、触摸事件等手指交互事件。
说明
从API Version 7开始支持。后续版本如有新增内容,则采用上角标单独标记该内容的起始版本。
属性
名称 | 参数类型 | 描述 |
touchable | boolean | 设置当前组件是否可以响应点击事件、触摸事件等手指交互事件。 默认值:true |
示例
// xxx.ets
@Entry
@Component
struct TouchAbleExample {
@State text1: string = ''
@State text2: string = ''
build() {
Stack() {
Rect()
.fill(Color.Gray).width(150).height(150)
.onClick(() => {
console.info(this.text1 = 'Rect Clicked')
})
.overlay(this.text1, { align: Alignment.Bottom, offset: { x: 0, y: 20 } })
Ellipse()
.fill(Color.Pink).width(150).height(80)
.touchable(false) // 点击Ellipse区域,不会打印 “Ellipse Clicked”
.onClick(() => {
console.info(this.text2 = 'Ellipse Clicked')
})
.overlay(this.text2, { align: Alignment.Bottom, offset: { x: 0, y: 20 } })
}.margin(100)
}
}
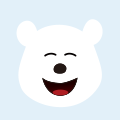