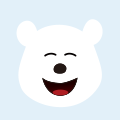
Uniapp中实现APP手写签名功能
手写签名功能在移动应用中有着广泛的应用场景,如电子合同签署、审批确认、单据验证等。本文将介绍如何在Uniapp中实现APP端的手写签名功能。
一、实现思路
在Uniapp中实现手写签名主要有两种方式:
使用Canvas绘图API实现自定义手写板
使用第三方插件或原生模块
本文将重点介绍第一种方式,即使用Canvas实现,这种方式跨平台兼容性好,且不需要依赖第三方库。
二、基础实现步骤
- 创建签名画布
- 样式设置
- JavaScript逻辑实现
三、功能优化
- 添加背景网格线
- 添加撤销功能
- 支持不同颜色和粗细
四、注意事项
Canvas尺寸问题:在移动设备上,Canvas的实际渲染尺寸和逻辑尺寸可能不同,需要根据设备像素比进行适配。
性能优化:对于复杂的签名,可以适当减少记录的点数或使用贝塞尔曲线优化。
跨平台兼容性:虽然Uniapp是跨平台的,但不同平台对Canvas的支持可能有细微差异,需要充分测试。
高清屏适配:在高DPI设备上,需要特别注意Canvas的清晰度问题。
保存格式:根据需求选择合适的图片格式(PNG/JPG),PNG支持透明背景,JPG文件更小。
五、完整组件封装
可以将签名功能封装为可复用的组件:
六、使用第三方插件
如果需要更强大的功能,可以考虑使用第三方插件:
uniapp-signature:专为Uniapp开发的签名插件
html2canvas:通过webview方式实现的截图方案
原生插件:对于性能要求高的场景,可以考虑开发原生插件
七、总结
本文介绍了在Uniapp中实现手写签名功能的完整方案,从基础的Canvas绘图到功能优化和组件封装。这种实现方式跨平台兼容性好,不需要依赖第三方库,适合大多数应用场景。对于更复杂的需求,可以考虑使用第三方插件或开发原生模块。
实际开发中,还需要根据具体业务需求进行调整,如添加时间戳、用户信息水印、多页签名等功能。
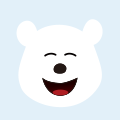