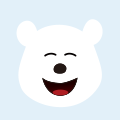
回复
本文包含两个文件的代码和一张测试效果图:
StackAndQueue.h文件: 用于存储信息:存放函数、结构体、栈的函数实现、变量名等
danceMatch.cpp文件: 用于测试
效果图:(位于最上方)
效果图:StackAndQueue.h文件:
#include<stdio.h>
#include <string.h>
#define OK 1
#define ERROR 0
#define TRUE 1
#define FALSE 0
#define MAXSIZE 20
typedef int Status;
//---------------栈
typedef char SElemType;
typedef struct{
SElemType data[MAXSIZE];
int top; //用于栈顶指针
}SqStack;
Status InitStack(SqStack *S){
for(int i = 0; i < MAXSIZE; i++){
S->data[i] = ' '; //用0初始化
}
S->top = -1;
}
Status StackSize(SqStack S){
return (S.top+1);
}
Status Push(SqStack *S, SElemType e){ //------进栈
if(S->top == MAXSIZE - 1){ //栈满
return ERROR;
}
S->top++; //栈顶指针增加一
S->data[S->top] = e; //将新插入元素赋值给栈顶空间
return OK;
}
Status Pop(SqStack *S, SElemType *e){
if(S->top == -1){ //栈空
return ERROR;
}
*e = S->data[S->top];
S->top--;
return OK;
}
//-------------队列
typedef char QElemType;
typedef struct{
QElemType data[MAXSIZE];
int front;
int rear;
}SqQueue;
Status InitQueue(SqQueue *Q){
Q->front = 0;
Q->rear = 0;
return OK;
}
int QueueLength(SqQueue Q){
return (Q.rear - Q.front +MAXSIZE) % MAXSIZE;
}
Status EnQueue(SqQueue *Q, QElemType e){ //入队列
if((Q->rear + 1) % MAXSIZE == Q->front){ //队列满
return ERROR;
}
Q->data[Q->rear] = e;
Q->rear = (Q->rear + 1) % MAXSIZE;
return OK;
}
Status DeQueue(SqQueue *Q, QElemType *e){ //出队列
if(Q->front == Q->rear){
return ERROR;
}
*e = Q->data[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return OK;
}
danceMatch.cpp文件:
#include "StackAndQueue.h"
void ManQueue(SqQueue* Q, int len){
InitQueue(Q);
char ch = 'A';
for(int i = 0; i < len; i++){
EnQueue(Q, ch++);
}
}
void WomanQueue(SqQueue* Q, int len){
InitQueue(Q);
char ch = 'a';
for(int i = 0; i < len; i++){
EnQueue(Q, ch++);
}
}
void PeiDui(SqQueue Q_man, SqQueue Q_woman, int m, int n, int num){
char ch_man, ch_woman;
printf("请输入舞会的轮数:");
scanf("%d", &num);
printf("分别输入男、女队人数(用空格隔开):");
scanf("%d%d", &m, &n);
ManQueue(&Q_man, m);
WomanQueue(&Q_woman, n);
int count = 1;
for(int i = 0; i < num; i++){
DeQueue(&Q_man, &ch_man);
DeQueue(&Q_woman, &ch_woman);
EnQueue(&Q_man, ch_man);
EnQueue(&Q_woman, ch_woman);
printf("第%d轮:有请男士%c与女士%c的带来的表演\n", count, ch_man, ch_woman);
count++;
if(count > num){
break;
}
}
}
int main(){
int m, n;
int num;
SqQueue Q_man, Q_woman;
PeiDui(Q_man, Q_woman, m, n, num);
}