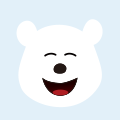
【牛角书】鸿蒙js实战,计算器功能开发
场景:
通过动态设置按钮组件button实现计算器的键盘,通过文本text显示计算的表达书,可以计算+,-,*,/,可以一个一个移除,可以重置 等。
一,实现思路
计算器的键盘,本来是想使用grid的 但是有一些默认属性不好控制,等后续组件完善了在做优化,目前grid适合一些均衡布局,通过监听计算符号添加判断逻辑,计算结果也是通过添加的计算类型进行计算,目前支持一级计算,后面做连续计算。
二,代码解析
子组件:
1,hml文件
实用了四个for循环实现了键盘效果,后面想了一下其实一个就能搞定,动态换行就行,时间有限后续优化(总感觉计算器挺简单,其实做起来还需要点时间)
<div class=“container”>
<text class=“input-content”>{{inputcontent}}</text>
<div class=“menu-content”>
<div class=“caluater” for=“{{ caluater }}” >
<button class=“content” onclick=“calculatorClick({{ $item.id }})”>{{ $item.id }}</button>
</div>
</div>
<div class=“menu-content”>
<div class=“caluater” for=“{{ caluater1 }}”>
<button class=“content2” on:click=“calculatorClick({{ $item.id }})”>{{ $item.id }}</button>
</div>
</div>
<div class=“menu-content”>
<div class=“caluater” for=“{{ caluater2 }}”>
<button class=“content2” on:click=“calculatorClick({{ $item.id }})”>{{ $item.id }}</button>
</div>
</div>
<div class="list2-content">
<div class="list3-content">
<div class="list2-content">
<div class="caluater" for="{{ caluater3 }}">
<button class="content2" on:click="calculatorClick({{ $item.id }})">{{ $item.id }}</button>
</div>
</div>
<div class="list2-content">
<div class="caluater" for="{{ caluater4 }}">
<button class="content2" on:click="calculatorClick({{ $item.id }})">{{ $item.id }}</button>
</div>
</div>
</div>
<button class="equals" onclick="calculatorResult">=</button>
</div>
</div
2,css文件
样式比较简单,主要控制键盘和表达式文本的颜色和大小
.container {
flex-direction: column;
justify-content: flex-end;
align-items: flex-end;
width: 100%;
}
.input-content{
background-color: #00ffffff;
text-align: right;
font-size: 25px;
padding: 10px;
font-weight: bold;
}
.menu-content{
width: 100%;
background-color: brown;
allow-scale: true;
}
.caluater {
flex-direction: row;
width: 100%;
height: 70px;
background-color: #00ffffff;
margin-left: 5px;
margin-right: 5px;
margin-top: 10px;
}
.content{
font-size: 30px;
font-weight: bold;
radius: 10px;
width: 100%;
height: 100%;
text-color: #007EFF;
background-color: #A8CCFB;
}
.content2{
font-size: 30px;
font-weight: bold;
radius: 10px;
width: 100%;
height: 100%;
text-color: #000000;
background-color: #dddcdc;
}
.list2-content{
flex-direction: row;
justify-content: center;
align-items: center;
background-color: brown;
}
.list3-content{
flex-direction: column;
margin-bottom: 10px;
}
.equals{
width: 30%;
height: 150px;
font-size: 30px;
font-weight: bold;
radius: 10px;
margin-left: 5px;
margin-right: 5px;
}
3,js文件
js中主要实现逻辑,请看具体计算的判断。
import prompt from ‘@system.prompt’;
export default {
data: {
title: “”,
inputcontent: “”,
number1: “”,
number2: “”,
type: “”,
caluater: [
{
‘id’: “C”,
},
{
‘id’: “÷”,
},
{
‘id’: “×”,
},
{
‘id’: “←”,
}
],
caluater1:[
{
‘id’: “7”,
},
{
‘id’: “8”,
},
{
‘id’: “9”,
},
{
‘id’: “+”,
}
],
caluater2:[
{
‘id’: “4”,
},
{
‘id’: “5”,
},
{
‘id’: “6”,
},
{
‘id’: “-”,
}
],
caluater3:[
{
‘id’: “1”,
},
{
‘id’: “2”,
},
{
‘id’: “3”,
}
],
caluater4:[
{
‘id’: “%”,
},
{
‘id’: “0”,
},
{
‘id’: “.”,
}
]
},
onInit() {
},
calculatorClick(result){
this.inputcontent = this.inputcontent+“”;
let str = this.inputcontent
.substring(this.inputcontent.length-1, this.inputcontent.length);
switch(result) {
case “←”:
if(this.inputcontent.length > 0) {
let str = this.inputcontent
.substring(0, this.inputcontent.length - 1);
this.inputcontent = str;
}
break;
case “C”:
this.inputcontent = “”;
break;
case “+”:
this.calcula(str,result,“+”);
break;
case “-”:
this.calcula(str,result,“-”);
break;
case “×”:
this.calcula(str,result,“×”);
break;
case “÷”:
this.calcula(str,result,“÷”);
break;
case “.”:
if(this.inputcontent.length > 0 && str != “.”) {
this.inputcontent += result;
}
break;
default:
this.inputcontent += result;
break;
}
},
calcula(str,result,cla){
if(this.inputcontent.length > 0 && str != “+” && str != “-” && str != “×” && str != “÷”) {
this.type = cla;
this.inputcontent += result;
} else {
this.calculatorResult();
}
},
calculatorResult(){// 计算结果
var temp = this.inputcontent.split(this.type);
console.log("this.inputcontent = "+this.inputcontent)
let str = this.inputcontent
.substring(this.inputcontent.length-1, this.inputcontent.length);
console.log("this.type = "+this.type)
if (this.type == “+”) { // 加法计算
if(temp.length > 1) {
console.log("temp = "+temp)
var num1 = parseFloat(temp[0]);
var num2 = parseFloat(temp[1]);
console.log("num1 = "+num1)
console.log("num2 = "+num2)
console.log("str = "+str)
if(str != “+”) {
this.inputcontent = num1 + num2;
this.type = “”;
}
}
} else if(this.type == “-”) { // 减法计算
if(temp.length > 1) {
var num1 = parseFloat(temp[0]);
var num2 = parseFloat(temp[1]);
if(str != “-”) {
this.inputcontent = num1 - num2;
this.type = “”;
}
}
} else if(this.type == “×”) { // 乘法计算
if(temp.length > 1) {
var num1 = parseFloat(temp[0]);
var num2 = parseFloat(temp[1]);
if(str != “×”) {
this.inputcontent = num1 * num2;
this.type = “”;
}
}
} else if(this.type == “÷”) { // 除法计算
if(temp.length > 1) {
var num1 = parseFloat(temp[0]);
var num2 = parseFloat(temp[1]);
if(str != “÷”) {
this.inputcontent = num1 / num2;
this.type = “”;
}
}
}
}
}
三,实现效果
四,总结
开发计算器最主要的是连续计算,连续计算需要添加计算优先级逻辑,后续考虑通过遍历来判断里面的计算。
计算器界面开发通过常用组件就能实现,实现方式可以自己定。
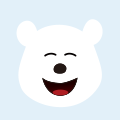